在 Python 类中使用另一个方法的方法有:通过实例方法调用、通过类方法调用、通过静态方法调用。其中,最常见的方式是通过实例方法调用。下面将详细描述如何通过实例方法调用来实现这一点,并介绍其他两种方法的使用场景。
通过实例方法调用
实例方法调用是 Python 类中最常见的调用方法之一。它使得一个方法可以调用同一个类中的另一个方法。例如:
class MyClass:
def method1(self):
print("Method 1 called")
self.method2() # 调用 method2
def method2(self):
print("Method 2 called")
创建类的实例
my_instance = MyClass()
my_instance.method1()
在这个例子中,method1
调用了 method2
。使用 self
关键字可以访问同一个类中的其他方法。这种方式广泛应用于类的方法之间的互相调用。接下来我们将详细探讨其他方法的使用场景和实现方式。
一、实例方法的调用
实例方法是类的实例对象所调用的方法。通过实例方法调用其他实例方法是最常见的方式。实例方法通常需要通过 self
参数来访问同一个类中的其他方法和属性。
1.1 基础实例方法调用
实例方法的调用通常用于在类的不同方法之间进行逻辑衔接。例如,假设我们有一个代表银行账户的类:
class BankAccount:
def __init__(self, owner, balance=0):
self.owner = owner
self.balance = balance
def deposit(self, amount):
if amount > 0:
self.balance += amount
self.show_balance() # 调用 show_balance 方法
else:
print("Deposit amount must be positive")
def withdraw(self, amount):
if 0 < amount <= self.balance:
self.balance -= amount
self.show_balance() # 调用 show_balance 方法
else:
print("Insufficient funds or invalid amount")
def show_balance(self):
print(f"Owner: {self.owner}, Balance: {self.balance}")
创建类的实例
account = BankAccount("Alice", 100)
account.deposit(50)
account.withdraw(30)
在这个例子中,deposit
和 withdraw
方法都调用了 show_balance
方法来显示当前的账户余额。
1.2 复杂的实例方法调用
在更复杂的场景中,实例方法之间的调用可能涉及更多的逻辑。例如,一个代表订单处理的类:
class OrderProcessor:
def __init__(self, order_id):
self.order_id = order_id
self.items = []
self.status = "New"
def add_item(self, item):
self.items.append(item)
self.update_status("Items Added") # 调用 update_status 方法
def process_payment(self, payment_info):
if self.verify_payment(payment_info):
self.update_status("Payment Processed") # 调用 update_status 方法
else:
self.update_status("Payment Failed") # 调用 update_status 方法
def update_status(self, status):
self.status = status
self.log_status() # 调用 log_status 方法
def log_status(self):
print(f"Order {self.order_id} is now {self.status}")
def verify_payment(self, payment_info):
# 这里省略支付验证的具体逻辑
return True
创建类的实例
order = OrderProcessor("A123")
order.add_item("Item1")
order.process_payment("PaymentInfo")
在这个例子中,add_item
和 process_payment
方法都调用了 update_status
方法,而 update_status
方法又调用了 log_status
方法。
二、类方法的调用
类方法使用 @classmethod
装饰器来定义,并且第一个参数是 cls
,表示类本身。类方法可以通过类名或者类的实例来调用。
2.1 基础类方法调用
类方法通常用于那些需要访问或修改类级别状态的方法。例如:
class MyClass:
class_variable = 0
@classmethod
def increment_class_variable(cls):
cls.class_variable += 1
cls.show_class_variable() # 调用类方法
@classmethod
def show_class_variable(cls):
print(f"Class variable is now {cls.class_variable}")
调用类方法
MyClass.increment_class_variable()
在这个例子中,increment_class_variable
方法调用了 show_class_variable
方法来显示类变量的当前值。
2.2 复杂的类方法调用
在更复杂的场景中,类方法之间的调用可能涉及类级别的状态管理。例如,一个用于管理员工的类:
class EmployeeManager:
employees = []
@classmethod
def add_employee(cls, name):
cls.employees.append(name)
cls.show_employees() # 调用类方法
@classmethod
def remove_employee(cls, name):
cls.employees.remove(name)
cls.show_employees() # 调用类方法
@classmethod
def show_employees(cls):
print(f"Employees: {', '.join(cls.employees)}")
调用类方法
EmployeeManager.add_employee("Alice")
EmployeeManager.add_employee("Bob")
EmployeeManager.remove_employee("Alice")
在这个例子中,add_employee
和 remove_employee
方法都调用了 show_employees
方法来显示当前的员工列表。
三、静态方法的调用
静态方法使用 @staticmethod
装饰器来定义,它不需要 self
或 cls
参数。静态方法通常用于那些不需要访问实例或类级别状态的方法。
3.1 基础静态方法调用
静态方法可以通过类名或者类的实例来调用。例如:
class MyClass:
@staticmethod
def static_method():
print("Static method called")
MyClass.another_static_method() # 调用静态方法
@staticmethod
def another_static_method():
print("Another static method called")
调用静态方法
MyClass.static_method()
在这个例子中,static_method
方法调用了 another_static_method
方法。
3.2 复杂的静态方法调用
在更复杂的场景中,静态方法之间的调用可能涉及独立于实例和类状态的逻辑。例如,一个用于计算数学公式的类:
class MathOperations:
@staticmethod
def add(a, b):
result = a + b
MathOperations.log_operation("add", a, b, result) # 调用静态方法
return result
@staticmethod
def multiply(a, b):
result = a * b
MathOperations.log_operation("multiply", a, b, result) # 调用静态方法
return result
@staticmethod
def log_operation(operation, a, b, result):
print(f"Operation {operation}: {a} and {b} resulted in {result}")
调用静态方法
MathOperations.add(5, 3)
MathOperations.multiply(4, 2)
在这个例子中,add
和 multiply
方法都调用了 log_operation
方法来记录操作结果。
四、实例方法、类方法和静态方法的混合调用
在实际应用中,类中可能会同时包含实例方法、类方法和静态方法,并且它们之间可能相互调用。了解这三种方法的不同特性和使用场景是非常重要的。
4.1 实例方法与类方法的混合调用
实例方法和类方法可以相互调用。例如,一个用于管理用户的类:
class UserManager:
users = []
def __init__(self, name):
self.name = name
self.add_user(self.name) # 调用类方法
@classmethod
def add_user(cls, name):
cls.users.append(name)
cls.show_users() # 调用类方法
@classmethod
def show_users(cls):
print(f"Users: {', '.join(cls.users)}")
创建类的实例
user1 = UserManager("Alice")
user2 = UserManager("Bob")
在这个例子中,实例方法 __init__
调用了类方法 add_user
,而 add_user
方法又调用了 show_users
方法。
4.2 类方法与静态方法的混合调用
类方法和静态方法也可以相互调用。例如,一个用于管理产品库存的类:
class InventoryManager:
inventory = {}
@classmethod
def add_product(cls, product, quantity):
if product in cls.inventory:
cls.inventory[product] += quantity
else:
cls.inventory[product] = quantity
cls.show_inventory() # 调用类方法
@classmethod
def show_inventory(cls):
for product, quantity in cls.inventory.items():
print(f"Product: {product}, Quantity: {quantity}")
@staticmethod
def calculate_total_value(prices):
total_value = sum(prices.values())
InventoryManager.log_total_value(total_value) # 调用静态方法
return total_value
@staticmethod
def log_total_value(value):
print(f"Total inventory value: {value}")
调用类方法
InventoryManager.add_product("Widget", 10)
InventoryManager.add_product("Gadget", 5)
调用静态方法
prices = {"Widget": 100, "Gadget": 200}
InventoryManager.calculate_total_value(prices)
在这个例子中,类方法 add_product
调用了 show_inventory
方法,而静态方法 calculate_total_value
调用了 log_total_value
方法。
五、总结
在 Python 中,类方法、实例方法和静态方法之间的调用非常灵活,但了解它们各自的特性和最佳使用场景是非常重要的。通过实例方法调用通常是最常见的方式,因为它们可以访问实例级别的状态和行为。类方法适用于需要访问或修改类级别状态的场景,而静态方法则适用于那些不依赖实例或类状态的独立逻辑。理解这些方法之间的区别和联系,可以帮助你编写更清晰、更有效的代码。
相关问答FAQs:
如何在Python类中调用其他方法?
在Python类中,可以通过使用self
关键字来调用其他方法。self
代表类的实例,通过self.method_name()
的方式即可调用同一类中的其他方法。这种方式确保了能够访问类的属性和其他方法。
在类中定义方法时,有哪些注意事项?
在定义类的方法时,确保第一个参数始终是self
,这使得方法能够访问实例的属性和其他方法。此外,方法名应具有描述性,以便清楚地表达其功能。避免使用与Python内置函数重名的方法名,以防止潜在的冲突。
如何处理类中的方法参数?
在定义类的方法时,可以传递参数以实现更灵活的功能。除了self
,可以定义其他参数并在调用时传入。例如,定义一个方法def example_method(self, param)
,在调用时通过self.example_method(value)
传递参数。这样能够增强方法的通用性和适应性。
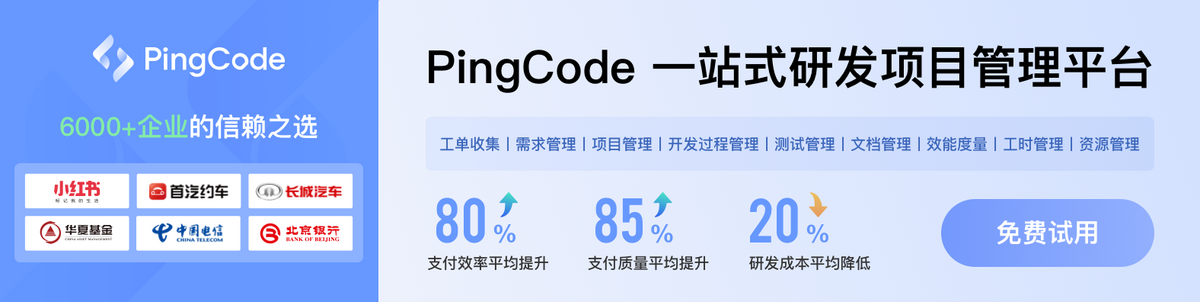