如何五天内速学Python:掌握基础语法、练习核心数据结构、理解控制流、熟悉常用库、进行实战项目
在五天内速学Python,关键在于掌握基础语法、练习核心数据结构、理解控制流、熟悉常用库、进行实战项目。其中,掌握基础语法是最重要的一步,因为了解Python的基本语法和结构是学习其他部分的前提。以下将详细描述如何在五天内速学Python:
一、掌握基础语法
掌握基础语法是学习Python的第一步。Python作为一种高级编程语言,以其简洁和易读的语法著称。学习基础语法包括变量和数据类型、运算符、输入输出、注释和基本的错误处理等。
1.1 变量和数据类型
变量是编程中的基本元素,用来存储数据。在Python中,变量的声明和赋值非常简单,不需要明确指定数据类型。
# 声明变量并赋值
a = 10
b = 3.14
c = "Hello, World!"
Python常见的数据类型包括整数(int)、浮点数(float)、字符串(str)、布尔值(bool)等。
1.2 运算符
Python支持多种运算符,包括算术运算符、比较运算符、逻辑运算符和赋值运算符。
# 算术运算符
x = 10
y = 3
print(x + y) # 加法
print(x - y) # 减法
print(x * y) # 乘法
print(x / y) # 除法
print(x // y) # 取整除
print(x % y) # 取余
print(x y) # 幂运算
比较运算符
print(x > y) # 大于
print(x < y) # 小于
print(x == y) # 等于
逻辑运算符
print(x > 5 and y < 5) # 与
print(x > 5 or y > 5) # 或
print(not(x > 5)) # 非
1.3 输入输出
输入和输出是程序与用户交互的基本方式。Python使用input()
函数获取用户输入,使用print()
函数输出结果。
# 获取用户输入
name = input("Enter your name: ")
print("Hello, " + name)
格式化输出
age = 25
print("My name is {} and I am {} years old.".format(name, age))
1.4 注释和基本错误处理
注释用于解释代码,便于理解和维护。Python使用井号(#)表示单行注释,使用三引号('''或""")表示多行注释。基本错误处理使用try
和except
语句。
# 单行注释
print("This is a single line comment.") # 这是单行注释
'''
多行注释
可以跨越多行
'''
print("This is a multi-line comment.")
错误处理
try:
result = 10 / 0
except ZeroDivisionError:
print("You cannot divide by zero!")
二、练习核心数据结构
Python提供了丰富的数据结构,如列表、元组、集合和字典。这些数据结构是编写高效代码的基础。
2.1 列表
列表是有序的、可变的集合,使用方括号表示。
# 创建列表
fruits = ["apple", "banana", "cherry"]
print(fruits)
访问列表元素
print(fruits[0]) # 输出第一个元素
print(fruits[-1]) # 输出最后一个元素
修改列表
fruits[1] = "blueberry"
print(fruits)
添加元素
fruits.append("orange")
print(fruits)
删除元素
del fruits[2]
print(fruits)
2.2 元组
元组是有序的、不可变的集合,使用圆括号表示。
# 创建元组
colors = ("red", "green", "blue")
print(colors)
访问元组元素
print(colors[1])
元组不可变,因此不能修改或删除元素
2.3 集合
集合是无序的、不重复的元素集合,使用大括号表示。
# 创建集合
animals = {"cat", "dog", "bird"}
print(animals)
添加元素
animals.add("fish")
print(animals)
删除元素
animals.remove("dog")
print(animals)
2.4 字典
字典是键值对的集合,使用大括号表示。
# 创建字典
person = {
"name": "Alice",
"age": 25,
"city": "New York"
}
print(person)
访问字典元素
print(person["name"])
修改字典
person["age"] = 26
print(person)
添加键值对
person["job"] = "Engineer"
print(person)
删除键值对
del person["city"]
print(person)
三、理解控制流
控制流语句用于控制程序的执行顺序,包括条件语句、循环语句和函数。
3.1 条件语句
条件语句根据条件的真假执行不同的代码块。Python使用if
、elif
和else
来实现条件判断。
age = 18
if age < 18:
print("You are a minor.")
elif age == 18:
print("You just turned 18!")
else:
print("You are an adult.")
3.2 循环语句
循环语句用于重复执行代码块。Python提供了for
和while
两种循环语句。
# for循环
fruits = ["apple", "banana", "cherry"]
for fruit in fruits:
print(fruit)
while循环
count = 0
while count < 5:
print(count)
count += 1
3.3 函数
函数是组织代码的基本单元,用于实现特定功能。Python使用def
关键字定义函数。
# 定义函数
def greet(name):
return "Hello, " + name
调用函数
print(greet("Alice"))
函数可以有默认参数
def greet(name, greeting="Hello"):
return greeting + ", " + name
print(greet("Bob"))
print(greet("Charlie", "Hi"))
四、熟悉常用库
Python拥有丰富的标准库和第三方库,涵盖了各种应用场景。熟悉这些库可以大大提高编程效率。
4.1 标准库
Python的标准库包含了许多常用的模块,如math
、datetime
、os
等。
import math
数学运算
print(math.sqrt(16)) # 平方根
print(math.pi) # 圆周率
import datetime
日期和时间
now = datetime.datetime.now()
print(now)
import os
操作系统接口
print(os.getcwd()) # 获取当前工作目录
4.2 第三方库
第三方库可以通过pip
安装,如numpy
、pandas
、matplotlib
等。
# 安装numpy
pip install numpy
import numpy as np
数组操作
array = np.array([1, 2, 3, 4])
print(array)
print(np.mean(array)) # 平均值
安装pandas
pip install pandas
import pandas as pd
数据处理
data = {'name': ['Alice', 'Bob', 'Charlie'], 'age': [25, 30, 35]}
df = pd.DataFrame(data)
print(df)
安装matplotlib
pip install matplotlib
import matplotlib.pyplot as plt
数据可视化
plt.plot([1, 2, 3], [4, 5, 6])
plt.show()
五、进行实战项目
通过实战项目巩固所学知识,是学习编程的关键。选择一个小型项目,如简单的计算器、数据分析、网页爬虫等,有助于综合运用各种知识点。
5.1 简单的计算器
编写一个简单的计算器,支持加法、减法、乘法和除法。
def add(x, y):
return x + y
def subtract(x, y):
return x - y
def multiply(x, y):
return x * y
def divide(x, y):
if y == 0:
return "Error! Division by zero."
return x / y
print("Select operation:")
print("1. Add")
print("2. Subtract")
print("3. Multiply")
print("4. Divide")
choice = input("Enter choice(1/2/3/4): ")
num1 = float(input("Enter first number: "))
num2 = float(input("Enter second number: "))
if choice == '1':
print("Result:", add(num1, num2))
elif choice == '2':
print("Result:", subtract(num1, num2))
elif choice == '3':
print("Result:", multiply(num1, num2))
elif choice == '4':
print("Result:", divide(num1, num2))
else:
print("Invalid input")
5.2 数据分析
使用pandas
进行简单的数据分析。
import pandas as pd
创建数据
data = {
'name': ['Alice', 'Bob', 'Charlie', 'David', 'Edward'],
'age': [24, 27, 22, 32, 29],
'city': ['New York', 'Chicago', 'San Francisco', 'Los Angeles', 'Boston']
}
转换为DataFrame
df = pd.DataFrame(data)
显示数据
print(df)
计算平均年龄
average_age = df['age'].mean()
print("Average age:", average_age)
按年龄排序
sorted_df = df.sort_values(by='age')
print("Sorted by age:")
print(sorted_df)
5.3 网页爬虫
使用requests
和BeautifulSoup
库编写一个简单的网页爬虫。
import requests
from bs4 import BeautifulSoup
发送HTTP请求
url = 'https://example.com'
response = requests.get(url)
解析HTML
soup = BeautifulSoup(response.text, 'html.parser')
提取数据
titles = soup.find_all('h2')
for title in titles:
print(title.text)
通过上述五个步骤,你可以在五天内速学Python。每天分配一定的时间,专注于一个或两个主题,逐步掌握Python的核心知识和技能。实践是学习编程的关键,动手编写代码,解决实际问题,可以帮助你更快地掌握Python。
相关问答FAQs:
如何在五天内有效学习Python?
在五天内学习Python的关键在于制定一个合理的学习计划。可以每天集中在特定的主题上,比如第一天学习基本语法,第二天掌握数据结构,第三天了解控制流,第四天进行函数和模块的学习,第五天进行项目实践和复习。通过这种方式,你可以在短时间内建立起Python的基础知识。
有哪些资源可以帮助我快速学习Python?
为了在短时间内掌握Python,可以利用在线课程、视频教程和编程书籍等多种资源。平台如Coursera、Udemy和YouTube上有许多针对初学者的优质课程。此外,Python的官方网站和相关社区(如Stack Overflow)也提供了丰富的学习材料和解答。
学习Python时,应该注意哪些常见的错误?
在学习Python的过程中,初学者常常会犯一些错误,比如忽视代码的缩进、对数据类型的理解不够深刻,以及未能有效使用Python的内置函数。建议在编写代码时多加注释,并时常回顾和练习已学过的知识,以避免这些常见的陷阱。通过参与编程社区的讨论,也能获得更多实用的建议。
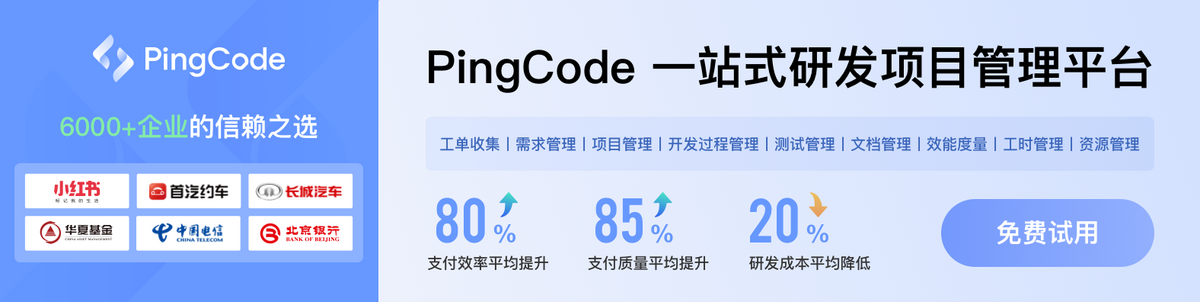