快速合并两个文件可以通过以下方法:使用文件读取与写入、借助操作系统命令、使用Pandas库。本文将详细介绍每种方法,并解释如何应用它们。
一、文件读取与写入
1.1 逐行读取与写入
这是最基本的方法之一,通过逐行读取两个文件的内容,然后将其写入到一个新文件中。
def merge_files(file1, file2, output_file):
with open(file1, 'r') as f1, open(file2, 'r') as f2, open(output_file, 'w') as out:
for line in f1:
out.write(line)
for line in f2:
out.write(line)
merge_files('file1.txt', 'file2.txt', 'merged.txt')
在这个示例中,首先打开两个要合并的文件和一个输出文件,然后逐行读取每个文件的内容并写入到输出文件中。这种方法简单直观,但在处理大文件时可能效率较低。
1.2 使用shutil
库
shutil
是Python标准库中的一个实用程序模块,提供了许多高级的文件操作功能。
import shutil
def merge_files(file1, file2, output_file):
with open(output_file, 'wb') as out:
with open(file1, 'rb') as f1:
shutil.copyfileobj(f1, out)
with open(file2, 'rb') as f2:
shutil.copyfileobj(f2, out)
merge_files('file1.txt', 'file2.txt', 'merged.txt')
使用shutil.copyfileobj
方法可以在不需要将文件内容全部读入内存的情况下实现文件的合并,从而提高效率。这个方法非常适合处理大文件。
二、借助操作系统命令
在某些情况下,直接调用操作系统命令可能会更快。Python的os
模块可以帮助我们执行这些命令。
2.1 使用os.system
方法
在Linux和macOS系统中,可以使用cat
命令合并文件;在Windows系统中,可以使用type
命令。
import os
def merge_files(file1, file2, output_file):
os.system(f"cat {file1} {file2} > {output_file}")
merge_files('file1.txt', 'file2.txt', 'merged.txt')
2.2 使用subprocess
模块
subprocess
模块提供了更强大的功能,可以捕获输出和错误信息。
import subprocess
def merge_files(file1, file2, output_file):
command = f"cat {file1} {file2} > {output_file}"
subprocess.run(command, shell=True, check=True)
merge_files('file1.txt', 'file2.txt', 'merged.txt')
使用操作系统命令可以充分利用系统的底层功能,通常比纯Python实现更高效,但这种方法的跨平台兼容性可能较差。
三、使用Pandas库
Pandas是一个强大的数据处理和分析库,适合处理结构化数据文件如CSV、Excel等。
3.1 合并CSV文件
如果要合并两个CSV文件,可以使用Pandas的concat
函数。
import pandas as pd
def merge_csv_files(file1, file2, output_file):
df1 = pd.read_csv(file1)
df2 = pd.read_csv(file2)
merged_df = pd.concat([df1, df2])
merged_df.to_csv(output_file, index=False)
merge_csv_files('file1.csv', 'file2.csv', 'merged.csv')
3.2 合并Excel文件
同样的,Pandas也可以用来合并Excel文件。
def merge_excel_files(file1, file2, output_file):
df1 = pd.read_excel(file1)
df2 = pd.read_excel(file2)
merged_df = pd.concat([df1, df2])
merged_df.to_excel(output_file, index=False)
merge_excel_files('file1.xlsx', 'file2.xlsx', 'merged.xlsx')
Pandas库提供了强大的数据处理功能,适合处理结构化数据文件,但对于纯文本文件可能稍显复杂。
四、优化与考虑
4.1 处理大文件
在处理大文件时,逐行读取与写入的方法可能会导致内存不足的问题。因此,使用shutil
库或操作系统命令是更优的选择。
4.2 异常处理
在实际应用中,必须考虑文件是否存在、是否有读写权限等问题。因此,异常处理是必不可少的。
def merge_files(file1, file2, output_file):
try:
with open(file1, 'r') as f1, open(file2, 'r') as f2, open(output_file, 'w') as out:
for line in f1:
out.write(line)
for line in f2:
out.write(line)
except FileNotFoundError as e:
print(f"Error: {e}")
except IOError as e:
print(f"IO Error: {e}")
merge_files('file1.txt', 'file2.txt', 'merged.txt')
4.3 性能测试
在选择合并文件的方法时,性能测试是非常重要的。可以通过实际测试不同方法的执行时间来选择最佳方案。
import time
start_time = time.time()
merge_files('file1.txt', 'file2.txt', 'merged.txt')
end_time = time.time()
print(f"Time taken: {end_time - start_time} seconds")
性能测试可以帮助我们找到最适合具体场景的方法。
4.4 并发处理
对于非常大的文件,可以考虑使用并发处理来提高效率。Python的concurrent.futures
模块提供了简单易用的并发工具。
import concurrent.futures
def read_file(file):
with open(file, 'r') as f:
return f.readlines()
def merge_files(file1, file2, output_file):
with concurrent.futures.ThreadPoolExecutor() as executor:
future1 = executor.submit(read_file, file1)
future2 = executor.submit(read_file, file2)
lines1 = future1.result()
lines2 = future2.result()
with open(output_file, 'w') as out:
out.writelines(lines1 + lines2)
merge_files('file1.txt', 'file2.txt', 'merged.txt')
并发处理可以显著提高文件合并的效率,但需要注意线程安全和潜在的竞争条件。
总的来说,Python提供了多种方法来快速合并两个文件,选择具体方法时应根据文件类型、大小以及具体应用场景进行优化。无论是文件读取与写入、借助操作系统命令,还是使用Pandas库,每种方法都有其独特的优缺点。通过合理的选择和优化,可以实现高效、可靠的文件合并。
相关问答FAQs:
如何在Python中合并两个文本文件?
在Python中,合并两个文本文件可以通过简单的文件读写操作实现。可以使用open()
函数打开两个文件,读取它们的内容,然后将内容写入到一个新的文件中。示例代码如下:
with open('file1.txt', 'r') as file1, open('file2.txt', 'r') as file2, open('merged_file.txt', 'w') as merged_file:
merged_file.write(file1.read())
merged_file.write(file2.read())
这段代码可以高效地将file1.txt
和file2.txt
合并到merged_file.txt
中。
在合并文件时如何处理文件编码问题?
处理文件编码时,可以在打开文件时指定编码格式。例如,使用encoding='utf-8'
可以确保文件以UTF-8编码格式读取和写入。示例代码如下:
with open('file1.txt', 'r', encoding='utf-8') as file1, open('file2.txt', 'r', encoding='utf-8') as file2, open('merged_file.txt', 'w', encoding='utf-8') as merged_file:
merged_file.write(file1.read())
merged_file.write(file2.read())
这样可以避免因编码不一致而导致的错误。
合并文件时如何确保不丢失数据?
为了确保在合并文件时不会丢失数据,可以在写入新文件前检查每个源文件的内容是否为空。可以使用条件语句来判断文件是否有内容,示例代码如下:
def merge_files(file1_path, file2_path, merged_file_path):
with open(file1_path, 'r', encoding='utf-8') as file1, open(file2_path, 'r', encoding='utf-8') as file2, open(merged_file_path, 'w', encoding='utf-8') as merged_file:
if file1.readable():
merged_file.write(file1.read())
if file2.readable():
merged_file.write(file2.read())
merge_files('file1.txt', 'file2.txt', 'merged_file.txt')
这种方法确保了即使一个文件为空,合并过程也不会中断。
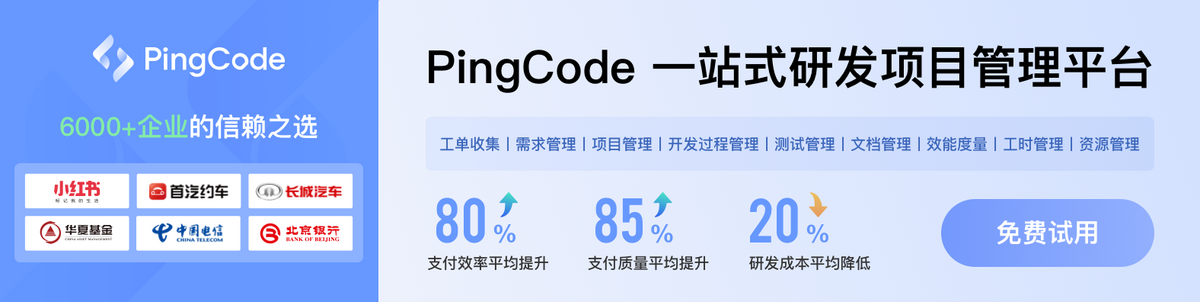