如何使用Python字符串打印出来
使用Python字符串打印出来的常用方法包括:print()函数、格式化字符串、字符串拼接、f-strings。在这篇文章中,我们将详细介绍这些方法,并提供实际的代码示例来帮助你更好地理解和应用它们。特别地,我们将深入探讨Python中的f-strings,这是一个非常强大和灵活的工具。
一、print()函数
Python中的print()
函数是最基本的输出方式。它可以将字符串、数字或其他数据类型打印到控制台。print()
函数的基本语法如下:
print(value, ..., sep=' ', end='\n', file=sys.stdout, flush=False)
1.1 打印单个字符串
最简单的用法是直接打印单个字符串:
print("Hello, World!")
这将输出:
Hello, World!
1.2 打印多个字符串
你可以同时打印多个字符串,默认情况下,它们之间会用空格分隔:
print("Hello", "World!")
这将输出:
Hello World!
1.3 使用sep参数
sep
参数可以用来指定分隔符:
print("Hello", "World!", sep=", ")
这将输出:
Hello, World!
1.4 使用end参数
end
参数可以用来指定结束符,默认是换行符\n
:
print("Hello", end=" ")
print("World!")
这将输出:
Hello World!
二、格式化字符串
Python提供了多种方式来格式化字符串,包括百分号(%
)、str.format()
方法和f-strings。在现代Python代码中,f-strings通常是首选,因为它们更简洁且性能更好。
2.1 百分号(%)格式化
这是较旧的格式化方式,但仍然广泛使用。基本语法如下:
name = "Alice"
age = 25
print("My name is %s and I am %d years old." % (name, age))
这将输出:
My name is Alice and I am 25 years old.
2.2 str.format()方法
这是Python 3引入的一种更强大和灵活的字符串格式化方式。基本语法如下:
name = "Alice"
age = 25
print("My name is {} and I am {} years old.".format(name, age))
这将输出:
My name is Alice and I am 25 years old.
2.3 f-strings
这是Python 3.6引入的一种更简洁的格式化方式。基本语法如下:
name = "Alice"
age = 25
print(f"My name is {name} and I am {age} years old.")
这将输出:
My name is Alice and I am 25 years old.
三、字符串拼接
字符串拼接是将多个字符串合并为一个字符串的操作。Python提供了多种拼接字符串的方法,包括使用加号(+
)、join()
方法和格式化字符串。
3.1 使用加号(+)
最简单的拼接方法是使用加号:
str1 = "Hello"
str2 = "World"
result = str1 + " " + str2
print(result)
这将输出:
Hello World
3.2 使用join()方法
join()
方法提供了一种更高效的方式来拼接多个字符串,特别是当你需要拼接大量字符串时:
words = ["Hello", "World"]
result = " ".join(words)
print(result)
这将输出:
Hello World
3.3 使用格式化字符串
你还可以使用格式化字符串来拼接:
str1 = "Hello"
str2 = "World"
result = "{} {}".format(str1, str2)
print(result)
这将输出:
Hello World
四、f-strings
f-strings是一种非常强大且灵活的字符串格式化方式。它们不仅支持简单的变量插值,还支持表达式和函数调用。
4.1 基本用法
基本用法非常简单,只需在字符串前加上字母f
,然后在花括号{}
中插入变量或表达式:
name = "Alice"
age = 25
print(f"My name is {name} and I am {age} years old.")
这将输出:
My name is Alice and I am 25 years old.
4.2 复杂表达式
你可以在花括号内使用任何有效的Python表达式:
a = 5
b = 10
print(f"The sum of {a} and {b} is {a + b}.")
这将输出:
The sum of 5 and 10 is 15.
4.3 函数调用
你还可以在f-strings中调用函数:
def greet(name):
return f"Hello, {name}!"
name = "Alice"
print(f"Greeting: {greet(name)}")
这将输出:
Greeting: Hello, Alice!
4.4 格式化选项
f-strings还支持格式化选项,例如数字格式化:
value = 1234.56789
print(f"Formatted value: {value:.2f}")
这将输出:
Formatted value: 1234.57
五、字符串操作
Python中的字符串是不可变的,但你可以通过各种操作来创建新的字符串。例如,你可以使用切片、替换和拆分等操作。
5.1 字符串切片
切片允许你提取字符串的子字符串。基本语法如下:
str = "Hello, World!"
substring = str[7:12]
print(substring)
这将输出:
World
5.2 字符串替换
replace()
方法允许你替换字符串中的子字符串:
str = "Hello, World!"
new_str = str.replace("World", "Python")
print(new_str)
这将输出:
Hello, Python!
5.3 字符串拆分
split()
方法允许你将字符串拆分为列表:
str = "Hello, World!"
words = str.split(", ")
print(words)
这将输出:
['Hello', 'World!']
5.4 字符串合并
你可以使用join()
方法将列表合并为字符串:
words = ['Hello', 'World!']
str = ", ".join(words)
print(str)
这将输出:
Hello, World!
六、字符串常见操作
6.1 大小写转换
你可以使用upper()
、lower()
、title()
等方法来转换字符串的大小写:
str = "Hello, World!"
print(str.upper()) # HELLO, WORLD!
print(str.lower()) # hello, world!
print(str.title()) # Hello, World!
6.2 去除空白字符
strip()
、lstrip()
、rstrip()
方法可以去除字符串两端的空白字符:
str = " Hello, World! "
print(str.strip()) # Hello, World!
print(str.lstrip()) # Hello, World!
print(str.rstrip()) # Hello, World!
6.3 查找子字符串
你可以使用find()
、index()
等方法查找子字符串的位置:
str = "Hello, World!"
print(str.find("World")) # 7
print(str.index("World")) # 7
6.4 字符串长度
len()
函数可以用来获取字符串的长度:
str = "Hello, World!"
print(len(str)) # 13
七、字符串编码与解码
在处理文本文件和网络数据时,理解字符串的编码与解码是非常重要的。Python提供了encode()
和decode()
方法来进行编码和解码操作。
7.1 编码字符串
你可以使用encode()
方法将字符串编码为字节对象:
str = "Hello, World!"
encoded_str = str.encode("utf-8")
print(encoded_str) # b'Hello, World!'
7.2 解码字符串
你可以使用decode()
方法将字节对象解码为字符串:
encoded_str = b'Hello, World!'
str = encoded_str.decode("utf-8")
print(str) # Hello, World!
八、字符串模板
Python的string
模块提供了Template
类,可以用来创建和使用字符串模板。这在需要重复使用相同格式的字符串时非常有用。
8.1 创建模板
你可以使用Template
类创建一个字符串模板:
from string import Template
template = Template("Hello, $name!")
8.2 替换占位符
你可以使用substitute()
方法替换模板中的占位符:
print(template.substitute(name="World")) # Hello, World!
8.3 处理缺失的占位符
substitute()
方法在占位符缺失时会抛出异常,你可以使用safe_substitute()
方法来避免这个问题:
print(template.safe_substitute(name="World")) # Hello, World!
print(template.safe_substitute()) # Hello, $name!
九、字符串常见错误及调试
在处理字符串时,可能会遇到一些常见的错误,例如字符串拼接错误、编码错误等。理解这些错误及其调试方法可以帮助你更有效地解决问题。
9.1 字符串拼接错误
常见的字符串拼接错误包括忘记空格或使用错误的拼接方法:
str1 = "Hello"
str2 = "World"
result = str1 + str2 # HelloWorld
解决方法是使用合适的拼接方法,例如使用加号或join()
方法:
result = str1 + " " + str2 # Hello World
result = " ".join([str1, str2]) # Hello World
9.2 编码错误
处理非ASCII字符时,可能会遇到编码错误:
str = "你好"
encoded_str = str.encode("ascii") # UnicodeEncodeError: 'ascii' codec can't encode characters
解决方法是使用合适的编码,例如UTF-8:
encoded_str = str.encode("utf-8") # b'\xe4\xbd\xa0\xe5\xa5\xbd'
9.3 调试方法
在调试字符串相关问题时,可以使用print()
函数或调试器来检查字符串的值和长度:
str = "Hello, World!"
print(str) # Hello, World!
print(len(str)) # 13
十、总结
在这篇文章中,我们详细介绍了如何使用Python字符串打印出来的各种方法,包括print()
函数、格式化字符串、字符串拼接和f-strings。我们还讨论了字符串操作、编码与解码以及字符串模板等高级主题。通过理解和掌握这些方法和技巧,你可以更有效地处理和操作字符串,从而提高代码的可读性和可维护性。
相关问答FAQs:
如何在Python中打印字符串?
在Python中,打印字符串非常简单。可以使用内置的print()
函数。只需将字符串作为参数传递给print()
,例如:print("Hello, World!")
。这段代码将会在控制台上输出“Hello, World!”。
可以在字符串中使用变量吗?
当然可以。在Python中,可以使用格式化字符串或f-string将变量插入到字符串中。例如:
name = "Alice"
print(f"Hello, {name}!") # 输出: Hello, Alice!
这种方式使得字符串的构建更加灵活和易读。
如何打印多行字符串?
要打印多行字符串,可以使用三重引号('''
或"""
)来定义字符串。例如:
print("""这是第一行
这是第二行
这是第三行""")
这样,字符串会按照书写的格式在控制台上逐行显示。
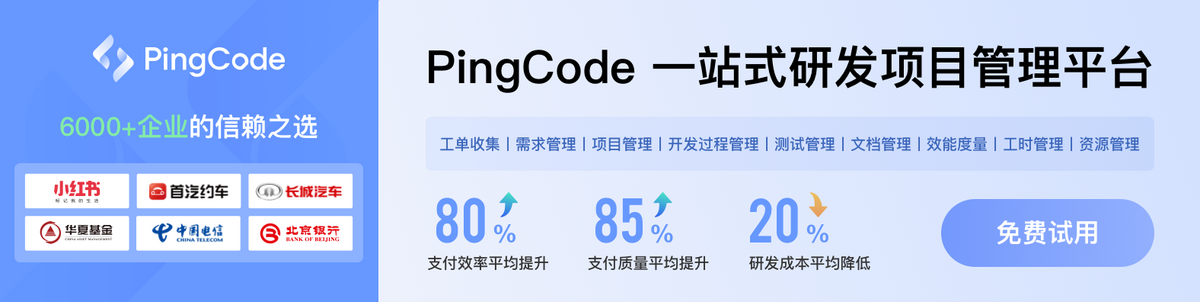