通过在Python折线图上画横线进行对比,可以有效地突出特定数值或区间、帮助分析数据变化趋势、增强数据可视化效果。在本文中,我们将详细介绍如何在Python中使用Matplotlib库来绘制折线图,并添加横线进行对比。
一、准备数据和环境
在开始绘制图形之前,我们需要准备数据和相应的环境。首先,我们需要安装Matplotlib库,这是Python中最常用的数据可视化库之一。可以使用以下命令安装:
pip install matplotlib
安装完成后,我们可以导入必要的库,并准备一些示例数据:
import matplotlib.pyplot as plt
示例数据
x = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
y = [2, 3, 5, 7, 11, 13, 17, 19, 23, 29]
二、绘制基本折线图
要绘制基本的折线图,我们使用Matplotlib的plot()
函数。以下是一个简单的示例代码:
plt.plot(x, y, marker='o')
plt.title('Basic Line Plot')
plt.xlabel('X-axis')
plt.ylabel('Y-axis')
plt.show()
在这个例子中,我们使用plot()
函数绘制了一个折线图,并使用marker
参数在数据点上添加标记。title()
、xlabel()
和ylabel()
函数分别设置图形的标题和轴标签。
三、在折线图上添加横线
为了在折线图上添加横线进行对比,我们可以使用axhline()
函数。这个函数允许我们在特定的y值处绘制一条水平线。以下是一个示例代码:
plt.plot(x, y, marker='o')
plt.axhline(y=10, color='r', linestyle='--', linewidth=2)
plt.title('Line Plot with Horizontal Line')
plt.xlabel('X-axis')
plt.ylabel('Y-axis')
plt.show()
在这个例子中,我们在y=10的位置添加了一条红色的、虚线风格的水平线。color
参数设置线条颜色,linestyle
参数设置线条风格,而linewidth
参数则控制线条的宽度。
四、使用多个横线进行对比
在实际应用中,可能需要在同一张图中添加多条横线,以便进行更详细的对比分析。我们可以多次调用axhline()
函数来实现这一点。以下是一个示例代码:
plt.plot(x, y, marker='o')
plt.axhline(y=10, color='r', linestyle='--', linewidth=2)
plt.axhline(y=20, color='g', linestyle='-.', linewidth=2)
plt.axhline(y=25, color='b', linestyle=':', linewidth=2)
plt.title('Line Plot with Multiple Horizontal Lines')
plt.xlabel('X-axis')
plt.ylabel('Y-axis')
plt.show()
在这个例子中,我们添加了三条水平线,分别位于y=10、y=20和y=25的位置,并使用不同的颜色和风格进行区分。
五、增强图形的可读性
为了增强图形的可读性,我们可以添加图例、注释和其他视觉元素。以下是一些常见的增强技巧:
1. 添加图例
使用legend()
函数可以为图形添加图例,以便更好地解释图形中的各个元素。以下是一个示例代码:
plt.plot(x, y, marker='o', label='Data Line')
plt.axhline(y=10, color='r', linestyle='--', linewidth=2, label='Threshold 10')
plt.axhline(y=20, color='g', linestyle='-.', linewidth=2, label='Threshold 20')
plt.axhline(y=25, color='b', linestyle=':', linewidth=2, label='Threshold 25')
plt.title('Line Plot with Legend')
plt.xlabel('X-axis')
plt.ylabel('Y-axis')
plt.legend()
plt.show()
2. 添加注释
使用annotate()
函数可以在图形上添加注释,以便突出特定的数据点或区域。以下是一个示例代码:
plt.plot(x, y, marker='o')
plt.axhline(y=10, color='r', linestyle='--', linewidth=2)
plt.axhline(y=20, color='g', linestyle='-.', linewidth=2)
plt.axhline(y=25, color='b', linestyle=':', linewidth=2)
plt.title('Line Plot with Annotations')
plt.xlabel('X-axis')
plt.ylabel('Y-axis')
添加注释
plt.annotate('Threshold 10', xy=(1, 10), xytext=(2, 15),
arrowprops=dict(facecolor='black', shrink=0.05))
plt.annotate('Threshold 20', xy=(1, 20), xytext=(2, 25),
arrowprops=dict(facecolor='black', shrink=0.05))
plt.annotate('Threshold 25', xy=(1, 25), xytext=(2, 30),
arrowprops=dict(facecolor='black', shrink=0.05))
plt.show()
在这个例子中,我们在每条水平线附近添加了注释,并使用箭头指向线条的位置。annotate()
函数的xy
参数指定注释指向的位置,而xytext
参数则指定注释文本的位置。
六、案例分析:在实际数据中应用
为了更好地理解如何在实际数据中应用这些技巧,我们可以使用一个具体的案例。例如,我们可以使用股票价格数据来绘制折线图,并添加水平线进行对比分析。
1. 准备数据
首先,我们需要从某个数据源获取股票价格数据。以下是一个示例代码,使用Pandas库从CSV文件中读取数据:
import pandas as pd
读取股票价格数据
data = pd.read_csv('stock_prices.csv')
dates = pd.to_datetime(data['Date'])
prices = data['Close']
2. 绘制折线图和添加水平线
接下来,我们可以使用Matplotlib库绘制折线图,并添加水平线进行对比分析:
plt.plot(dates, prices, marker='o', label='Stock Prices')
plt.axhline(y=100, color='r', linestyle='--', linewidth=2, label='Threshold 100')
plt.axhline(y=150, color='g', linestyle='-.', linewidth=2, label='Threshold 150')
plt.axhline(y=200, color='b', linestyle=':', linewidth=2, label='Threshold 200')
plt.title('Stock Prices with Thresholds')
plt.xlabel('Date')
plt.ylabel('Price')
plt.legend()
plt.show()
3. 添加注释和其他视觉元素
为了增强图形的可读性,我们可以添加注释和其他视觉元素:
plt.plot(dates, prices, marker='o', label='Stock Prices')
plt.axhline(y=100, color='r', linestyle='--', linewidth=2, label='Threshold 100')
plt.axhline(y=150, color='g', linestyle='-.', linewidth=2, label='Threshold 150')
plt.axhline(y=200, color='b', linestyle=':', linewidth=2, label='Threshold 200')
plt.title('Stock Prices with Annotations')
plt.xlabel('Date')
plt.ylabel('Price')
plt.legend()
添加注释
plt.annotate('Threshold 100', xy=(dates[50], 100), xytext=(dates[60], 110),
arrowprops=dict(facecolor='black', shrink=0.05))
plt.annotate('Threshold 150', xy=(dates[50], 150), xytext=(dates[60], 160),
arrowprops=dict(facecolor='black', shrink=0.05))
plt.annotate('Threshold 200', xy=(dates[50], 200), xytext=(dates[60], 210),
arrowprops=dict(facecolor='black', shrink=0.05))
plt.show()
在这个例子中,我们通过添加注释和图例,使图形更加清晰易懂。
七、总结
通过本文的介绍,我们详细讲解了如何在Python中使用Matplotlib库绘制折线图,并添加横线进行对比。首先,我们介绍了如何准备数据和环境,然后展示了绘制基本折线图和添加横线的基本方法。接着,我们讨论了如何使用多个横线进行对比,并介绍了增强图形可读性的一些技巧。最后,我们通过一个实际数据的案例,展示了如何在实际应用中使用这些技巧。
通过掌握这些技巧,您可以在数据可视化中更加有效地分析和展示数据变化趋势,帮助您做出更加准确的决策。无论是在学术研究、商业分析还是日常工作中,这些技巧都将是非常有用的工具。
相关问答FAQs:
如何在Python中绘制折线图并添加横线进行对比?
在Python中,使用Matplotlib库可以轻松绘制折线图并添加横线。您需要先安装Matplotlib库,然后使用plt.axhline()
函数绘制水平线。例如,可以使用以下代码:
import matplotlib.pyplot as plt
# 示例数据
x = [1, 2, 3, 4, 5]
y = [2, 3, 5, 1, 4]
# 绘制折线图
plt.plot(x, y, label='数据线')
# 添加横线进行对比
plt.axhline(y=3, color='r', linestyle='--', label='对比线')
# 添加图例和标题
plt.legend()
plt.title('折线图与对比线')
plt.xlabel('X轴')
plt.ylabel('Y轴')
# 显示图形
plt.show()
可以在折线图上添加多条横线吗?
是的,可以在同一图表中添加多条横线。只需多次调用plt.axhline()
函数,指定不同的y值、颜色和线型。例如,您可以添加一条用于显示目标值的横线,另一条用于显示平均值。
如何定制横线的样式和颜色?
横线的样式和颜色可以通过color
和linestyle
参数进行定制。常见的线型有实线('-'
)、虚线('--'
)、点线(':'
)等。您可以选择自己喜欢的颜色,例如'blue'
、'green'
或'#FF5733'
等,以使图表更具视觉效果。
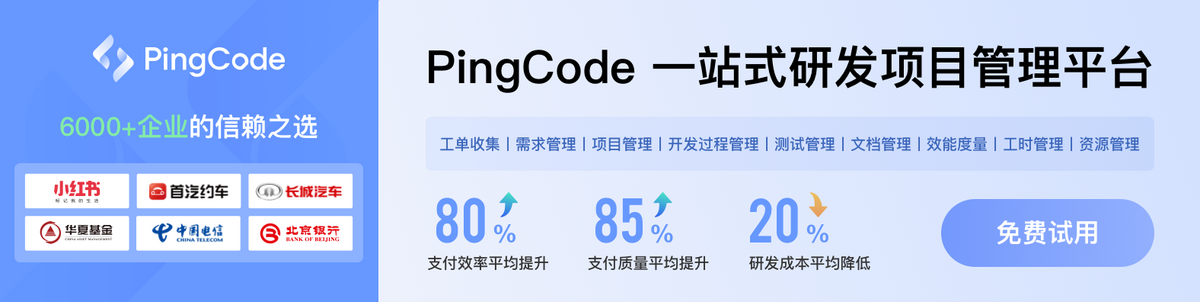