要用Python创建一个文件夹,可以使用os
模块中的os.makedirs()
或os.mkdir()
函数、确保路径的正确性、处理可能出现的错误。 其中,使用os.makedirs()
可以递归地创建多级目录,而os.mkdir()
则只能创建单级目录。为了更好地理解这个过程,我们将详细讲解每个步骤。
一、使用os
模块创建文件夹
Python的os
模块提供了与操作系统交互的功能,其中包括文件和目录操作。os.makedirs()
和os.mkdir()
是两个用于创建目录的函数。
1、os.mkdir()
os.mkdir()
用于创建单个目录。如果指定的目录已经存在,则会引发FileExistsError。
import os
def create_single_directory(path):
try:
os.mkdir(path)
print(f"Directory {path} created successfully")
except FileExistsError:
print(f"Directory {path} already exists")
except Exception as e:
print(f"Error occurred: {e}")
2、os.makedirs()
os.makedirs()
用于创建多级目录。如果其中任何一级目录已经存在,它会自动跳过,不会引发错误。
import os
def create_multiple_directories(path):
try:
os.makedirs(path, exist_ok=True)
print(f"Directories {path} created successfully")
except Exception as e:
print(f"Error occurred: {e}")
二、处理路径和错误
在创建文件夹时,处理路径和潜在的错误是非常重要的。我们可以使用os.path
模块来验证路径的有效性。
1、路径处理
在实际应用中,路径可能会包含用户输入,因此必须验证其有效性。os.path.exists()
可以用来检查路径是否存在。
import os
def create_directory_with_validation(path):
if not os.path.exists(path):
try:
os.makedirs(path)
print(f"Directories {path} created successfully")
except Exception as e:
print(f"Error occurred: {e}")
else:
print(f"Path {path} already exists")
2、错误处理
在实际开发中,处理错误是至关重要的。除了FileExistsError
,还有可能遇到权限错误和路径错误。
import os
def create_directory_with_error_handling(path):
try:
if not os.path.exists(path):
os.makedirs(path)
print(f"Directories {path} created successfully")
else:
print(f"Path {path} already exists")
except PermissionError:
print("Permission denied: You do not have the right permissions to create this directory")
except FileNotFoundError:
print("File not found: The system cannot find the path specified")
except Exception as e:
print(f"An unexpected error occurred: {e}")
三、集成示例
综合以上内容,我们可以编写一个综合示例,展示如何使用Python创建一个文件夹,并处理路径验证和错误。
import os
def create_directory(path):
"""
Create a directory at the specified path, with error handling and path validation.
Parameters:
path (str): The path of the directory to create.
"""
# Validate the path
if not isinstance(path, str) or path.strip() == "":
print("Invalid path: The path must be a non-empty string")
return
# Attempt to create the directory
try:
if not os.path.exists(path):
os.makedirs(path)
print(f"Directories {path} created successfully")
else:
print(f"Path {path} already exists")
except PermissionError:
print("Permission denied: You do not have the right permissions to create this directory")
except FileNotFoundError:
print("File not found: The system cannot find the path specified")
except Exception as e:
print(f"An unexpected error occurred: {e}")
Example usage
create_directory("/path/to/directory")
四、实际应用中的注意事项
1、跨平台兼容性
在不同操作系统中,路径表示方式不同。在Windows中,路径使用反斜杠\
,而在Linux和macOS中,路径使用正斜杠/
。os.path.join()
函数可以帮助我们构建跨平台兼容的路径。
import os
def create_platform_compatible_directory(base_path, folder_name):
path = os.path.join(base_path, folder_name)
create_directory(path)
Example usage
create_platform_compatible_directory("C:\\Users\\User", "NewFolder")
2、权限管理
在某些操作系统或环境中,创建目录可能需要特殊权限。例如,在Linux系统中,某些目录可能需要超级用户权限。可以使用os.access()
检查是否具有所需的权限。
import os
def create_directory_with_permission_check(path):
if os.access(os.path.dirname(path), os.W_OK):
create_directory(path)
else:
print(f"Permission denied: Cannot write to the directory {os.path.dirname(path)}")
Example usage
create_directory_with_permission_check("/path/to/directory")
3、日志记录
为了在生产环境中更好地跟踪和排查问题,可以使用日志记录。Python的logging
模块可以帮助我们记录各种级别的日志信息。
import os
import logging
Configure logging
logging.basicConfig(level=logging.INFO, format='%(asctime)s - %(levelname)s - %(message)s')
def create_directory_with_logging(path):
try:
if not os.path.exists(path):
os.makedirs(path)
logging.info(f"Directories {path} created successfully")
else:
logging.warning(f"Path {path} already exists")
except PermissionError:
logging.error("Permission denied: You do not have the right permissions to create this directory")
except FileNotFoundError:
logging.error("File not found: The system cannot find the path specified")
except Exception as e:
logging.error(f"An unexpected error occurred: {e}")
Example usage
create_directory_with_logging("/path/to/directory")
五、进阶应用
1、创建临时目录
在某些情况下,可能需要创建临时目录用于临时存储数据。Python的tempfile
模块提供了创建临时目录的方法。
import tempfile
def create_temporary_directory():
temp_dir = tempfile.mkdtemp()
print(f"Temporary directory created at {temp_dir}")
return temp_dir
Example usage
temp_dir = create_temporary_directory()
Perform operations in the temporary directory
2、创建目录并设置权限
在创建目录后,可以使用os.chmod()
设置目录的权限。例如,可以设置目录为只读。
import os
def create_directory_with_permissions(path, mode):
try:
if not os.path.exists(path):
os.makedirs(path)
os.chmod(path, mode)
print(f"Directories {path} created successfully with mode {oct(mode)}")
else:
print(f"Path {path} already exists")
except Exception as e:
print(f"An unexpected error occurred: {e}")
Example usage
create_directory_with_permissions("/path/to/directory", 0o755) # Read-write-execute for owner, read-execute for others
六、总结
通过本文,我们详细介绍了如何使用Python创建一个文件夹,包括使用os
模块中的os.mkdir()
和os.makedirs()
函数,路径验证和错误处理,跨平台兼容性,权限管理,日志记录,创建临时目录以及设置目录权限等。Python提供了丰富的标准库,使得文件和目录操作变得非常简便和高效。希望本文能为你在实际开发中提供有用的指导和帮助。
相关问答FAQs:
如何在Python中创建一个多层次的文件夹结构?
在Python中,可以使用os
模块的makedirs
函数来创建多层次的文件夹。该函数允许用户一次性创建多个子文件夹。如果目标文件夹的父文件夹不存在,makedirs
也会自动创建。示例代码如下:
import os
path = 'parent_folder/child_folder/grandchild_folder'
os.makedirs(path)
运行以上代码后,系统会在指定路径下创建完整的文件夹结构。
在创建文件夹时,如何处理已存在的文件夹?
为了避免在创建文件夹时出现错误,可以在使用os.makedirs
函数时设置exist_ok=True
参数。这意味着如果目标文件夹已经存在,程序将不会抛出异常。以下是示例代码:
import os
path = 'existing_folder'
os.makedirs(path, exist_ok=True)
这样可以确保代码的健壮性,避免因为重复创建文件夹而导致的错误。
如何使用Python创建临时文件夹,并确保在使用后自动删除?
Python的tempfile
模块提供了创建临时文件夹的功能。使用TemporaryDirectory
函数可以创建一个临时文件夹,并在退出时自动删除。示例代码如下:
import tempfile
with tempfile.TemporaryDirectory() as temp_dir:
print(f'临时文件夹创建在: {temp_dir}')
# 在这里可以进行文件操作
# 临时文件夹在此处自动删除
这样做不仅简化了临时文件夹的管理,还能有效释放系统资源。
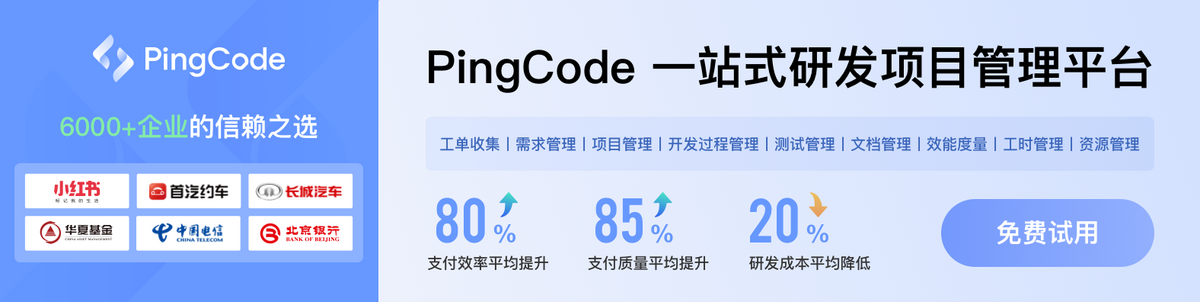