在Python中,将文件移动到指定路径下的方法有多种,常见的方法包括使用shutil模块、os模块、和pathlib模块。其中,使用shutil模块的shutil.move()函数是最常见且高效的方法之一。shutil.move()不仅可以移动文件,还可以移动整个目录。下面详细介绍如何使用shutil.move()函数来移动文件,并讨论其他方法。
一、使用shutil模块移动文件
shutil模块是Python标准库的一部分,提供了一些高级的文件操作功能,包括复制、移动和删除文件及目录。shutil.move()函数可以实现文件的移动。
import shutil
def move_file(source, destination):
try:
shutil.move(source, destination)
print(f"File moved from {source} to {destination}")
except Exception as e:
print(f"Error: {e}")
source = "path/to/source/file.txt"
destination = "path/to/destination/directory"
move_file(source, destination)
在上面的代码中,shutil.move()函数将文件从source路径移动到destination路径。shutil模块的优点在于它简单易用,并且可以处理文件及目录的移动。
二、使用os模块移动文件
os模块是Python标准库中进行操作系统相关操作的模块。虽然os模块没有专门的移动函数,但我们可以使用os.rename()函数来实现文件的移动。
import os
def move_file(source, destination):
try:
os.rename(source, destination)
print(f"File moved from {source} to {destination}")
except Exception as e:
print(f"Error: {e}")
source = "path/to/source/file.txt"
destination = "path/to/destination/directory/file.txt"
move_file(source, destination)
在上面的代码中,os.rename()函数将文件从source路径移动到destination路径。与shutil.move()不同,os.rename()只适用于在同一个文件系统内移动文件。
三、使用pathlib模块移动文件
pathlib模块提供了面向对象的路径操作方法,自Python 3.4起成为标准库的一部分。我们可以使用pathlib模块中的Path对象和rename()方法来移动文件。
from pathlib import Path
def move_file(source, destination):
try:
source_path = Path(source)
destination_path = Path(destination)
source_path.rename(destination_path)
print(f"File moved from {source} to {destination}")
except Exception as e:
print(f"Error: {e}")
source = "path/to/source/file.txt"
destination = "path/to/destination/directory/file.txt"
move_file(source, destination)
在上面的代码中,Path().rename()方法将文件从source路径移动到destination路径。pathlib模块的优点在于其面向对象的路径操作方式,更加直观和易读。
四、处理文件路径和错误
在文件移动过程中,处理文件路径和错误是非常重要的。我们需要确保源文件存在,并且目标路径有效。同时,还需要处理文件已存在的情况,避免覆盖重要文件。
import shutil
from pathlib import Path
def move_file(source, destination):
try:
source_path = Path(source)
destination_path = Path(destination)
if not source_path.exists():
raise FileNotFoundError(f"Source file {source} does not exist")
if destination_path.exists():
raise FileExistsError(f"Destination file {destination} already exists")
shutil.move(source, destination)
print(f"File moved from {source} to {destination}")
except Exception as e:
print(f"Error: {e}")
source = "path/to/source/file.txt"
destination = "path/to/destination/directory/file.txt"
move_file(source, destination)
在上面的代码中,我们使用了Path().exists()方法来检查源文件和目标文件是否存在,并相应地抛出错误。
五、跨平台文件移动
在实际应用中,我们可能需要在不同操作系统之间移动文件。Python的shutil模块、os模块和pathlib模块都是跨平台的,能够在Windows、macOS和Linux系统上正常工作。
import shutil
import os
from pathlib import Path
def move_file(source, destination):
try:
source_path = Path(source)
destination_path = Path(destination)
if not source_path.exists():
raise FileNotFoundError(f"Source file {source} does not exist")
if destination_path.exists():
raise FileExistsError(f"Destination file {destination} already exists")
shutil.move(source, destination)
print(f"File moved from {source} to {destination}")
except Exception as e:
print(f"Error: {e}")
source = "path/to/source/file.txt"
destination = "path/to/destination/directory/file.txt"
move_file(source, destination)
在上面的代码中,我们使用了shutil.move()函数来移动文件,确保代码在不同操作系统上都能正常运行。
六、日志记录和异常处理
在实际应用中,日志记录和异常处理是非常重要的。我们可以使用Python的logging模块来记录文件移动操作的日志,方便后续的调试和维护。
import shutil
import logging
from pathlib import Path
logging.basicConfig(level=logging.INFO, format='%(asctime)s - %(levelname)s - %(message)s')
def move_file(source, destination):
try:
source_path = Path(source)
destination_path = Path(destination)
if not source_path.exists():
raise FileNotFoundError(f"Source file {source} does not exist")
if destination_path.exists():
raise FileExistsError(f"Destination file {destination} already exists")
shutil.move(source, destination)
logging.info(f"File moved from {source} to {destination}")
except Exception as e:
logging.error(f"Error: {e}")
source = "path/to/source/file.txt"
destination = "path/to/destination/directory/file.txt"
move_file(source, destination)
在上面的代码中,我们使用了logging模块来记录文件移动操作的日志,包括成功移动文件和异常情况。这样可以方便后续的调试和维护。
七、批量移动文件
在实际应用中,我们可能需要批量移动文件。我们可以使用循环来遍历文件列表,并使用shutil.move()函数来移动每个文件。
import shutil
import logging
from pathlib import Path
logging.basicConfig(level=logging.INFO, format='%(asctime)s - %(levelname)s - %(message)s')
def move_files(file_list, destination):
for file in file_list:
try:
source_path = Path(file)
destination_path = Path(destination) / source_path.name
if not source_path.exists():
raise FileNotFoundError(f"Source file {file} does not exist")
if destination_path.exists():
raise FileExistsError(f"Destination file {destination_path} already exists")
shutil.move(file, destination_path)
logging.info(f"File moved from {file} to {destination_path}")
except Exception as e:
logging.error(f"Error: {e}")
file_list = ["path/to/source/file1.txt", "path/to/source/file2.txt", "path/to/source/file3.txt"]
destination = "path/to/destination/directory"
move_files(file_list, destination)
在上面的代码中,我们使用了循环来遍历文件列表,并使用shutil.move()函数来移动每个文件,同时记录日志。
八、总结
通过本文的介绍,我们详细讨论了在Python中将文件移动到指定路径下的多种方法,包括使用shutil模块、os模块、和pathlib模块。我们还介绍了如何处理文件路径和错误,跨平台文件移动,日志记录和异常处理,以及批量移动文件。
无论是简单的文件移动还是复杂的批量操作,Python都提供了丰富的工具和模块来帮助我们实现这些任务。希望本文能够对您在实际应用中有所帮助。
相关问答FAQs:
如何在Python中实现文件的移动功能?
在Python中,可以使用内置的shutil
模块来移动文件。具体方法是调用shutil.move(source, destination)
函数,其中source
是要移动的文件路径,destination
是目标路径。这个函数会将文件从源路径移动到指定的目标路径,并且会覆盖同名文件。
在移动文件时,如何处理文件名冲突?
如果目标路径下已存在同名文件,使用shutil.move()
函数时,默认情况下会覆盖该文件。如果你希望在移动时避免文件覆盖,可以在移动之前检查目标路径下是否已有同名文件,并根据情况重命名源文件或者选择另一个目标路径。
移动文件时,如何处理文件权限问题?
在移动文件过程中,可能会遇到权限问题。如果你没有足够的权限去访问源文件或者写入目标路径,Python会抛出PermissionError
异常。确保在移动文件之前,你有适当的权限。如果需要,可以使用os.chmod()
调整文件权限,或者在管理员模式下运行Python脚本。
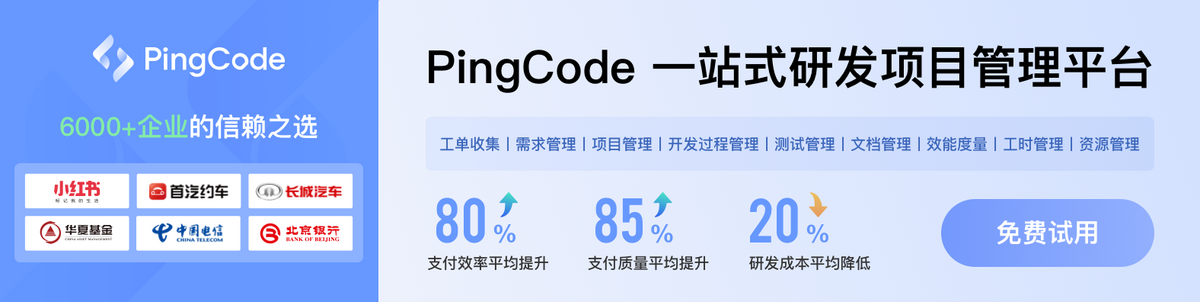