在Python中将结果写到txt文件中可以使用open
函数创建或打开一个文件,使用write
方法将内容写入文件,并使用close
方法关闭文件。使用with语句可以自动管理文件资源。接下来,我将详细展开其中一个方法,即使用with
语句的方式来将结果写到txt文件中。
使用with
语句的优势在于它能够自动管理文件资源,无需显式调用close
方法,这样可以有效避免文件泄露问题。通过with
语句,我们可以确保在代码块执行完毕后,文件会自动关闭。下面是一个简单的示例:
with open('output.txt', 'w') as file:
file.write('Hello, World!')
在这个示例中,open
函数以写模式('w')打开了一个名为output.txt
的文件,并返回一个文件对象。write
方法将字符串'Hello, World!'
写入文件。完成后,with
语句会自动关闭文件。
接下来,我将详细介绍Python中将结果写到txt文件中的多种方法和最佳实践。
一、基本文件操作
1. 使用open
函数和write
方法
Python中的open
函数是文件操作的基础。以下是使用open
函数和write
方法写入文件的步骤:
# 打开文件(如果文件不存在,则会创建一个)
file = open('output.txt', 'w')
写入内容
file.write('Hello, World!')
关闭文件
file.close()
2. 使用with
语句
正如前面所提到的,with
语句可以简化文件操作,并确保文件在使用后正确关闭:
with open('output.txt', 'w') as file:
file.write('Hello, World!')
二、文件模式
1. 写入模式('w')
写入模式会覆盖文件中的现有内容:
with open('output.txt', 'w') as file:
file.write('This will overwrite the existing content.')
2. 追加模式('a')
追加模式会在文件末尾添加新内容,而不会覆盖现有内容:
with open('output.txt', 'a') as file:
file.write('This content will be appended to the file.\n')
3. 读取模式('r')
读取模式用于从文件中读取内容:
with open('output.txt', 'r') as file:
content = file.read()
print(content)
三、写入多行内容
1. 使用write
方法逐行写入
lines = ['First line\n', 'Second line\n', 'Third line\n']
with open('output.txt', 'w') as file:
for line in lines:
file.write(line)
2. 使用writelines
方法
writelines
方法可以将一个字符串列表写入文件:
lines = ['First line\n', 'Second line\n', 'Third line\n']
with open('output.txt', 'w') as file:
file.writelines(lines)
四、格式化写入内容
1. 使用字符串格式化
通过字符串格式化可以更灵活地写入内容:
name = 'Alice'
age = 30
with open('output.txt', 'w') as file:
file.write(f'Name: {name}, Age: {age}\n')
2. 使用str.format
方法
name = 'Alice'
age = 30
with open('output.txt', 'w') as file:
file.write('Name: {}, Age: {}\n'.format(name, age))
五、读取文件内容并写入另一个文件
可以将一个文件的内容读取出来,并写入到另一个文件中:
# 读取文件内容
with open('input.txt', 'r') as infile:
content = infile.read()
写入到另一个文件
with open('output.txt', 'w') as outfile:
outfile.write(content)
六、处理大文件
当处理大文件时,逐行读取和写入是一个更高效的方法:
# 逐行读取和写入
with open('input.txt', 'r') as infile, open('output.txt', 'w') as outfile:
for line in infile:
outfile.write(line)
七、处理不同编码格式
在处理包含不同字符编码的文件时,可以指定编码格式:
with open('output.txt', 'w', encoding='utf-8') as file:
file.write('这是一些中文内容\n')
八、异常处理
在文件操作中,添加异常处理可以确保代码的健壮性:
try:
with open('output.txt', 'w') as file:
file.write('This is some content.\n')
except IOError as e:
print(f'An error occurred: {e}')
九、使用第三方库
除了Python内置的文件操作方法,还有一些第三方库可以简化文件操作,例如pathlib
库:
from pathlib import Path
创建文件路径对象
file_path = Path('output.txt')
写入文件
file_path.write_text('Hello, World!')
十、总结
Python提供了丰富且灵活的文件操作方法,可以满足不同场景下的需求。使用open
函数和with
语句是最基本也是最常用的方式,能够确保文件资源得到正确管理。通过掌握不同的文件模式、格式化写入、多行写入、处理大文件、处理不同编码格式以及异常处理等技巧,能够提高代码的健壮性和可读性。
此外,使用第三方库如pathlib
可以进一步简化文件操作。无论是简单的文件写入,还是复杂的文件操作需求,Python都能够提供有效的解决方案。掌握这些方法和技巧,将帮助你在实际开发中更加高效地处理文件操作。
相关问答FAQs:
如何在Python中创建并写入一个新的txt文件?
在Python中,可以使用内置的open()
函数创建一个新的txt文件并写入内容。使用'w'
模式打开文件,如果文件不存在则会创建它。如果文件已存在,内容会被覆盖。示例代码如下:
with open('output.txt', 'w') as file:
file.write('这是写入文件的内容。')
Python中如何追加内容到已存在的txt文件?
为了向一个已经存在的txt文件中追加内容,可以使用'a'
模式打开文件。这样,写入的内容将添加到文件的末尾,而不会覆盖原有内容。示例代码如下:
with open('output.txt', 'a') as file:
file.write('\n这是追加到文件的新内容。')
在Python中如何写入多行内容到txt文件?
若想在txt文件中写入多行内容,可以使用writelines()
方法,传入一个包含每行内容的列表。确保每行末尾有换行符。示例代码如下:
lines = ['第一行\n', '第二行\n', '第三行\n']
with open('output.txt', 'w') as file:
file.writelines(lines)
这样就可以在txt文件中写入多行内容了。
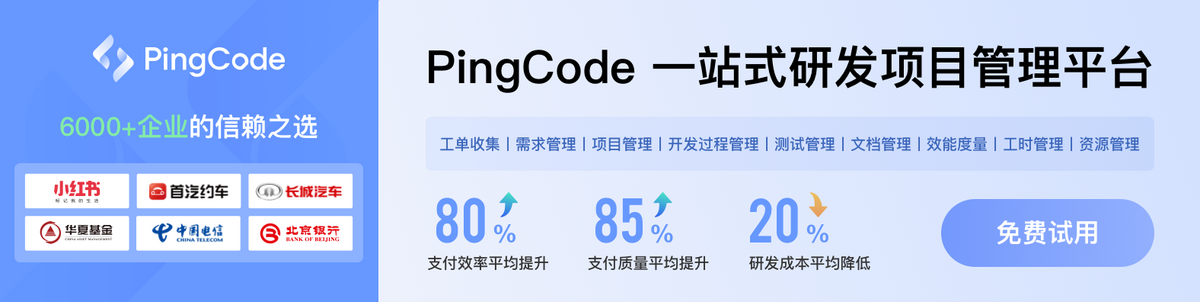