在Python中输出带有值的字符串,可以使用字符串格式化、f-string、以及字符串拼接等方法。 推荐使用f-string、format()方法。
字符串格式化方法
一、f-string方法
f-string是Python 3.6引入的一种新的字符串格式化方法。f-string使用大括号{}来包含表达式,前面加上字母f或F即可。在大括号内,可以放置变量、表达式或函数调用,Python会将其结果插入到字符串中。
name = "Alice"
age = 30
formatted_string = f"My name is {name} and I am {age} years old."
print(formatted_string)
在上面的例子中,name
和age
变量的值会被插入到字符串中,生成的字符串为"My name is Alice and I am 30 years old."
。f-string的方法不仅简洁,而且效率高,是推荐的字符串格式化方法。
二、format()方法
format()
是Python 2.7和3.x的一个方法,它利用大括号{}作为占位符,然后通过str.format()
方法将变量或表达式的值插入到字符串中。这种方法允许在同一个字符串中插入多个值,并且可以控制插入位置和格式。
name = "Bob"
age = 25
formatted_string = "My name is {} and I am {} years old.".format(name, age)
print(formatted_string)
在上面的例子中,name
和age
变量的值会被插入到字符串中,生成的字符串为"My name is Bob and I am 25 years old."
。format()
方法的一个优点是可以通过位置参数或关键字参数来控制插入的值。
字符串拼接方法
三、使用加号进行拼接
最简单的字符串拼接方法是使用加号+
将多个字符串连接起来。这种方法适用于拼接少量字符串,代码可读性较差。
name = "Charlie"
age = 35
formatted_string = "My name is " + name + " and I am " + str(age) + " years old."
print(formatted_string)
在上面的例子中,使用加号将多个字符串和变量连接起来,生成的字符串为"My name is Charlie and I am 35 years old."
。需要注意的是,必须将非字符串类型的变量转换为字符串类型。
四、使用百分号进行格式化
百分号%
是一种旧式的字符串格式化方法,类似于C语言中的printf
。这种方法使用%
作为占位符,后面跟一个元组,包含要插入的值。
name = "David"
age = 40
formatted_string = "My name is %s and I am %d years old." % (name, age)
print(formatted_string)
在上面的例子中,%s
和%d
分别是字符串和整数的占位符,生成的字符串为"My name is David and I am 40 years old."
。这种方法的缺点是代码可读性较差,并且容易出错。
详细描述f-string方法
f-string是Python 3.6引入的一种新的字符串格式化方法,其全称为"formatted string literals"。相较于其他字符串格式化方法,f-string具有以下优点:
- 简洁明了:f-string语法简洁,容易理解,不需要额外的方法调用或占位符。
- 高效:f-string在运行时直接插入变量值,不需要额外的格式化操作,性能较高。
- 灵活:f-string支持在大括号内嵌入任意表达式,包括函数调用和计算结果。
- 可读性强:f-string语法清晰,代码可读性高,便于维护。
下面是一个使用f-string的例子,演示了如何在字符串中插入变量、表达式和函数调用:
name = "Eve"
age = 28
height = 1.75
weight = 65
def bmi(weight, height):
return weight / (height 2)
formatted_string = (f"My name is {name}, I am {age} years old, "
f"my height is {height} meters, "
f"my weight is {weight} kilograms, "
f"and my BMI is {bmi(weight, height):.2f}.")
print(formatted_string)
在上面的例子中,f-string不仅插入了name
、age
、height
和weight
变量的值,还插入了bmi
函数的计算结果,并且格式化为小数点后两位。生成的字符串为"My name is Eve, I am 28 years old, my height is 1.75 meters, my weight is 65 kilograms, and my BMI is 21.22."
。
综上所述,f-string是Python中推荐的字符串格式化方法,具有简洁、高效、灵活和可读性强的优点。通过f-string,开发者可以方便地在字符串中插入变量、表达式和函数调用的结果,从而提高代码的可读性和维护性。
相关问答FAQs:
如何在Python中将变量值插入字符串中?
在Python中,可以使用多种方法将变量值插入字符串。最常用的方法包括使用f-string(格式化字符串),str.format()
方法,以及百分号格式化。例如,使用f-string时,可以这样写:name = "Alice"; print(f"Hello, {name}!")
,这将输出Hello, Alice!
。
Python中是否可以通过字符串连接来输出带值的字符串?
当然可以。在Python中,可以使用加号运算符进行字符串连接。例如,name = "Alice"; greeting = "Hello, " + name + "!"; print(greeting)
,这样也会输出Hello, Alice!
。不过需要注意,使用加号连接多个字符串时,可能会影响性能,特别是在处理大量数据时。
在Python中,如何格式化输出多个变量到字符串中?
可以使用f-string或str.format()
方法来格式化多个变量。例如,使用f-string时,可以这样写:name = "Alice"; age = 30; print(f"{name} is {age} years old.")
,输出结果为Alice is 30 years old.
。如果选择str.format()
方法,则可以这样写:print("{} is {} years old.".format(name, age))
,效果相同。选择合适的方法可以使代码更清晰易读。
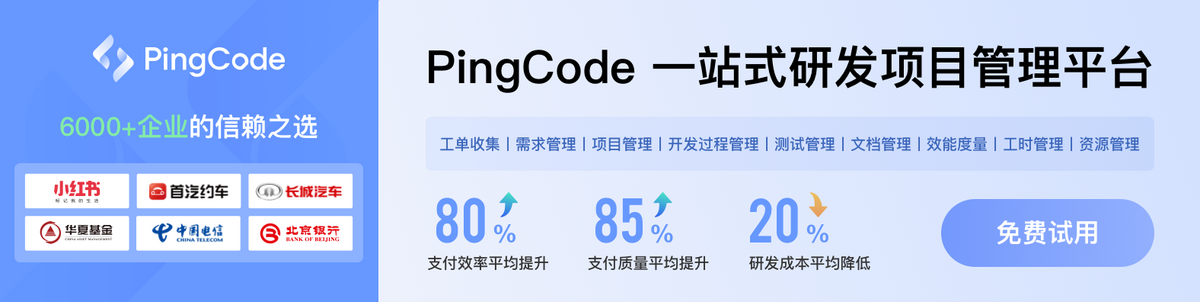