Python 如何将 log 文件信息展示出来
使用 Python 读取 log 文件信息并展示,可以通过以下几种方式实现:使用内置的 open() 函数读取文件、使用 logging 模块进行日志管理、借助第三方库如 pandas 进行数据分析和展示。 在这篇文章中,我们将详细探讨这些方法,并提供代码示例和最佳实践。
一、使用内置的 open() 函数读取文件
1.1 简单读取和显示
使用 Python 的内置函数 open() 可以非常方便地读取文本文件,包括 log 文件。以下是一个简单的示例,展示如何读取和打印 log 文件的内容:
def read_log_file(file_path):
with open(file_path, 'r') as file:
for line in file:
print(line.strip())
read_log_file('example.log')
这个示例展示了如何逐行读取 log 文件并将其内容打印到控制台。使用 with open()
的方式可以确保文件在读取完成后自动关闭,避免文件句柄泄漏。
1.2 文件过滤和格式化输出
在实际应用中,我们可能需要对 log 文件进行过滤和格式化输出。以下示例展示了如何过滤特定级别的日志信息,并将其格式化输出:
def filter_and_format_log(file_path, log_level):
with open(file_path, 'r') as file:
for line in file:
if log_level in line:
formatted_line = format_log_line(line)
print(formatted_line)
def format_log_line(line):
# 这里可以添加更多的格式化逻辑
return line.strip()
filter_and_format_log('example.log', 'ERROR')
二、使用 logging 模块进行日志管理
Python 的 logging 模块是一个功能强大的日志管理工具,它不仅可以创建日志文件,还可以读取和展示日志信息。
2.1 创建和配置日志文件
首先,我们需要创建并配置日志文件。以下示例展示了如何使用 logging 模块创建一个日志文件,并记录不同级别的日志信息:
import logging
def setup_logging():
logging.basicConfig(
filename='example.log',
level=logging.DEBUG,
format='%(asctime)s %(levelname)s: %(message)s',
datefmt='%Y-%m-%d %H:%M:%S'
)
def log_messages():
logging.debug('This is a debug message')
logging.info('This is an info message')
logging.warning('This is a warning message')
logging.error('This is an error message')
logging.critical('This is a critical message')
setup_logging()
log_messages()
2.2 读取和展示日志信息
配置好日志文件后,我们可以使用 logging 模块中的 FileHandler 读取日志文件,并将其内容展示出来:
import logging
def read_log_file_with_logging(file_path):
logger = logging.getLogger()
handler = logging.FileHandler(file_path, 'r')
handler.setLevel(logging.DEBUG)
formatter = logging.Formatter('%(asctime)s %(levelname)s: %(message)s')
handler.setFormatter(formatter)
logger.addHandler(handler)
with open(file_path, 'r') as file:
for line in file:
print(line.strip())
read_log_file_with_logging('example.log')
三、借助第三方库进行数据分析和展示
对于复杂的 log 文件分析和展示需求,我们可以借助第三方库如 pandas。这些库可以帮助我们更高效地处理和展示日志数据。
3.1 使用 pandas 读取 log 文件
pandas 是一个强大的数据分析工具,适用于处理结构化数据。以下示例展示了如何使用 pandas 读取和展示 log 文件中的数据:
import pandas as pd
def read_log_with_pandas(file_path):
log_data = pd.read_csv(file_path, sep=' ', header=None, names=['Date', 'Time', 'Level', 'Message'])
print(log_data)
read_log_with_pandas('example.log')
3.2 数据过滤和可视化
pandas 提供了强大的数据过滤和可视化功能。以下示例展示了如何过滤特定级别的日志信息,并使用 matplotlib 进行数据可视化:
import pandas as pd
import matplotlib.pyplot as plt
def filter_and_visualize_logs(file_path, log_level):
log_data = pd.read_csv(file_path, sep=' ', header=None, names=['Date', 'Time', 'Level', 'Message'])
filtered_data = log_data[log_data['Level'] == log_level]
print(filtered_data)
# 可视化日志数据
filtered_data['DateTime'] = pd.to_datetime(filtered_data['Date'] + ' ' + filtered_data['Time'])
filtered_data.set_index('DateTime', inplace=True)
filtered_data['Message'].resample('D').count().plot(kind='bar')
plt.show()
filter_and_visualize_logs('example.log', 'ERROR')
四、处理大文件和性能优化
在处理大文件时,性能可能成为一个瓶颈。以下是一些优化建议:
4.1 分批次读取文件
对于非常大的 log 文件,可以分批次读取,以减少内存占用:
def read_large_log_file(file_path, batch_size=1000):
with open(file_path, 'r') as file:
batch = []
for line in file:
batch.append(line.strip())
if len(batch) >= batch_size:
process_batch(batch)
batch = []
if batch:
process_batch(batch)
def process_batch(batch):
for line in batch:
print(line)
read_large_log_file('example.log')
4.2 多线程和多进程
对于需要并行处理的任务,可以使用多线程或多进程来提高性能:
import threading
def read_log_file_in_threads(file_path, num_threads=4):
with open(file_path, 'r') as file:
lines = file.readlines()
def worker(lines):
for line in lines:
print(line.strip())
chunk_size = len(lines) // num_threads
threads = []
for i in range(num_threads):
start = i * chunk_size
end = (i + 1) * chunk_size if i != num_threads - 1 else len(lines)
thread = threading.Thread(target=worker, args=(lines[start:end],))
threads.append(thread)
thread.start()
for thread in threads:
thread.join()
read_log_file_in_threads('example.log')
五、日志分析的高级技巧
5.1 日志聚合和统计
日志聚合和统计是日志分析中的重要部分。以下示例展示了如何使用 pandas 对日志数据进行聚合和统计:
import pandas as pd
def aggregate_and_stat_logs(file_path):
log_data = pd.read_csv(file_path, sep=' ', header=None, names=['Date', 'Time', 'Level', 'Message'])
# 聚合统计不同级别的日志数量
log_counts = log_data['Level'].value_counts()
print(log_counts)
# 按日期聚合统计
log_data['Date'] = pd.to_datetime(log_data['Date'])
daily_log_counts = log_data.groupby(log_data['Date'].dt.date)['Level'].value_counts()
print(daily_log_counts)
aggregate_and_stat_logs('example.log')
5.2 事件关联分析
事件关联分析可以帮助我们发现日志中的潜在问题和关联事件。以下示例展示了如何进行简单的事件关联分析:
import pandas as pd
def event_correlation_analysis(file_path):
log_data = pd.read_csv(file_path, sep=' ', header=None, names=['Date', 'Time', 'Level', 'Message'])
# 添加日期时间列
log_data['DateTime'] = pd.to_datetime(log_data['Date'] + ' ' + log_data['Time'])
# 按时间排序
log_data.sort_values('DateTime', inplace=True)
# 计算相邻事件的时间差
log_data['TimeDiff'] = log_data['DateTime'].diff()
# 筛选出时间差小于某个阈值的事件
threshold = pd.Timedelta(seconds=30)
correlated_events = log_data[log_data['TimeDiff'] < threshold]
print(correlated_events)
event_correlation_analysis('example.log')
总结
在这篇文章中,我们详细探讨了如何使用 Python 读取和展示 log 文件信息。我们介绍了使用内置的 open() 函数、logging 模块以及第三方库 pandas 进行日志管理和展示的方法,并提供了多个代码示例。最后,我们探讨了处理大文件和性能优化的技巧,以及日志分析的高级技巧。希望这篇文章能够帮助你更好地理解和应用 Python 进行日志文件的读取和展示。
相关问答FAQs:
如何使用Python读取和分析log文件中的数据?
可以使用Python的内置模块,如os
和re
,来读取log文件。os
模块可用于文件路径操作,而re
模块则可以帮助提取特定的信息。通过逐行读取文件,可以使用正则表达式匹配感兴趣的日志条目,从而实现数据分析和展示。
Python中有哪些库可以帮助处理log文件?
一些常用的Python库包括pandas
、loguru
和logging
。pandas
可以方便地将log数据转化为DataFrame格式,便于数据分析和可视化;loguru
提供了简单易用的日志记录功能,同时支持多种输出格式;logging
模块则是Python的标准库,适合用来创建和管理自己的日志系统。
如何将log文件信息以图表形式展示?
可以使用matplotlib
或seaborn
等可视化库来将log信息以图表形式展示。首先,使用Python读取log文件并提取需要的数据信息,然后利用这些库绘制条形图、折线图或饼图等,帮助更直观地理解log文件中的数据趋势和分布。
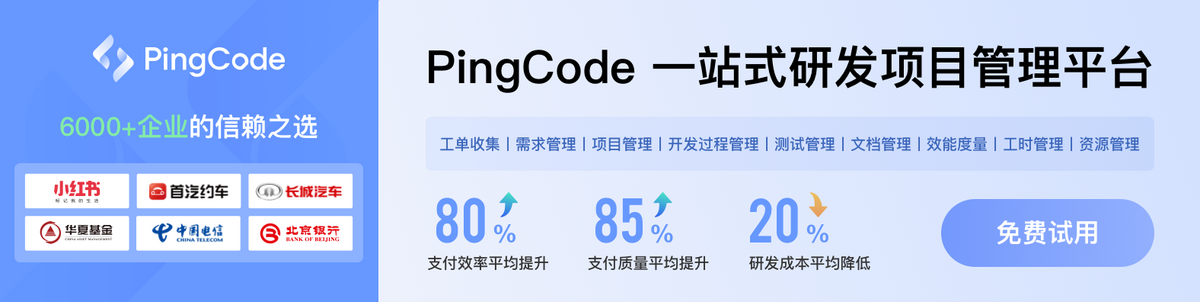