知道图片地址如何用Python下载文件:
使用requests库、处理异常、保存文件到本地
在这篇文章中,我们将详细讨论如何使用Python下载图片文件。Python提供了多种库和方法来实现这一任务,其中最常用的包括requests
库和urllib
库。本文将详细介绍如何使用这些工具,并讨论一些常见问题和解决方案。
一、使用requests库
1. 安装requests库
首先,我们需要安装requests
库。你可以通过以下命令安装:
pip install requests
2. 下载图片文件
使用requests
库下载图片文件非常简单。我们可以通过以下代码实现:
import requests
def download_image(url, save_path):
response = requests.get(url)
if response.status_code == 200:
with open(save_path, 'wb') as f:
f.write(response.content)
else:
print(f"Failed to retrieve image. Status code: {response.status_code}")
示例使用
download_image('https://example.com/image.jpg', 'image.jpg')
在这个示例中,我们定义了一个名为download_image
的函数,该函数接受两个参数:图片的URL和保存路径。我们使用requests.get()
方法发送HTTP GET请求以获取图片文件,并检查响应状态码。如果状态码为200(表示请求成功),我们将图片内容写入本地文件。
二、处理异常
在实际应用中,我们可能会遇到各种异常情况,例如网络连接错误、超时等。我们可以通过捕获异常来处理这些情况,以提高代码的鲁棒性。
import requests
from requests.exceptions import RequestException
def download_image(url, save_path):
try:
response = requests.get(url, timeout=10)
response.raise_for_status()
with open(save_path, 'wb') as f:
f.write(response.content)
except RequestException as e:
print(f"An error occurred: {e}")
示例使用
download_image('https://example.com/image.jpg', 'image.jpg')
在这个示例中,我们使用try
和except
块来捕获可能的异常。我们还设置了请求超时时间(例如10秒),以防止请求无限期挂起。
三、保存文件到本地
在保存文件时,我们可以根据需要指定保存路径和文件名。为了确保文件路径的有效性,我们可以使用os
库来处理文件路径。
import requests
import os
from requests.exceptions import RequestException
def download_image(url, save_path):
try:
response = requests.get(url, timeout=10)
response.raise_for_status()
os.makedirs(os.path.dirname(save_path), exist_ok=True)
with open(save_path, 'wb') as f:
f.write(response.content)
except RequestException as e:
print(f"An error occurred: {e}")
示例使用
download_image('https://example.com/image.jpg', 'images/image.jpg')
在这个示例中,我们使用os.makedirs()
函数来创建保存路径所需的目录。如果目录已经存在,exist_ok=True
参数将防止抛出异常。
四、使用urllib库
除了requests
库,我们还可以使用Python标准库中的urllib
库来下载图片文件。urllib
库是Python内置库,无需额外安装。
1. 使用urlretrieve方法
urllib
库提供了一个名为urlretrieve
的方法,可以直接将远程文件下载到本地。
import urllib.request
def download_image(url, save_path):
try:
urllib.request.urlretrieve(url, save_path)
except Exception as e:
print(f"An error occurred: {e}")
示例使用
download_image('https://example.com/image.jpg', 'image.jpg')
在这个示例中,我们使用urllib.request.urlretrieve()
方法下载图片文件,并捕获可能的异常。
2. 使用urlopen方法
我们还可以使用urlopen
方法手动处理HTTP请求和响应。
import urllib.request
def download_image(url, save_path):
try:
with urllib.request.urlopen(url) as response:
with open(save_path, 'wb') as f:
f.write(response.read())
except Exception as e:
print(f"An error occurred: {e}")
示例使用
download_image('https://example.com/image.jpg', 'image.jpg')
在这个示例中,我们使用urllib.request.urlopen()
方法发送HTTP GET请求,并使用read()
方法读取响应内容。
五、处理大文件下载
在处理大文件下载时,我们可以通过分块读取响应内容,以避免占用过多内存。
import requests
from requests.exceptions import RequestException
def download_image(url, save_path):
try:
response = requests.get(url, stream=True, timeout=10)
response.raise_for_status()
with open(save_path, 'wb') as f:
for chunk in response.iter_content(chunk_size=8192):
if chunk:
f.write(chunk)
except RequestException as e:
print(f"An error occurred: {e}")
示例使用
download_image('https://example.com/large_image.jpg', 'large_image.jpg')
在这个示例中,我们使用stream=True
参数发送流式请求,并通过iter_content()
方法分块读取响应内容。这样可以有效减少内存占用,适用于下载大文件。
六、总结
在本文中,我们详细讨论了如何使用Python下载图片文件。我们介绍了使用requests
库和urllib
库的方法,并讨论了处理异常、保存文件到本地和处理大文件下载等内容。通过这些方法,你可以轻松地实现图片文件的下载,并根据实际需求进行调整。
了解更多Python库和方法的使用,可以帮助你在处理网络请求和文件下载时更加得心应手。如果你有任何疑问或建议,欢迎在评论区留言。
相关问答FAQs:
如何使用Python下载图片文件?
可以使用Python的requests
库来下载图片。首先,确保安装了requests
库。接着,使用requests.get()
方法获取图片内容,并将其写入文件中。例如:
import requests
url = '图片地址'
response = requests.get(url)
with open('图片文件名.jpg', 'wb') as file:
file.write(response.content)
确保替换图片地址
和图片文件名.jpg
为实际的图片链接和您希望保存的文件名。
在下载图片时,如何处理可能出现的错误?
在下载过程中,可能会遇到网络问题或文件保存权限问题。为了处理这些错误,可以使用try
和except
语句。以下是一个示例:
try:
response = requests.get(url)
response.raise_for_status() # 检查请求是否成功
with open('图片文件名.jpg', 'wb') as file:
file.write(response.content)
except requests.exceptions.RequestException as e:
print(f"下载失败: {e}")
这样可以确保在发生错误时能够捕获并进行相应处理。
下载的图片如何在本地查看?
下载完图片后,可以使用多种方式在本地查看。常见的方法包括使用操作系统自带的图片查看器,或者使用Python的图形库,例如PIL
(Pillow)库。以下是一个使用PIL
库查看图片的示例:
from PIL import Image
image = Image.open('图片文件名.jpg')
image.show()
确保安装了Pillow
库,这样您就可以直接在Python中查看下载的图片了。
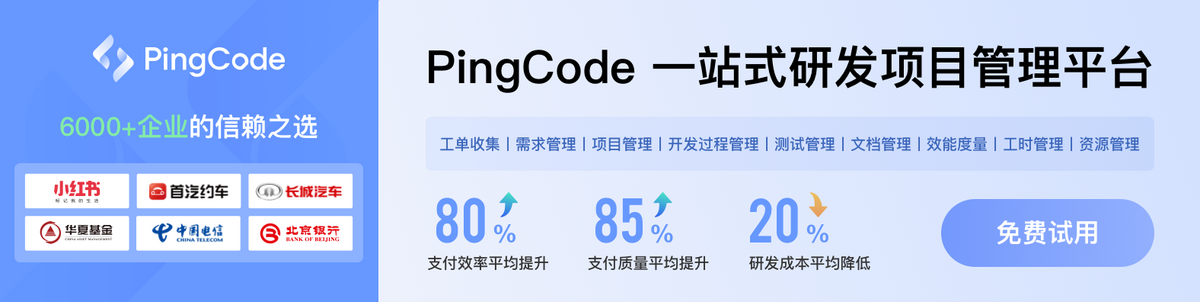