要获取东方财富网的股票代码,可以通过以下几种方法: 使用网页爬虫、利用API接口、东方财富网数据导出功能。其中,利用API接口是最为便捷和高效的方法之一。东方财富网提供了丰富的数据接口,可以方便地获取股票代码及其他相关数据。下面我们将详细介绍如何通过Python实现这几种方法,尤其是利用API接口的方法。
一、使用网页爬虫
1. 基本概念
网页爬虫是一种自动化的工具,用于从网页上提取数据。Python提供了多种库来实现网页爬虫功能,其中最常用的是requests和BeautifulSoup。
2. 安装相关库
首先,你需要安装这两个库,可以通过pip安装:
pip install requests
pip install beautifulsoup4
3. 编写爬虫代码
以下是一个简单的示例代码,用于从东方财富网获取股票代码:
import requests
from bs4 import BeautifulSoup
def get_stock_codes():
url = 'http://quote.eastmoney.com/stocklist.html'
response = requests.get(url)
response.encoding = 'gb2312'
soup = BeautifulSoup(response.text, 'html.parser')
stock_codes = []
for link in soup.find_all('a'):
href = link.get('href')
if 'shtml' in href:
code = href.split('/')[-1].split('.')[0]
stock_codes.append(code)
return stock_codes
print(get_stock_codes())
这个代码通过访问东方财富网的股票列表页面,解析HTML内容并提取股票代码。
4. 优化爬虫
为了提高爬虫的效率和稳定性,可以添加错误处理和请求间隔:
import time
import random
def get_stock_codes():
url = 'http://quote.eastmoney.com/stocklist.html'
response = requests.get(url)
response.encoding = 'gb2312'
soup = BeautifulSoup(response.text, 'html.parser')
stock_codes = []
for link in soup.find_all('a'):
href = link.get('href')
if 'shtml' in href:
code = href.split('/')[-1].split('.')[0]
stock_codes.append(code)
time.sleep(random.uniform(0.1, 0.3)) # 随机间隔,防止被封IP
return stock_codes
print(get_stock_codes())
二、利用API接口
1. 基本概念
API(应用程序编程接口)是软件之间进行通信的接口。东方财富网提供了一些公开的API,可以用于获取股票代码及其他相关数据。
2. 安装相关库
我们将使用requests库来发送HTTP请求。可以通过pip安装:
pip install requests
3. 获取API接口
东方财富网的API接口并没有公开的文档,但可以通过分析网页请求找到相关的API。例如,股票代码列表的API接口通常可以在股票筛选页面的网络请求中找到。
4. 编写获取股票代码的代码
以下是一个示例代码,通过API接口获取股票代码:
import requests
def get_stock_codes():
url = 'http://push2.eastmoney.com/api/qt/clist/get'
params = {
'pn': '1', # 页码
'pz': '5000', # 每页条数
'po': '1', # 排序
'np': '1', # 是否需要总页数
'ut': 'b2884a393a59ad64002292a3e90d46a5',
'fltt': '2',
'invt': '2',
'fid': 'f3',
'fs': 'm:0+t:6,m:0+t:13,m:1+t:2,m:1+t:23', # 股票类型
'fields': 'f12,f14' # 返回的字段,f12是股票代码,f14是股票名称
}
response = requests.get(url, params=params)
data = response.json()
stock_codes = []
for item in data['data']['diff']:
stock_codes.append(item['f12'])
return stock_codes
print(get_stock_codes())
这个代码通过东方财富网的API接口获取股票代码,并返回一个列表。
5. 优化API请求
为了提高稳定性,可以添加错误处理和请求重试机制:
import requests
import time
def get_stock_codes():
url = 'http://push2.eastmoney.com/api/qt/clist/get'
params = {
'pn': '1',
'pz': '5000',
'po': '1',
'np': '1',
'ut': 'b2884a393a59ad64002292a3e90d46a5',
'fltt': '2',
'invt': '2',
'fid': 'f3',
'fs': 'm:0+t:6,m:0+t:13,m:1+t:2,m:1+t:23',
'fields': 'f12,f14'
}
retries = 3
for _ in range(retries):
try:
response = requests.get(url, params=params)
response.raise_for_status()
data = response.json()
stock_codes = [item['f12'] for item in data['data']['diff']]
return stock_codes
except requests.exceptions.RequestException as e:
print(f"请求失败: {e}")
time.sleep(2) # 等待2秒后重试
return []
print(get_stock_codes())
三、东方财富网数据导出功能
1. 基本概念
东方财富网提供了数据导出功能,可以将股票代码等数据导出为Excel或CSV文件。这种方法适合非编程用户,或者作为编程方法的补充。
2. 操作步骤
- 打开东方财富网,进入股票筛选页面。
- 根据筛选条件选择所需的股票。
- 点击页面上的“导出”按钮,选择导出为Excel或CSV文件。
- 下载并保存文件。
3. 编写读取文件的代码
如果你导出了CSV文件,可以使用Pandas库来读取和处理数据:
import pandas as pd
def read_stock_codes(file_path):
df = pd.read_csv(file_path)
stock_codes = df['股票代码'].tolist()
return stock_codes
file_path = 'path/to/your/file.csv'
print(read_stock_codes(file_path))
这个代码将读取导出的CSV文件,并提取股票代码。
四、总结
通过上述方法,你可以轻松获取东方财富网的股票代码。使用网页爬虫适合需要灵活定制的用户,利用API接口是最为便捷和高效的方法,而东方财富网数据导出功能则适合非编程用户。希望这篇文章能帮助你更好地获取和处理东方财富网的股票数据。
相关问答FAQs:
如何使用Python爬取东方财富网的股票代码?
要使用Python爬取东方财富网的股票代码,首先需要利用requests库获取网页内容,然后使用BeautifulSoup等库解析HTML,提取出所需的股票代码。确保遵循网站的爬虫协议,并注意频率控制,以避免被封禁。
东方财富网提供哪些股票信息?
东方财富网不仅提供股票代码,还有丰富的股票市场数据,包括实时股价、历史走势、公司基本面分析、财务报表等。用户可以通过不同的接口获取多种信息,方便进行投资决策。
获取股票代码后,如何在Python中进行分析?
获取股票代码后,可以使用pandas等数据处理库将数据存储在数据框中,进行数据清洗和分析。利用matplotlib或seaborn等可视化库,可以生成图表,帮助用户直观了解市场趋势和个股表现。
爬取股票代码时需要注意哪些法律法规?
在爬取股票代码时,需要遵循相关法律法规,确保不违反网站的使用条款。避免频繁请求导致对方服务器负担过重,建议采用合理的间隔时间,保护自己的IP不被封锁。
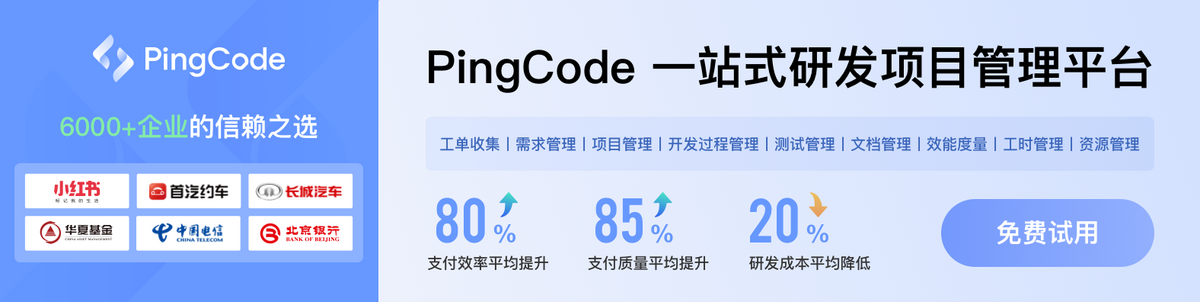