Python正则表达式如何提取字符
Python正则表达式(Regular Expression,简称regex)是一种强大的工具,用于模式匹配、查找和替换文本。提取特定字符、检查字符串是否符合特定模式、替换字符串中的特定部分是正则表达式在Python中的主要应用。本文将详细讲解Python正则表达式的使用方法,包括基础语法、常用函数以及在实际应用中的案例。
一、正则表达式的基础语法
正则表达式的基础语法是理解其功能的第一步。以下是一些常见的正则表达式符号和其含义:
.
:匹配任意单个字符(除换行符)。^
:匹配字符串的开始位置。- `Python正则表达式如何提取字符
Python正则表达式(Regular Expression,简称regex)是一种强大的工具,用于模式匹配、查找和替换文本。提取特定字符、检查字符串是否符合特定模式、替换字符串中的特定部分是正则表达式在Python中的主要应用。本文将详细讲解Python正则表达式的使用方法,包括基础语法、常用函数以及在实际应用中的案例。
一、正则表达式的基础语法
正则表达式的基础语法是理解其功能的第一步。以下是一些常见的正则表达式符号和其含义:
.
:匹配任意单个字符(除换行符)。^
:匹配字符串的开始位置。- :匹配字符串的结束位置。
*
:匹配前一个字符0次或多次。+
:匹配前一个字符1次或多次。?
:匹配前一个字符0次或1次。{n}
:匹配前一个字符恰好n次。{n,}
:匹配前一个字符至少n次。{n,m}
:匹配前一个字符n到m次。[]
:匹配括号内的任意一个字符。:转义字符,用于匹配一些特殊字符(如
.
、*
等)。
二、Python中的正则表达式模块
Python中的re
模块提供了对正则表达式的支持。以下是一些常用的函数:
1、re.match()
re.match()
函数用于检测字符串是否符合正则表达式。它从字符串的起始位置匹配,如果起始位置不符合正则表达式,则返回None
。
import re
pattern = r'hello'
text = 'hello world'
match = re.match(pattern, text)
if match:
print("Match found:", match.group())
else:
print("No match found")
2、re.search()
re.search()
函数用于在字符串中搜索匹配正则表达式的模式。如果找到则返回一个Match
对象,否则返回None
。
import re
pattern = r'world'
text = 'hello world'
match = re.search(pattern, text)
if match:
print("Match found:", match.group())
else:
print("No match found")
3、re.findall()
re.findall()
函数用于查找字符串中所有符合正则表达式的模式,并以列表形式返回。
import re
pattern = r'\d+'
text = 'There are 123 apples and 456 oranges'
matches = re.findall(pattern, text)
print("Matches found:", matches)
4、re.sub()
re.sub()
函数用于替换字符串中符合正则表达式的模式。
import re
pattern = r'apples'
text = 'I like apples'
replacement = 'oranges'
result = re.sub(pattern, replacement, text)
print("Result:", result)
三、提取特定字符的常见案例
1、提取电子邮件地址
提取电子邮件地址是一个常见的需求。电子邮件地址通常由用户名、@
符号和域名组成。
import re
pattern = r'[a-zA-Z0-9_.+-]+@[a-zA-Z0-9-]+\.[a-zA-Z0-9-.]+'
text = 'Please contact us at support@example.com for further assistance.'
matches = re.findall(pattern, text)
print("Email addresses found:", matches)
2、提取电话号码
电话号码的格式可能有所不同,但通常由数字和可能的分隔符组成。
import re
pattern = r'\+?\d[\d -]{8,12}\d'
text = 'You can reach us at +1 123-456-7890 or 098-765-4321.'
matches = re.findall(pattern, text)
print("Phone numbers found:", matches)
3、提取URL
URL通常由协议、域名和路径组成。
import re
pattern = r'https?://(?:[-\w.]|(?:%[\da-fA-F]{2}))+'
text = 'Visit our website at https://www.example.com or follow us at http://blog.example.com.'
matches = re.findall(pattern, text)
print("URLs found:", matches)
四、深入理解正则表达式的高级用法
1、使用捕获组
捕获组用于提取匹配的一部分,并且可以通过编号或名称引用。
import re
pattern = r'(\d{3})-(\d{2})-(\d{4})'
text = 'My social security number is 123-45-6789.'
match = re.search(pattern, text)
if match:
print("Full match:", match.group(0))
print("Area number:", match.group(1))
print("Group number:", match.group(2))
print("Serial number:", match.group(3))
2、使用非捕获组
非捕获组用于匹配但不捕获文本,主要用于优化性能。
import re
pattern = r'(?:\d{3})-(?:\d{2})-(?:\d{4})'
text = 'My social security number is 123-45-6789.'
match = re.search(pattern, text)
if match:
print("Full match:", match.group(0))
3、使用前瞻和后顾断言
前瞻和后顾断言用于匹配前面或后面的文本,但不包括在匹配结果中。
import re
pattern = r'(?<=\$)\d+'
text = 'The price is $100.'
match = re.search(pattern, text)
if match:
print("Price:", match.group(0))
五、正则表达式的性能优化
1、尽量避免回溯
复杂的正则表达式可能会导致大量的回溯,降低性能。使用非贪婪匹配(*?
、+?
等)可以减少回溯。
import re
pattern = r'a.*?b'
text = 'a' * 1000 + 'b'
match = re.search(pattern, text)
if match:
print("Match found:", match.group(0))
2、使用预编译的正则表达式
对于频繁使用的正则表达式,可以使用re.compile()
函数进行预编译,提高匹配速度。
import re
pattern = re.compile(r'\d+')
text = '123 abc 456 def'
matches = pattern.findall(text)
print("Matches found:", matches)
六、实战案例:从网页提取信息
1、提取网页中的所有链接
在网页爬虫中,提取所有链接是一个常见的需求。
import re
import requests
url = 'https://www.example.com'
response = requests.get(url)
html = response.text
pattern = r'href="(https?://[^"]+)"'
links = re.findall(pattern, html)
print("Links found:", links)
2、提取网页中的所有图片链接
类似地,提取网页中的所有图片链接也是一个常见需求。
import re
import requests
url = 'https://www.example.com'
response = requests.get(url)
html = response.text
pattern = r'src="(https?://[^"]+\.jpg)"'
images = re.findall(pattern, html)
print("Image URLs found:", images)
七、总结
Python正则表达式是一种强大的文本处理工具,能够高效地完成文本匹配、提取和替换任务。本文详细介绍了正则表达式的基础语法、常用函数以及在实际应用中的案例。通过掌握这些知识,您将能够在实际项目中灵活运用正则表达式,提高文本处理的效率和准确性。
相关问答FAQs:
如何使用Python正则表达式提取特定格式的字符?
Python的正则表达式模块re
可以帮助你提取符合特定模式的字符。首先,你需要导入re
模块,然后使用re.findall()
函数来查找所有符合模式的字符。例如,如果你想提取所有以字母开头的单词,可以使用模式r'\b[a-zA-Z]\w*'
。这样可以获得符合条件的所有单词列表。
正则表达式中的分组和捕获是如何工作的?
在Python正则表达式中,使用括号()
可以创建分组,这样可以提取特定的字符序列。例如,如果你想从字符串中提取日期格式“YYYY-MM-DD”,可以使用模式r'(\d{4})-(\d{2})-(\d{2})'
。这会将年份、月份和日期分别捕获到分组中,方便后续处理。
如何处理提取过程中可能遇到的特殊字符?
在使用正则表达式提取字符时,特殊字符如.
、*
、?
等具有特殊含义,可能会干扰提取结果。为了解决这个问题,可以使用反斜杠\
对这些特殊字符进行转义。例如,如果你需要提取包含句点的文件名,可以使用模式r'[a-zA-Z0-9]+\.[a-zA-Z]{2,4}'
,确保句点被正确处理。
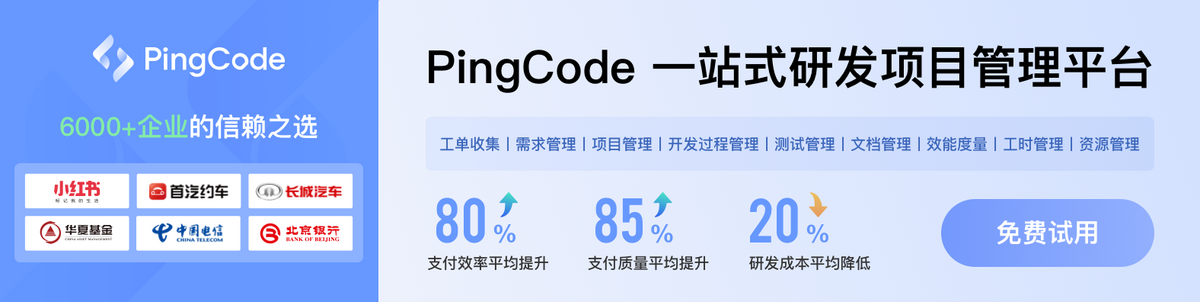