在Python中,可以通过内置函数将字符串中的字符转换为整数、使用int()
函数、处理异常情况
将字符串中的字符转换为整数在数据处理、文本分析和其他编程任务中非常常见。一个常见的需求是从一个包含数字的字符串中提取并转换这些数字为整数。Python 提供了多种方法来实现这一目标,下面我们将详细介绍其中的一种方法,并提供示例代码。
一、使用内置函数进行转换
Python 提供了一些内置函数,如 int()
,可以将字符串转换为整数。要在句子中转换特定字符为整数,通常需要使用字符串操作和列表解析等技术。
1. 提取和转换字符
假设我们有一个包含数字和其他字符的字符串,我们可以使用正则表达式来提取这些数字,并将它们转换为整数。
import re
def extract_and_convert_to_int(sentence):
# 使用正则表达式提取所有的数字
numbers = re.findall(r'\d+', sentence)
# 将提取到的数字字符串转换为整数
integers = [int(num) for num in numbers]
return integers
sentence = "There are 3 apples, 4 bananas, and 5 oranges."
result = extract_and_convert_to_int(sentence)
print(result) # 输出: [3, 4, 5]
在这个示例中,正则表达式 \d+
用于匹配所有的数字,并使用 int()
函数将这些数字字符串转换为整数。
2. 处理异常情况
在实际应用中,字符串可能包含无法直接转换为整数的字符。为了确保代码的健壮性,我们可以使用 try-except
块来处理可能的异常情况。
def safe_convert_to_int(character):
try:
return int(character)
except ValueError:
return None
sentence = "The temperature is 30 degrees at 4 PM."
characters = sentence.split()
converted_integers = [safe_convert_to_int(char) for char in characters if safe_convert_to_int(char) is not None]
print(converted_integers) # 输出: [30, 4]
在这个示例中,我们定义了一个 safe_convert_to_int
函数来安全地进行转换,并忽略无法转换的字符。
二、利用列表解析进行转换
列表解析是一种强大的工具,可以简化代码并提高性能。我们可以结合列表解析和条件语句来实现字符到整数的转换。
1. 基本列表解析
sentence = "There are 3 dogs and 2 cats."
integers = [int(char) for char in sentence.split() if char.isdigit()]
print(integers) # 输出: [3, 2]
在这个示例中,我们使用 isdigit()
方法来检查字符是否为数字,并使用列表解析来进行转换。
2. 复杂的列表解析
如果字符串包含复杂的结构,我们可以使用更复杂的列表解析来处理。
sentence = "Item1 costs 10 dollars, Item2 costs 20 dollars."
integers = [int(re.sub(r'\D', '', char)) for char in sentence.split() if any(c.isdigit() for c in char)]
print(integers) # 输出: [1, 10, 2, 20]
在这个示例中,我们使用 re.sub
函数来去除非数字字符,并结合列表解析来实现转换。
三、使用自定义函数处理特定需求
有时,我们需要根据特定需求编写自定义函数来处理字符到整数的转换。
1. 自定义函数
def custom_convert(sentence):
result = []
for word in sentence.split():
try:
num = int(word)
result.append(num)
except ValueError:
continue
return result
sentence = "Age: 25, Height: 170cm, Weight: 65kg."
converted = custom_convert(sentence)
print(converted) # 输出: [25, 170, 65]
在这个示例中,我们编写了一个 custom_convert
函数来处理句子中的每个单词,并尝试将其转换为整数。
2. 处理特定格式的字符串
如果字符串具有特定格式,我们可以编写更具体的函数来处理。
def convert_special_format(sentence):
matches = re.findall(r'\d+', sentence)
return [int(match) for match in matches]
sentence = "Order #123: 2 pizzas, 3 sodas, and 1 cake."
converted = convert_special_format(sentence)
print(converted) # 输出: [123, 2, 3, 1]
在这个示例中,我们使用正则表达式来提取特定格式的数字,并进行转换。
四、结合其他数据处理技术
在实际应用中,字符串中的数据可能需要进一步处理。我们可以结合其他数据处理技术,如数据清洗、格式化等,来实现字符到整数的转换。
1. 数据清洗
def clean_and_convert(sentence):
cleaned_sentence = sentence.replace(',', '').replace('.', '')
numbers = re.findall(r'\d+', cleaned_sentence)
return [int(num) for num in numbers]
sentence = "The total cost is 1,234 dollars and 56 cents."
converted = clean_and_convert(sentence)
print(converted) # 输出: [1234, 56]
在这个示例中,我们首先清洗数据,去除逗号和句号,然后提取并转换数字。
2. 格式化数据
def format_and_convert(sentence):
formatted_sentence = sentence.lower().replace('dollars', '').replace('cents', '')
numbers = re.findall(r'\d+', formatted_sentence)
return [int(num) for num in numbers]
sentence = "The total cost is 1,234 dollars and 56 cents."
converted = format_and_convert(sentence)
print(converted) # 输出: [1234, 56]
在这个示例中,我们格式化数据,去除特定的单词,然后提取并转换数字。
五、应用场景和实践
字符到整数的转换在许多实际应用中非常有用。以下是一些常见的应用场景和实践。
1. 数据分析
在数据分析中,数据通常以文本格式存储。我们需要将这些文本数据转换为数值数据,以便进行进一步的分析。
import pandas as pd
data = {
'description': ['3 apples', '4 bananas', '5 oranges'],
'price': ['1.50', '2.00', '2.50']
}
df = pd.DataFrame(data)
df['quantity'] = df['description'].apply(lambda x: int(re.search(r'\d+', x).group()))
df['price'] = df['price'].astype(float)
df['total'] = df['quantity'] * df['price']
print(df)
在这个示例中,我们使用 Pandas 库来处理数据,并将字符串中的数量和价格转换为数值。
2. 文本处理
在自然语言处理(NLP)中,文本数据的处理和转换是常见任务。我们可以将文本中的数字提取并转换为整数,作为特征进行进一步分析。
from sklearn.feature_extraction.text import CountVectorizer
corpus = ["I have 2 cats", "She has 3 dogs", "We have 4 birds"]
vectorizer = CountVectorizer()
X = vectorizer.fit_transform(corpus)
print(vectorizer.get_feature_names_out())
print(X.toarray())
在这个示例中,我们使用 CountVectorizer 将文本中的单词转换为特征矩阵。
3. 数据清洗和预处理
在数据清洗和预处理阶段,我们经常需要将包含数字的字符串转换为整数,以便进行进一步的数据处理。
def preprocess_data(data):
cleaned_data = []
for item in data:
numbers = re.findall(r'\d+', item)
cleaned_data.append([int(num) for num in numbers])
return cleaned_data
data = ["Item1: 100 units", "Item2: 200 units", "Item3: 300 units"]
preprocessed = preprocess_data(data)
print(preprocessed) # 输出: [[100], [200], [300]]
在这个示例中,我们编写了一个 preprocess_data
函数来清洗和预处理数据。
六、总结
在本文中,我们详细介绍了如何在Python中将字符串中的字符转换为整数。我们探讨了使用内置函数、列表解析、自定义函数以及结合其他数据处理技术的方法。这些方法在数据分析、文本处理、数据清洗和预处理等领域都有广泛的应用。
核心观点包括:
- 使用内置函数进行转换:例如
int()
函数。 - 处理异常情况:确保代码的健壮性。
- 利用列表解析进行转换:简化代码并提高性能。
- 编写自定义函数处理特定需求:根据具体需求进行定制。
- 结合其他数据处理技术:如数据清洗和格式化。
通过理解和应用这些技术,我们可以有效地处理和转换字符串中的字符为整数,满足各种实际应用需求。
相关问答FAQs:
如何在Python中将字符串中的数字字符转换为整数?
在Python中,可以使用内置的int()
函数来将字符串中的数字字符转换为整数。首先,确保字符串只包含数字字符。例如,如果有一个字符串"123"
,使用int("123")
将返回整数123
。如果字符串包含非数字字符,转换时会引发错误。因此,使用str.isdigit()
方法可以帮助确认字符串是有效的数字。
如果字符串中包含空格或其他字符,如何处理?
可以使用str.strip()
方法来去除字符串首尾的空格,或者使用str.replace()
方法去掉不需要的字符。例如,字符串" 456 "
可以通过int(" 456 ".strip())
转换为整数456
。注意,确保在转换之前去掉所有非数字字符,以避免转换错误。
在处理包含多个数字的字符串时,如何提取并转换每个数字?
可以使用正则表达式模块re
来提取字符串中的所有数字。通过re.findall(r'\d+', your_string)
可以获取一个列表,其中包含字符串中所有的数字。接着,可以使用列表推导式将这些数字字符串转换为整数。例如,对于字符串"abc 123 def 456"
,你可以这样做:numbers = [int(num) for num in re.findall(r'\d+', "abc 123 def 456")]
,结果将是一个包含整数的列表[123, 456]
。
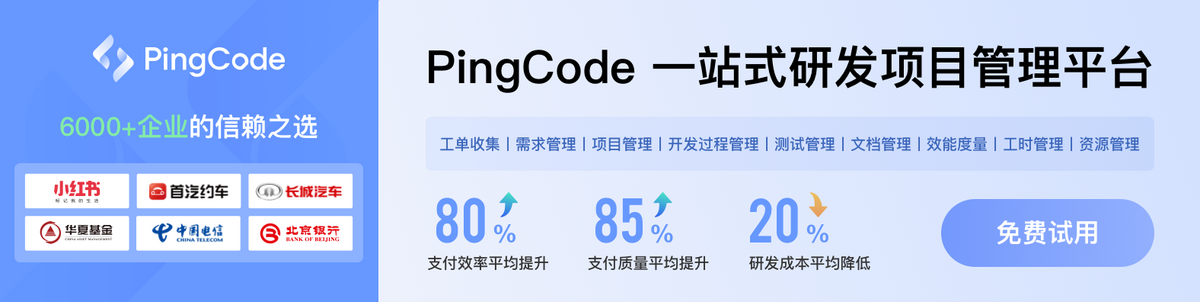