Python去掉TXT中的行数据库的几种方法有:使用内置函数、正则表达式、第三方库、逐行读取再写入。 其中,最常用和最简单的方法是使用内置函数。这种方法不仅代码简洁,而且执行效率较高。本文将详细介绍如何使用Python实现去掉TXT文件中的行数据库,并提供实际操作的代码示例。
一、使用Python内置函数
Python内置的文件操作函数十分强大且易用。通过使用open()
、readlines()
和writelines()
,我们可以轻松地读取和写入文件。
1.1、读取文件并过滤行
首先,我们需要读取文件内容,并过滤掉不需要的行。以下是一个示例代码:
def remove_lines(input_file, output_file, lines_to_remove):
with open(input_file, 'r') as file:
lines = file.readlines()
with open(output_file, 'w') as file:
for number, line in enumerate(lines):
if number not in lines_to_remove:
file.write(line)
input_file = 'input.txt'
output_file = 'output.txt'
lines_to_remove = [1, 3, 5] # 需要去掉的行号(从0开始)
remove_lines(input_file, output_file, lines_to_remove)
上面的代码展示了如何从input.txt
文件中去掉指定行,并将结果写入到output.txt
文件中。
1.2、逐行读取和写入
这种方法适用于大文件,因为它不会一次性将整个文件读入内存。示例如下:
def remove_lines(input_file, output_file, lines_to_remove):
with open(input_file, 'r') as infile, open(output_file, 'w') as outfile:
for lineno, line in enumerate(infile):
if lineno not in lines_to_remove:
outfile.write(line)
input_file = 'input.txt'
output_file = 'output.txt'
lines_to_remove = [1, 3, 5]
remove_lines(input_file, output_file, lines_to_remove)
这段代码的工作原理与前面的例子类似,但它逐行读取和写入文件,更加适合处理大文件。
二、使用正则表达式
正则表达式是处理文本数据的强大工具。在Python中,可以使用re
模块来完成这一任务。
2.1、删除特定模式的行
假设我们需要删除包含特定模式的行,如包含某个单词或字符的行。以下是示例代码:
import re
def remove_lines_with_pattern(input_file, output_file, pattern):
with open(input_file, 'r') as infile, open(output_file, 'w') as outfile:
for line in infile:
if not re.search(pattern, line):
outfile.write(line)
input_file = 'input.txt'
output_file = 'output.txt'
pattern = r'pattern_to_remove' # 需要去掉的模式
remove_lines_with_pattern(input_file, output_file, pattern)
这段代码中,re.search()
用于查找行中是否包含指定的模式,如果不包含,则将该行写入输出文件。
三、使用第三方库
Python有许多第三方库可以简化文件操作,例如pandas
库。pandas
库非常适合处理结构化的数据,例如CSV或Excel文件,但它同样可以处理TXT文件。
3.1、使用Pandas读取和过滤行
以下是一个示例,演示如何使用pandas
库来删除指定的行:
import pandas as pd
def remove_lines_with_pandas(input_file, output_file, lines_to_remove):
df = pd.read_csv(input_file, header=None) # 读取TXT文件
df = df.drop(lines_to_remove) # 删除指定的行
df.to_csv(output_file, index=False, header=False) # 写入新的TXT文件
input_file = 'input.txt'
output_file = 'output.txt'
lines_to_remove = [1, 3, 5]
remove_lines_with_pandas(input_file, output_file, lines_to_remove)
在这个例子中,pandas.read_csv()
用于读取TXT文件,df.drop()
用于删除指定的行,最后将结果写入新的TXT文件。
四、逐行读取再写入
这种方法适用于需要逐行处理的情况,如需要对每一行进行复杂的处理或判断。
4.1、逐行处理并写入新文件
以下是一个示例代码:
def remove_lines_with_custom_logic(input_file, output_file, custom_logic):
with open(input_file, 'r') as infile, open(output_file, 'w') as outfile:
for line in infile:
if custom_logic(line):
outfile.write(line)
def custom_logic(line):
# 定义你的自定义逻辑,例如去掉包含特定单词的行
return 'specific_word' not in line
input_file = 'input.txt'
output_file = 'output.txt'
remove_lines_with_custom_logic(input_file, output_file, custom_logic)
在这个例子中,custom_logic()
函数可以包含任何自定义的行处理逻辑。remove_lines_with_custom_logic()
函数逐行读取输入文件,并根据自定义逻辑决定是否将行写入输出文件。
总结
Python提供了多种方法来去掉TXT文件中的行数据库,如使用内置函数、正则表达式、第三方库和逐行读取再写入。 每种方法都有其适用的场景和优缺点。对于简单的行删除操作,使用内置函数是最简单和高效的选择;对于复杂的文本处理任务,正则表达式和第三方库如pandas
可以提供强大的支持;而对于需要逐行处理的情况,逐行读取和写入的方法则更加灵活和适用。通过本文的介绍,相信你已经掌握了多种实现方法,可以根据具体需求选择最适合的方法进行操作。
相关问答FAQs:
如何在Python中删除txt文件中的特定行?
在Python中,删除txt文件中的特定行可以通过读取文件内容并将不需要的行过滤掉来实现。您可以使用with open()
语句打开文件,读取每一行,然后使用列表推导式创建一个新的列表,包含所有不需要删除的行。最后,将新内容写回到文件中。
使用Python处理大文件时,有什么技巧可以提高效率?
当处理大型txt文件时,可以逐行读取文件而不是一次性加载整个文件到内存中。使用file.readline()
或for line in file
的方式可以有效减少内存使用。同时,使用fileinput
模块来直接在文件上进行行的过滤和处理也是一个不错的选择,这样能够避免生成临时列表,从而提高效率。
如何确保在删除txt文件的行时不会丢失重要数据?
在对txt文件进行行删除操作之前,最好先备份原始文件。您可以将文件复制到另一个位置,或者在处理文件时使用临时文件。这样,即使在处理过程中出现错误,您也可以轻松恢复数据。此外,在进行行删除操作时,建议先在小文件上进行测试,以确保逻辑正确,避免误删重要信息。
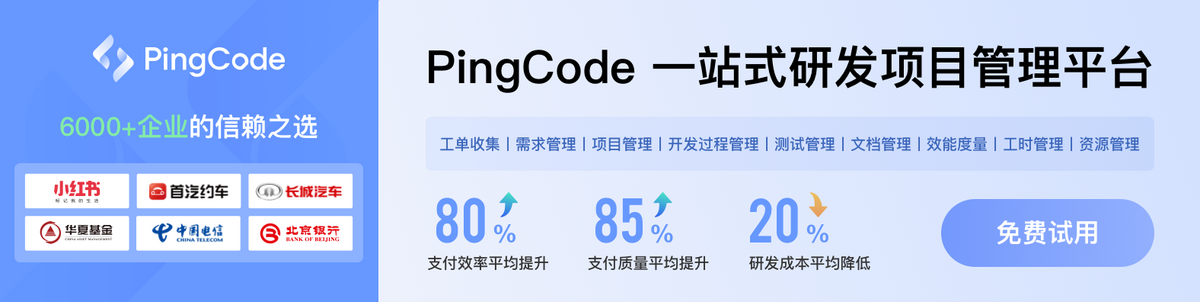