Python 不用第三方库的方法包括:基本数据类型与内置函数、文件操作、数据结构、面向对象编程、错误处理、并发编程。 其中,基本数据类型与内置函数 是最基础和最常用的内容,它们构成了Python编程的核心。Python内置了多种数据类型和函数,可以在不依赖任何外部库的情况下进行各种操作。了解这些基本数据类型和内置函数是掌握Python编程的第一步。
Python是一门功能强大的编程语言,其内置了丰富的功能和数据结构,能够在不依赖第三方库的情况下完成许多任务。接下来我们将详细探讨如何在不使用第三方库的前提下,利用Python进行各种编程任务。
一、基本数据类型与内置函数
Python内置了多种数据类型和函数,能够满足各种编程需求。了解和熟练使用这些基本数据类型和内置函数是Python编程的基础。
1. 基本数据类型
数字(Number)
Python支持多种数字类型,包括整数(int)、浮点数(float)和复数(complex)。这些数据类型支持多种操作,如加减乘除、取余、幂运算等。
# 整数
a = 10
b = 5
浮点数
c = 3.14
复数
d = 1 + 2j
基本运算
print(a + b) # 加法
print(a - b) # 减法
print(a * b) # 乘法
print(a / b) # 除法
print(a % b) # 取余
print(a b) # 幂运算
print(d.real) # 复数的实部
print(d.imag) # 复数的虚部
字符串(String)
字符串是不可变的数据类型,可以用单引号、双引号或三引号创建。Python提供了丰富的字符串操作函数,如拼接、分割、查找、替换等。
# 字符串
s1 = 'Hello'
s2 = "World"
字符串拼接
s3 = s1 + ' ' + s2
print(s3)
字符串分割
words = s3.split()
print(words)
查找子字符串
print(s3.find('World'))
替换子字符串
s4 = s3.replace('World', 'Python')
print(s4)
列表(List)
列表是Python中最常用的数据结构之一,可以用来存储任意类型的元素,并且支持多种操作,如添加、删除、排序等。
# 列表
lst = [1, 2, 3, 4, 5]
添加元素
lst.append(6)
print(lst)
删除元素
lst.remove(3)
print(lst)
列表排序
lst.sort(reverse=True)
print(lst)
列表切片
print(lst[1:4])
2. 内置函数
Python提供了许多内置函数,可以直接调用,无需导入任何模块。这些函数涵盖了数学运算、序列操作、类型转换等多方面的功能。
数学运算
# 绝对值
print(abs(-5))
最大值和最小值
print(max(1, 2, 3, 4, 5))
print(min(1, 2, 3, 4, 5))
幂运算
print(pow(2, 3))
四舍五入
print(round(3.14159, 2))
序列操作
# 长度
print(len([1, 2, 3, 4, 5]))
枚举
for index, value in enumerate(['a', 'b', 'c']):
print(index, value)
过滤
print(list(filter(lambda x: x % 2 == 0, [1, 2, 3, 4, 5])))
映射
print(list(map(lambda x: x * 2, [1, 2, 3, 4, 5])))
类型转换
# 转换为整数
print(int('123'))
转换为浮点数
print(float('3.14'))
转换为字符串
print(str(123))
转换为列表
print(list('hello'))
二、文件操作
Python内置了丰富的文件操作函数,可以方便地进行文件的读写操作。
1. 文件读操作
# 打开文件
with open('example.txt', 'r') as file:
# 读取全部内容
content = file.read()
print(content)
# 逐行读取
file.seek(0)
for line in file:
print(line.strip())
2. 文件写操作
# 打开文件
with open('example.txt', 'w') as file:
# 写入内容
file.write('Hello, World!\n')
file.write('Python is awesome!\n')
追加写入
with open('example.txt', 'a') as file:
file.write('Append this line.\n')
三、数据结构
Python内置了多种数据结构,包括列表、元组、集合和字典,能够满足不同的编程需求。
1. 元组(Tuple)
元组是不可变的序列,可以用来存储多个元素。元组的操作与列表类似,但不能修改元素。
# 元组
t = (1, 2, 3, 4, 5)
访问元素
print(t[0])
元组切片
print(t[1:4])
元组解包
a, b, c, d, e = t
print(a, b, c, d, e)
2. 集合(Set)
集合是无序且不重复的元素集合,可以用来进行集合运算,如交集、并集、差集等。
# 集合
s1 = {1, 2, 3, 4, 5}
s2 = {4, 5, 6, 7, 8}
集合运算
print(s1 & s2) # 交集
print(s1 | s2) # 并集
print(s1 - s2) # 差集
3. 字典(Dictionary)
字典是键值对的集合,可以用来存储和快速查找数据。
# 字典
d = {'a': 1, 'b': 2, 'c': 3}
访问元素
print(d['a'])
添加和修改元素
d['d'] = 4
d['a'] = 10
print(d)
删除元素
del d['b']
print(d)
遍历字典
for key, value in d.items():
print(key, value)
四、面向对象编程
Python支持面向对象编程,可以定义类和创建对象。
1. 定义类
class Person:
def __init__(self, name, age):
self.name = name
self.age = age
def greet(self):
print(f'Hello, my name is {self.name} and I am {self.age} years old.')
创建对象
p = Person('Alice', 30)
p.greet()
2. 继承与多态
class Animal:
def make_sound(self):
print('Some generic sound')
class Dog(Animal):
def make_sound(self):
print('Woof! Woof!')
class Cat(Animal):
def make_sound(self):
print('Meow! Meow!')
多态
animals = [Dog(), Cat(), Animal()]
for animal in animals:
animal.make_sound()
五、错误处理
Python提供了异常处理机制,可以捕获和处理程序运行时的错误。
1. 捕获异常
try:
result = 10 / 0
except ZeroDivisionError as e:
print(f'Error: {e}')
2. 自定义异常
class CustomError(Exception):
pass
try:
raise CustomError('This is a custom error')
except CustomError as e:
print(f'Error: {e}')
六、并发编程
Python支持多线程和多进程编程,可以提高程序的执行效率。
1. 多线程
import threading
def print_numbers():
for i in range(5):
print(i)
创建线程
thread = threading.Thread(target=print_numbers)
thread.start()
thread.join()
2. 多进程
import multiprocessing
def print_numbers():
for i in range(5):
print(i)
创建进程
process = multiprocessing.Process(target=print_numbers)
process.start()
process.join()
通过以上内容,我们可以看到,Python内置了丰富的功能和数据结构,能够在不依赖第三方库的情况下完成许多编程任务。掌握这些基本数据类型与内置函数、文件操作、数据结构、面向对象编程、错误处理和并发编程的知识,可以帮助我们更好地利用Python进行编程。
相关问答FAQs:
1. 如何在Python中实现基本的文件操作而无需第三方库?
在Python中,可以使用内置的open()
函数进行文件操作。通过指定文件路径和模式(如读取、写入),可以轻松地打开、读取、写入和关闭文件。例如,使用with open('example.txt', 'r') as file:
可以在上下文管理器中安全地读取文件内容。这样可以确保文件在操作完成后被正确关闭,避免资源泄露。
2. 在Python中实现网络请求而无需使用第三方库,是否可行?
可以使用Python内置的http.client
模块来进行基本的网络请求。通过建立HTTP连接并使用request
方法,可以获取远程服务器的数据。例如,使用http.client.HTTPConnection('www.example.com')
可以创建连接,然后通过conn.request('GET', '/')
发送请求,最终通过conn.getresponse()
获取响应。
3. 如何在不依赖第三方库的情况下处理JSON数据?
Python的内置json
模块提供了处理JSON数据的功能。通过json.loads()
可以将JSON字符串转换为Python字典,使用json.dumps()
则可以将Python字典序列化为JSON字符串。这两个方法使得在处理API返回的数据或存储配置时,可以方便地进行数据格式转换,完全不需要额外的库。
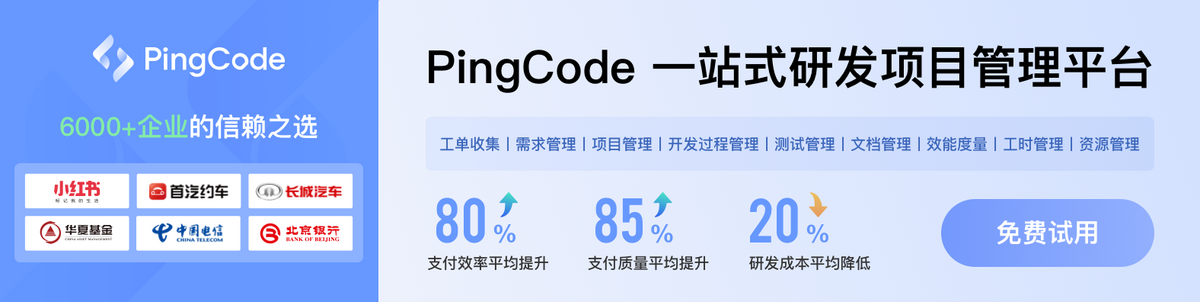