要在Python中一行一行地读取数据,可以使用readline()
、readlines()
和迭代器的方法。其中,使用迭代器是最常见也是推荐的方式,因为它不仅简单而且高效。下面是对其中一种方法的详细描述。使用迭代器来逐行读取文件内容,是因为它避免了将整个文件一次性加载到内存中,适用于大文件处理。
一、使用迭代器逐行读取
Python提供了非常简便的方式来逐行读取文件,那就是使用文件对象本身作为迭代器。通过这种方法,我们可以逐行读取文件内容,避免了内存占用过高的问题。以下是一个简单的示例代码:
with open('example.txt', 'r') as file:
for line in file:
print(line.strip())
解释:
open
函数:用于打开文件,'r'
表示以只读方式打开文件。with
语句:确保文件在使用完毕后被正确地关闭。for line in file
:通过迭代器一行一行读取文件内容。line.strip()
:去掉每行末尾的换行符。
接下来,我们将详细探讨Python中逐行读取数据的不同方法和它们的应用场景。
二、readline()
方法
readline()
方法每次调用会读取文件中的一行内容,并在末尾添加换行符(如果有)。这种方法适用于需要逐行处理文件内容的场景。
with open('example.txt', 'r') as file:
while True:
line = file.readline()
if not line:
break
print(line.strip())
解释:
file.readline()
:每次读取一行,如果读取到文件末尾,会返回空字符串。if not line
:判断是否已到文件末尾。
三、readlines()
方法
readlines()
方法会将文件中的所有行一次性读取并存储在一个列表中,每行作为列表中的一个元素。这种方法适用于文件内容较小的场景。
with open('example.txt', 'r') as file:
lines = file.readlines()
for line in lines:
print(line.strip())
解释:
file.readlines()
:将文件内容全部读取并存储到一个列表中。for line in lines
:遍历列表,逐行处理内容。
四、逐行处理大文件
在处理大文件时,使用迭代器的方法是最为有效的,因为它不会一次性将整个文件加载到内存中,从而节省内存资源。以下是一个实际应用的示例:
def process_large_file(file_path):
with open(file_path, 'r') as file:
for line in file:
# 假设需要对每行进行某种处理
process_line(line)
def process_line(line):
# 在这里对每行进行处理
print(line.strip())
process_large_file('large_example.txt')
五、逐行读取并写入新文件
有时我们需要在读取文件的同时,将处理后的内容写入到一个新文件中。这种需求在数据清洗和转换过程中非常常见。
with open('input.txt', 'r') as infile, open('output.txt', 'w') as outfile:
for line in infile:
processed_line = process_line(line)
outfile.write(processed_line + '\n')
def process_line(line):
# 对每行进行处理
return line.strip().upper()
解释:
- 同时打开两个文件:一个用于读取,一个用于写入。
process_line(line)
:对每行内容进行处理,并返回处理后的结果。
六、逐行读取并计数
在一些数据分析任务中,我们可能需要统计文件中的行数,或者统计包含某些特定模式的行数。以下是一个示例代码:
def count_lines(file_path):
line_count = 0
with open(file_path, 'r') as file:
for line in file:
line_count += 1
return line_count
def count_pattern_lines(file_path, pattern):
pattern_count = 0
with open(file_path, 'r') as file:
for line in file:
if pattern in line:
pattern_count += 1
return pattern_count
line_count = count_lines('example.txt')
pattern_count = count_pattern_lines('example.txt', 'specific_pattern')
print(f"Total lines: {line_count}")
print(f"Lines containing 'specific_pattern': {pattern_count}")
解释:
count_lines
函数:统计文件中的总行数。count_pattern_lines
函数:统计包含特定模式的行数。
七、逐行读取并提取特定信息
在数据处理和分析中,我们通常需要从文件中提取特定的信息。以下示例展示了如何逐行读取文件并提取特定列的数据:
def extract_column(file_path, column_index):
column_data = []
with open(file_path, 'r') as file:
for line in file:
columns = line.strip().split(',')
if len(columns) > column_index:
column_data.append(columns[column_index])
return column_data
column_data = extract_column('data.csv', 2)
print(column_data)
解释:
extract_column
函数:提取CSV文件中某一列的数据。line.strip().split(',')
:将每行按逗号分隔为多个列。
八、逐行读取并进行多线程处理
在处理大文件时,利用多线程可以加速数据处理。以下示例展示了如何使用Python的concurrent.futures
模块进行多线程处理:
import concurrent.futures
def process_large_file_multithread(file_path):
with open(file_path, 'r') as file:
lines = file.readlines()
with concurrent.futures.ThreadPoolExecutor(max_workers=5) as executor:
executor.map(process_line_multithread, lines)
def process_line_multithread(line):
# 在这里对每行进行多线程处理
print(line.strip().upper())
process_large_file_multithread('large_example.txt')
解释:
concurrent.futures.ThreadPoolExecutor
:创建一个线程池。executor.map
:将每行数据分配给线程池中的线程进行处理。
九、逐行读取并处理JSON数据
在实际应用中,文件中存储的数据可能是JSON格式的。以下示例展示了如何逐行读取文件并解析JSON数据:
import json
def process_json_file(file_path):
with open(file_path, 'r') as file:
for line in file:
data = json.loads(line)
process_json_data(data)
def process_json_data(data):
# 在这里对JSON数据进行处理
print(data)
process_json_file('data.json')
解释:
json.loads(line)
:将每行内容解析为JSON对象。process_json_data(data)
:对解析后的JSON数据进行处理。
十、逐行读取并处理日志文件
日志文件通常是以文本形式存储的,逐行读取日志文件是分析和监控系统运行状态的重要手段。以下示例展示了如何逐行读取日志文件并提取特定信息:
import re
def process_log_file(file_path, pattern):
with open(file_path, 'r') as file:
for line in file:
if re.search(pattern, line):
process_log_line(line)
def process_log_line(line):
# 在这里对匹配到的日志行进行处理
print(line.strip())
process_log_file('server.log', r'ERROR')
解释:
re.search(pattern, line)
:使用正则表达式匹配日志行。process_log_line(line)
:对匹配到的日志行进行处理。
总结
在Python中逐行读取数据的方法多种多样,每种方法都有其适用的场景。使用迭代器逐行读取文件内容是最常用且推荐的方法,因为它简单高效,适用于大文件处理。其他方法如readline()
、readlines()
、逐行处理大文件、逐行读取并写入新文件、逐行读取并计数、逐行读取并提取特定信息、逐行读取并进行多线程处理、逐行读取并处理JSON数据、逐行读取并处理日志文件等,都是在不同场景下的有效手段。
通过掌握这些方法,我们可以灵活地处理不同类型和规模的文件,满足各种数据处理和分析需求。希望这篇文章能够帮助你更好地理解和应用Python中的逐行读取数据技巧。
相关问答FAQs:
如何在Python中逐行读取文本文件?
在Python中,逐行读取文本文件可以使用内置的open()
函数结合for
循环。打开文件后,可以直接遍历文件对象,这样每次循环都会读取一行内容。示例代码如下:
with open('file.txt', 'r') as file:
for line in file:
print(line.strip()) # 使用strip()去除行末的换行符
使用readline()
方法逐行读取的优缺点是什么?readline()
方法允许你逐行读取文件,每次调用该方法会返回下一行内容。优点在于可以精确控制读取的行数,但缺点是需要手动管理文件的读取状态,可能会导致代码复杂化。示例代码如下:
with open('file.txt', 'r') as file:
while True:
line = file.readline()
if not line:
break
print(line.strip())
在大文件中逐行读取数据时应注意什么?
处理大文件时,逐行读取是一个节省内存的好方法。使用with
语句打开文件可以确保文件在操作后自动关闭。此外,使用生成器表达式也可以减少内存占用,例如:
with open('large_file.txt') as file:
for line in (line.strip() for line in file):
print(line)
这种方法有效地处理大文件,避免一次性将整个文件加载到内存中。
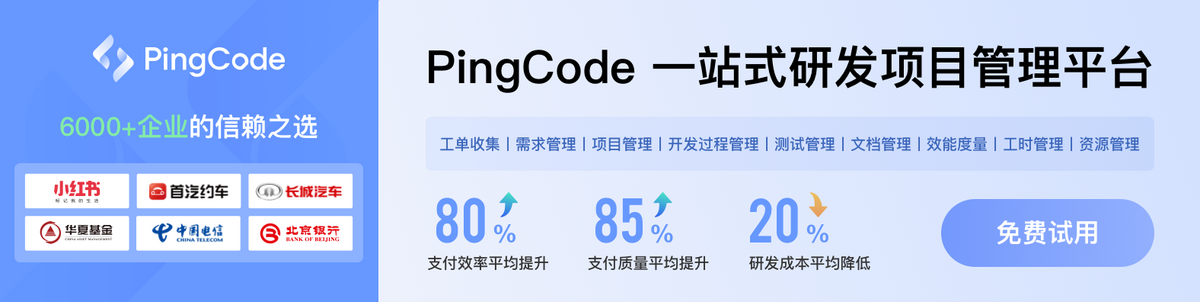