Python 3.6 如何使用 urllib 下载
在Python 3.6中,urllib2库已被分解为几个模块,主要包括urllib.request
和urllib.error
,你需要使用urllib.request
模块来实现下载功能。具体操作包括导入模块、构建请求、处理响应等步骤。下面将详细介绍如何使用这些模块进行文件下载。
一、urllib库概述
在Python 3.6中,urllib
库分为了几个不同的子模块:urllib.request
用于打开和读取URLs,urllib.error
用于处理错误,urllib.parse
用于解析URLs,urllib.robotparser
用于解析robots.txt文件。对于下载操作,我们主要关注urllib.request
。
二、导入模块并构建请求
首先,你需要导入urllib.request
模块,这个模块提供了许多类和函数来处理URL。接着,你可以使用urllib.request.urlopen()
函数来发送HTTP请求,并获取响应。
import urllib.request
url = 'http://example.com/somefile.txt'
response = urllib.request.urlopen(url)
三、读取响应并保存文件
在获取响应对象后,可以使用read()
方法来读取文件内容,并将其保存到本地文件系统中。
import urllib.request
url = 'http://example.com/somefile.txt'
response = urllib.request.urlopen(url)
读取内容并写入文件
with open('downloaded_file.txt', 'wb') as out_file:
out_file.write(response.read())
四、处理错误
在网络请求过程中,可能会遇到各种错误,例如404错误、500错误等。为了使程序更加健壮,可以使用try-except
块来捕获和处理这些错误。
import urllib.request
import urllib.error
url = 'http://example.com/somefile.txt'
try:
response = urllib.request.urlopen(url)
with open('downloaded_file.txt', 'wb') as out_file:
out_file.write(response.read())
except urllib.error.HTTPError as e:
print(f'HTTP error: {e.code}')
except urllib.error.URLError as e:
print(f'URL error: {e.reason}')
五、其他高级用法
除了基本的下载功能,urllib.request
模块还支持许多高级用法,例如设置请求头、使用代理、处理重定向等。
- 设置请求头
有时候你可能需要设置一些请求头,例如User-Agent、Referer等。可以使用urllib.request.Request
类来实现。
import urllib.request
url = 'http://example.com/somefile.txt'
headers = {'User-Agent': 'Mozilla/5.0'}
request = urllib.request.Request(url, headers=headers)
response = urllib.request.urlopen(request)
with open('downloaded_file.txt', 'wb') as out_file:
out_file.write(response.read())
- 使用代理
如果你需要通过代理服务器来访问目标URL,可以使用ProxyHandler
来设置代理。
import urllib.request
url = 'http://example.com/somefile.txt'
proxy = 'http://proxy.example.com:8080'
proxy_handler = urllib.request.ProxyHandler({'http': proxy, 'https': proxy})
opener = urllib.request.build_opener(proxy_handler)
urllib.request.install_opener(opener)
response = urllib.request.urlopen(url)
with open('downloaded_file.txt', 'wb') as out_file:
out_file.write(response.read())
- 处理重定向
默认情况下,urllib.request
会自动处理HTTP重定向。如果你需要获取重定向后的最终URL,可以使用geturl()
方法。
import urllib.request
url = 'http://example.com/somefile.txt'
response = urllib.request.urlopen(url)
final_url = response.geturl()
print(f'Final URL: {final_url}')
with open('downloaded_file.txt', 'wb') as out_file:
out_file.write(response.read())
六、总结
通过以上内容,我们详细介绍了在Python 3.6中如何使用urllib
库来实现文件下载,包括导入模块、构建请求、读取响应、处理错误以及一些高级用法。掌握这些技能可以帮助你在各种网络请求场景中更加得心应手。
在实际应用中,下载文件只是网络请求中的一个基本操作,urllib
库还支持许多其他功能,例如表单提交、Cookies处理等。建议读者进一步学习和探索,以便更好地应用于实际项目中。
以上就是关于如何在Python 3.6中使用urllib
进行下载的详细介绍。希望对你有所帮助。
相关问答FAQs:
如何在Python 3.6中使用urllib下载文件?
在Python 3.6中,可以使用urllib.request
模块来下载文件。示例代码如下:
import urllib.request
url = 'http://example.com/file.zip'
urllib.request.urlretrieve(url, 'local_filename.zip')
这段代码会将指定的文件下载到当前工作目录中,并命名为local_filename.zip
。确保替换url
为实际的文件地址。
在Python 3.6中使用urllib时,有哪些常见的错误需要注意?
使用urllib
时,常见的错误包括网络连接问题、URL格式错误以及权限问题。确保输入的URL是有效的,并且在运行代码时网络连接正常。此外,如果下载的文件需要特定的访问权限,请确保您有适当的权限。
如何在Python 3.6中使用urllib实现下载进度条?
要在下载过程中显示进度条,可以通过自定义回调函数来实现。以下是一个简单的示例:
import urllib.request
def download_progress(block_num, block_size, total_size):
downloaded = block_num * block_size
percentage = min(downloaded / total_size * 100, 100)
print(f'Downloaded: {percentage:.2f}%')
url = 'http://example.com/file.zip'
urllib.request.urlretrieve(url, 'local_filename.zip', reporthook=download_progress)
这个代码片段会在下载时显示下载进度的百分比。根据文件大小和下载进度,您可以更改输出的格式。
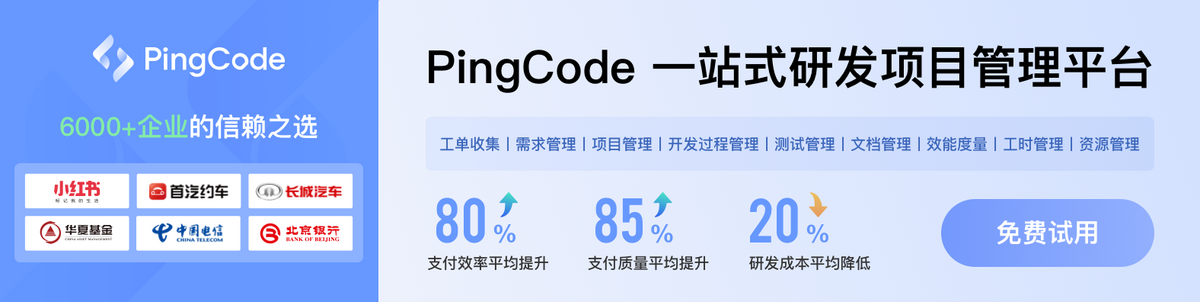