多线程时传入两个列表参数的核心要点是:使用Thread
对象的args
参数传递元组、使用线程池、使用全局变量。我们将展开详细描述如何使用Thread
对象的args
参数传递元组来实现这一点。
使用Thread
对象的args
参数传递元组:在Python的threading
模块中,我们可以通过将多个参数打包成一个元组,然后将这个元组传递给Thread
对象的args
参数,从而实现多线程时传入多个参数。这样做既简洁又有效。下面是具体的实现步骤。
一、使用Thread
对象的args
参数传递元组
在多线程编程中,我们通常会使用threading.Thread
类来创建和管理线程。下面是一个示例,演示了如何使用Thread
对象的args
参数传递两个列表参数。
import threading
def process_lists(list1, list2):
for i in range(len(list1)):
print(f"Processing {list1[i]} and {list2[i]}")
创建两个列表
list1 = [1, 2, 3, 4, 5]
list2 = ['a', 'b', 'c', 'd', 'e']
创建线程并传入两个列表作为参数
thread = threading.Thread(target=process_lists, args=(list1, list2))
thread.start()
thread.join()
在这个示例中,process_lists
函数接受两个列表作为参数,并在循环中处理它们。我们通过将两个列表打包成一个元组传递给Thread
对象的args
参数,实现了多线程时传入两个列表参数。
二、使用线程池(ThreadPoolExecutor)
另一个处理多线程时传入多个参数的方法是使用concurrent.futures
模块中的ThreadPoolExecutor
。这个模块提供了一个高级接口,可以更方便地管理线程。
from concurrent.futures import ThreadPoolExecutor
def process_lists(list1, list2):
for i in range(len(list1)):
print(f"Processing {list1[i]} and {list2[i]}")
创建两个列表
list1 = [1, 2, 3, 4, 5]
list2 = ['a', 'b', 'c', 'd', 'e']
使用线程池执行任务
with ThreadPoolExecutor(max_workers=2) as executor:
executor.submit(process_lists, list1, list2)
在这个示例中,我们使用ThreadPoolExecutor
创建了一个线程池,并通过submit
方法将任务提交给线程池执行。submit
方法接受可调用对象和参数,因此我们可以直接传递两个列表参数。
三、使用全局变量
虽然不推荐,但在某些情况下,我们可以使用全局变量来传递参数。需要注意的是,这种方法可能会导致线程安全问题,因此应谨慎使用。
import threading
创建两个全局列表
list1 = [1, 2, 3, 4, 5]
list2 = ['a', 'b', 'c', 'd', 'e']
def process_lists():
for i in range(len(list1)):
print(f"Processing {list1[i]} and {list2[i]}")
创建线程并执行任务
thread = threading.Thread(target=process_lists)
thread.start()
thread.join()
在这个示例中,我们将两个列表定义为全局变量,并在线程中直接访问它们。这种方法简单易行,但需要注意线程安全问题。
四、线程安全和锁机制
在多线程编程中,线程安全是一个重要的问题。为了确保多个线程在访问共享资源时不会发生冲突,我们可以使用线程锁(Lock)机制。
import threading
创建两个列表
list1 = [1, 2, 3, 4, 5]
list2 = ['a', 'b', 'c', 'd', 'e']
创建一个线程锁
lock = threading.Lock()
def process_lists():
with lock:
for i in range(len(list1)):
print(f"Processing {list1[i]} and {list2[i]}")
创建多个线程并执行任务
threads = []
for _ in range(3):
thread = threading.Thread(target=process_lists)
threads.append(thread)
thread.start()
for thread in threads:
thread.join()
在这个示例中,我们使用threading.Lock
创建了一个锁对象,并在访问共享资源时使用with lock
语句确保线程安全。
五、示例应用场景
以下是几个实际应用场景,展示了如何在多线程时传入两个列表参数:
数据处理
假设我们有两个列表,一个包含传感器数据,另一个包含时间戳。我们可以使用多线程并行处理这些数据。
import threading
sensor_data = [1.2, 2.4, 3.6, 4.8, 6.0]
timestamps = ['10:00', '10:01', '10:02', '10:03', '10:04']
def process_data(data, times):
for i in range(len(data)):
print(f"At {times[i]}, the sensor reading was {data[i]}")
thread = threading.Thread(target=process_data, args=(sensor_data, timestamps))
thread.start()
thread.join()
文件处理
我们可以使用多线程并行读取和处理多个文件。例如,一个列表包含文件名,另一个列表包含相应的操作。
import threading
filenames = ['file1.txt', 'file2.txt', 'file3.txt']
operations = ['read', 'write', 'append']
def process_files(files, ops):
for i in range(len(files)):
print(f"Performing {ops[i]} operation on {files[i]}")
thread = threading.Thread(target=process_files, args=(filenames, operations))
thread.start()
thread.join()
网络请求
我们可以使用多线程并行发送多个网络请求。例如,一个列表包含URL,另一个列表包含请求方法。
import threading
import requests
urls = ['http://example.com', 'http://example.org', 'http://example.net']
methods = ['GET', 'POST', 'GET']
def send_requests(urls, methods):
for i in range(len(urls)):
response = requests.request(methods[i], urls[i])
print(f"Response from {urls[i]}: {response.status_code}")
thread = threading.Thread(target=send_requests, args=(urls, methods))
thread.start()
thread.join()
六、总结
通过上述示例,我们详细介绍了在多线程编程中如何传入两个列表参数。我们可以使用Thread
对象的args
参数传递元组、使用线程池、使用全局变量,并确保线程安全。无论是在数据处理、文件处理还是网络请求中,这些方法都可以帮助我们更高效地完成任务。希望这些示例能够帮助您在实际项目中更好地应用多线程技术。
相关问答FAQs:
如何在Python多线程中传递多个列表参数?
在Python中,可以通过将多个列表作为参数传递给线程来实现多线程的需求。可以使用threading
模块中的Thread
类,并在创建线程时通过args
参数传递所需的列表。例如,定义一个目标函数,该函数接受多个列表作为参数,然后在创建线程时将这些列表作为元组传入。
在多线程中如何处理列表的共享和同步?
在处理共享列表时,确保线程安全是至关重要的。可以使用threading.Lock
来防止多个线程同时访问和修改同一个列表。通过在对列表进行操作之前获得锁,确保在操作完成后释放锁,这样可以防止数据竞争和不一致的问题。
如何检查多线程操作完成后列表的结果?
可以使用join()
方法来等待线程完成操作,确保所有线程执行结束后再进行结果的处理。通过在每个线程中使用返回值的方式,或者通过共享的结果列表,在主线程中收集各个线程的结果数据,从而实现对结果的验证和使用。
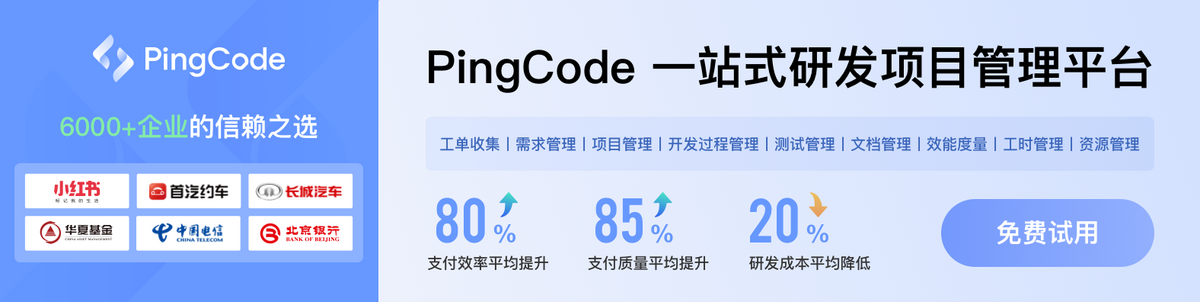