Python 列表的增删改查
Python 是一种非常灵活和强大的编程语言,其中列表(list)是最常用的数据结构之一。通过使用内置的方法和操作符,Python 允许我们轻松地对列表进行增、删、改、查等操作。本文将详细介绍如何在 Python 中实现这些操作,包括使用具体的代码示例和场景应用。
一、列表的增操作
1. 使用 append()
方法
append()
方法用于在列表的末尾添加一个元素。
fruits = ['apple', 'banana', 'cherry']
fruits.append('orange')
print(fruits) # 输出: ['apple', 'banana', 'cherry', 'orange']
2. 使用 insert()
方法
insert()
方法用于在列表的指定位置插入一个元素。
fruits = ['apple', 'banana', 'cherry']
fruits.insert(1, 'orange')
print(fruits) # 输出: ['apple', 'orange', 'banana', 'cherry']
3. 使用 extend()
方法
extend()
方法用于将另一个列表中的元素添加到当前列表的末尾。
fruits = ['apple', 'banana', 'cherry']
more_fruits = ['orange', 'mango']
fruits.extend(more_fruits)
print(fruits) # 输出: ['apple', 'banana', 'cherry', 'orange', 'mango']
二、列表的删操作
1. 使用 remove()
方法
remove()
方法用于删除列表中第一个匹配的元素。
fruits = ['apple', 'banana', 'cherry', 'banana']
fruits.remove('banana')
print(fruits) # 输出: ['apple', 'cherry', 'banana']
2. 使用 pop()
方法
pop()
方法用于删除列表中的一个元素(默认是最后一个),并返回该元素。
fruits = ['apple', 'banana', 'cherry']
removed_fruit = fruits.pop()
print(removed_fruit) # 输出: 'cherry'
print(fruits) # 输出: ['apple', 'banana']
3. 使用 del
关键字
del
关键字用于删除列表中的指定位置的元素。
fruits = ['apple', 'banana', 'cherry']
del fruits[1]
print(fruits) # 输出: ['apple', 'cherry']
三、列表的改操作
1. 通过索引修改元素
直接通过索引访问并修改列表中的元素。
fruits = ['apple', 'banana', 'cherry']
fruits[1] = 'orange'
print(fruits) # 输出: ['apple', 'orange', 'cherry']
2. 使用切片修改多个元素
可以使用切片语法一次修改多个元素。
fruits = ['apple', 'banana', 'cherry']
fruits[1:3] = ['orange', 'mango']
print(fruits) # 输出: ['apple', 'orange', 'mango']
四、列表的查操作
1. 使用索引查找元素
通过索引直接访问列表中的元素。
fruits = ['apple', 'banana', 'cherry']
print(fruits[1]) # 输出: 'banana'
2. 使用 index()
方法
index()
方法用于查找指定元素在列表中的位置。
fruits = ['apple', 'banana', 'cherry']
print(fruits.index('banana')) # 输出: 1
3. 使用 count()
方法
count()
方法用于统计某个元素在列表中出现的次数。
fruits = ['apple', 'banana', 'cherry', 'banana']
print(fruits.count('banana')) # 输出: 2
五、列表的高级操作
1. 列表的排序
使用 sort()
方法对列表进行排序。
numbers = [3, 1, 4, 1, 5, 9]
numbers.sort()
print(numbers) # 输出: [1, 1, 3, 4, 5, 9]
2. 列表的反转
使用 reverse()
方法对列表进行反转。
fruits = ['apple', 'banana', 'cherry']
fruits.reverse()
print(fruits) # 输出: ['cherry', 'banana', 'apple']
3. 列表的复制
使用 copy()
方法复制列表。
fruits = ['apple', 'banana', 'cherry']
fruits_copy = fruits.copy()
print(fruits_copy) # 输出: ['apple', 'banana', 'cherry']
六、列表的遍历操作
1. 使用 for
循环
通过 for
循环遍历列表中的每个元素。
fruits = ['apple', 'banana', 'cherry']
for fruit in fruits:
print(fruit)
2. 使用 while
循环
使用 while
循环结合索引遍历列表。
fruits = ['apple', 'banana', 'cherry']
i = 0
while i < len(fruits):
print(fruits[i])
i += 1
七、列表的嵌套操作
1. 创建嵌套列表
嵌套列表是指列表中的元素也是列表。
nested_list = [['apple', 'banana'], ['cherry', 'orange']]
print(nested_list) # 输出: [['apple', 'banana'], ['cherry', 'orange']]
2. 访问嵌套列表中的元素
通过多重索引访问嵌套列表中的元素。
nested_list = [['apple', 'banana'], ['cherry', 'orange']]
print(nested_list[1][0]) # 输出: 'cherry'
八、列表的内置函数
1. 使用 len()
函数
len()
函数用于获取列表的长度(元素的数量)。
fruits = ['apple', 'banana', 'cherry']
print(len(fruits)) # 输出: 3
2. 使用 sum()
函数
sum()
函数用于计算列表中所有数值元素的和。
numbers = [1, 2, 3, 4, 5]
print(sum(numbers)) # 输出: 15
3. 使用 min()
和 max()
函数
min()
和 max()
函数分别用于找到列表中的最小值和最大值。
numbers = [1, 2, 3, 4, 5]
print(min(numbers)) # 输出: 1
print(max(numbers)) # 输出: 5
九、列表的综合应用
1. 列表推导式
列表推导式是一种简洁的生成列表的方法。
squares = [x2 for x in range(10)]
print(squares) # 输出: [0, 1, 4, 9, 16, 25, 36, 49, 64, 81]
2. 列表与其他数据类型的转换
可以使用 list()
函数将其他数据类型(如元组、字符串)转换为列表。
# 从字符串转换为列表
string = "hello"
string_list = list(string)
print(string_list) # 输出: ['h', 'e', 'l', 'l', 'o']
从元组转换为列表
tuple_data = (1, 2, 3)
tuple_list = list(tuple_data)
print(tuple_list) # 输出: [1, 2, 3]
十、列表的性能优化
1. 尽量避免频繁的增删操作
频繁的增删操作会导致列表的性能下降,尤其是在大列表中。尽量在可能的情况下一次性完成所有的增删操作。
2. 使用生成器表达式
对于需要生成大数据集的情况,使用生成器表达式比列表推导式更为高效,因为生成器表达式不会一次性生成整个数据集,而是按需生成。
gen = (x2 for x in range(10))
for val in gen:
print(val)
3. 使用 deque
进行高效的插入和删除操作
Python 的 collections
模块提供了 deque
数据结构,它在两端的插入和删除操作都非常高效。
from collections import deque
d = deque(['apple', 'banana', 'cherry'])
d.append('orange')
print(d) # 输出: deque(['apple', 'banana', 'cherry', 'orange'])
d.appendleft('mango')
print(d) # 输出: deque(['mango', 'apple', 'banana', 'cherry', 'orange'])
总结,Python 列表提供了丰富的操作方法,能够满足各种应用需求。通过本文的学习,你应该能够熟练掌握列表的增删改查操作,并在实际项目中灵活应用这些知识。
相关问答FAQs:
如何在Python中向列表添加新元素?
在Python中,可以使用append()
方法将元素添加到列表的末尾,或使用insert()
方法在指定位置插入元素。例如,my_list.append('新元素')
会在列表末尾添加“新元素”,而my_list.insert(0, '新元素')
则会将其插入到列表的第一个位置。
Python中如何删除列表中的元素?
要删除列表中的元素,可以使用remove()
方法删除指定值的第一个匹配项,或使用pop()
方法根据索引删除并返回元素。例如,my_list.remove('要删除的元素')
会删除列表中第一个出现的“要删除的元素”,而my_list.pop(1)
则会删除并返回索引为1的元素。
如何在Python中修改列表中的特定元素?
修改列表中特定元素的值非常简单。你只需通过索引访问该元素并赋予其新的值。例如,my_list[2] = '新值'
会将索引为2的元素更新为“新值”。确保索引在列表的范围内,以避免引发IndexError
。
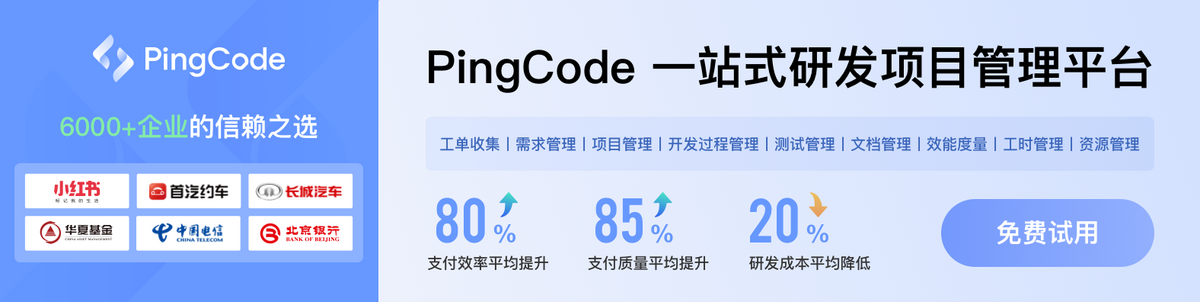