Python如何将txt只保留汉字字符
使用正则表达式提取汉字字符、遍历文本文件逐行处理、保留并输出提取后的汉字字符。为了在Python中将txt文件中的内容只保留汉字字符,可以使用正则表达式进行匹配和提取。正则表达式是一种强大的工具,可以用来匹配文本中的特定模式。通过遍历txt文件的每一行,将每一行中的汉字字符提取出来并写入新的文件中,可以实现需求。以下是详细描述。
一、正则表达式提取汉字字符
正则表达式是一种描述文本模式的语法,可以用于文本搜索、替换和提取。Python的re
模块提供了丰富的正则表达式功能。我们可以使用正则表达式来匹配中文字符。
1. 正则表达式基本概念
正则表达式是一种用来描述或者匹配字符串的工具。它具有极高的灵活性和强大的功能,能够方便地对字符串进行复杂的查找、替换、提取等操作。在Python中,使用re
模块来实现正则表达式的功能。
2. 匹配汉字字符的正则表达式
在Python中,可以使用正则表达式来匹配汉字字符。汉字的Unicode编码范围是[\u4e00-\u9fa5]
。因此,可以使用如下的正则表达式来匹配汉字字符:
import re
def extract_chinese_characters(text):
pattern = re.compile(r'[\u4e00-\u9fa5]')
return ''.join(pattern.findall(text))
二、遍历文本文件逐行处理
为了处理txt文件中的内容,我们需要逐行读取文件,并对每一行进行汉字字符的提取。可以使用Python的内置文件操作函数来实现这一功能。
1. 读取txt文件内容
首先,需要打开txt文件并逐行读取其中的内容。可以使用open
函数以读模式打开文件,并使用readlines
方法读取文件的所有行。
def read_file(file_path):
with open(file_path, 'r', encoding='utf-8') as file:
lines = file.readlines()
return lines
2. 逐行处理并提取汉字字符
在读取文件内容后,可以逐行处理每一行,并使用正则表达式提取其中的汉字字符。处理后的结果可以存储在一个列表中。
def process_lines(lines):
processed_lines = []
for line in lines:
processed_line = extract_chinese_characters(line)
processed_lines.append(processed_line)
return processed_lines
三、保留并输出提取后的汉字字符
在提取并处理txt文件中的汉字字符后,需要将处理后的内容写入新的文件中。可以使用open
函数以写模式打开新的文件,并使用writelines
方法将处理后的内容写入文件。
1. 写入新文件
可以将处理后的内容写入新的txt文件中,以便后续使用。
def write_file(file_path, lines):
with open(file_path, 'w', encoding='utf-8') as file:
file.writelines(lines)
2. 完整示例代码
以下是完整的示例代码,展示了如何读取txt文件内容、提取汉字字符并写入新文件。
import re
def extract_chinese_characters(text):
pattern = re.compile(r'[\u4e00-\u9fa5]')
return ''.join(pattern.findall(text))
def read_file(file_path):
with open(file_path, 'r', encoding='utf-8') as file:
lines = file.readlines()
return lines
def process_lines(lines):
processed_lines = []
for line in lines:
processed_line = extract_chinese_characters(line)
processed_lines.append(processed_line + '\n')
return processed_lines
def write_file(file_path, lines):
with open(file_path, 'w', encoding='utf-8') as file:
file.writelines(lines)
def main(input_file, output_file):
lines = read_file(input_file)
processed_lines = process_lines(lines)
write_file(output_file, processed_lines)
if __name__ == '__main__':
input_file = 'input.txt'
output_file = 'output.txt'
main(input_file, output_file)
四、处理大文件的优化方法
如果txt文件非常大,一次性将所有内容读入内存可能会导致内存不足的问题。为了处理大文件,可以逐行读取和处理文件内容,并将处理后的内容逐行写入新的文件中。
1. 逐行读取和处理
可以使用open
函数以读模式打开文件,并使用readline
方法逐行读取文件内容。每读取一行内容后,立即处理并写入新的文件中。
def process_large_file(input_file, output_file):
with open(input_file, 'r', encoding='utf-8') as infile, open(output_file, 'w', encoding='utf-8') as outfile:
for line in infile:
processed_line = extract_chinese_characters(line)
outfile.write(processed_line + '\n')
2. 完整示例代码
以下是处理大文件的完整示例代码,展示了如何逐行读取、处理并写入新文件。
import re
def extract_chinese_characters(text):
pattern = re.compile(r'[\u4e00-\u9fa5]')
return ''.join(pattern.findall(text))
def process_large_file(input_file, output_file):
with open(input_file, 'r', encoding='utf-8') as infile, open(output_file, 'w', encoding='utf-8') as outfile:
for line in infile:
processed_line = extract_chinese_characters(line)
outfile.write(processed_line + '\n')
def main(input_file, output_file):
process_large_file(input_file, output_file)
if __name__ == '__main__':
input_file = 'input.txt'
output_file = 'output.txt'
main(input_file, output_file)
五、处理特殊字符和格式
在实际应用中,txt文件中可能包含一些特殊字符或格式,例如空格、标点符号、数字等。在提取汉字字符时,可以选择保留或删除这些特殊字符和格式。
1. 保留空格和标点符号
如果需要保留空格和标点符号,可以在正则表达式中添加相应的匹配模式。例如,可以使用[\u4e00-\u9fa5\s,。!?]
来匹配汉字字符、空格和常见的中文标点符号。
def extract_chinese_characters_with_punctuation(text):
pattern = re.compile(r'[\u4e00-\u9fa5\s,。!?]')
return ''.join(pattern.findall(text))
2. 删除特殊字符和格式
如果需要删除特殊字符和格式,只保留汉字字符,可以使用之前的正则表达式[\u4e00-\u9fa5]
。
def extract_chinese_characters(text):
pattern = re.compile(r'[\u4e00-\u9fa5]')
return ''.join(pattern.findall(text))
六、总结与扩展
通过本文所述的方法,可以在Python中方便地将txt文件中的内容只保留汉字字符。使用正则表达式提取汉字字符、逐行处理txt文件内容,并将处理后的内容写入新文件,可以实现这一需求。对于大文件,可以逐行读取和处理,以避免内存不足的问题。
此外,根据实际需求,还可以选择保留或删除特殊字符和格式。通过灵活使用正则表达式,可以实现对文本内容的各种复杂操作。
扩展阅读
- 正则表达式的深入学习:正则表达式是一种非常强大的工具,可以用于文本匹配、替换和提取。深入学习正则表达式的语法和使用技巧,可以帮助我们更高效地处理文本数据。
- Python文件操作技巧:在处理大文件时,逐行读取和处理是一种常见的优化方法。学习更多Python文件操作的技巧,可以帮助我们更高效地处理各种文件格式的数据。
- 文本数据清洗和预处理:在数据分析和机器学习中,文本数据的清洗和预处理是非常重要的步骤。了解更多文本数据清洗和预处理的方法,可以帮助我们更好地准备数据用于后续的分析和建模。
通过这些扩展阅读,可以进一步提高我们在处理文本数据方面的能力和技巧。
相关问答FAQs:
如何使用Python读取txt文件并保留汉字字符?
使用Python读取txt文件时,可以通过正则表达式来筛选出汉字字符。可以使用re
模块中的findall
函数来提取汉字。首先,打开文件并读取内容,然后应用正则表达式匹配汉字。示例代码如下:
import re
# 读取文件
with open('yourfile.txt', 'r', encoding='utf-8') as file:
content = file.read()
# 提取汉字
hanzi = re.findall(r'[\u4e00-\u9fa5]+', content)
result = ''.join(hanzi)
# 输出或保存结果
print(result)
如何确保在提取汉字时不会丢失其他重要信息?
在提取汉字字符时,可以考虑在提取前先备份原文件,确保不影响原始内容。此外,还可以在提取过程中记录其他需要的信息,例如汉字出现的上下文,以便后续使用。使用findall
时,可以调整正则表达式,以包含特定的标点符号或其他字符。
如何将提取的汉字字符写入新的txt文件?
提取完汉字后,可以使用Python的写文件功能将结果保存到新文件中。使用open
函数以写模式打开新文件,并写入提取的汉字字符串。示例代码如下:
with open('output.txt', 'w', encoding='utf-8') as output_file:
output_file.write(result)
这样可以将提取的汉字字符保存到output.txt
文件中,方便后续查看和使用。
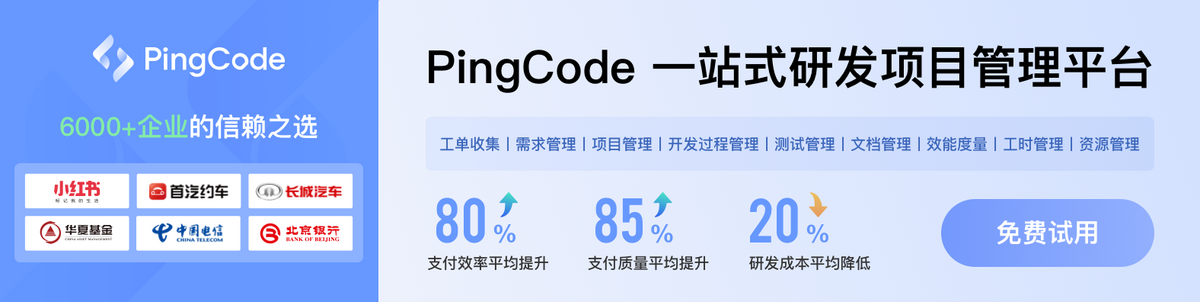