Python 写客户资料的方法
Python 写客户资料的方法有多种,包括使用字典、类、CSV 文件等方式。本文将详细介绍其中一种方法,即使用类来管理客户资料。
具体来说,使用类可以让代码更加模块化和易维护。我们可以创建一个客户类,包含客户的基本信息,如姓名、联系方式、地址等。然后,通过实例化该类来创建不同客户对象,并将这些对象存储在列表或字典中进行管理。
一、创建客户类
在 Python 中,类是一种用于定义对象的模板。我们可以使用类来表示客户,并为每个客户对象存储其属性和方法。
class Customer:
def __init__(self, name, contact, address):
self.name = name
self.contact = contact
self.address = address
def update_contact(self, new_contact):
self.contact = new_contact
def update_address(self, new_address):
self.address = new_address
def display_info(self):
print(f"Name: {self.name}")
print(f"Contact: {self.contact}")
print(f"Address: {self.address}")
在上述代码中,我们定义了一个 Customer
类,并在其初始化方法 __init__
中设置了 name
、contact
和 address
属性。此外,还定义了两个更新方法 update_contact
和 update_address
,以及一个显示信息的方法 display_info
。
二、创建客户对象并管理
创建客户对象后,我们可以将其存储在列表或字典中进行管理。下面是一个示例,展示了如何创建客户对象并将其存储在列表中。
# 创建客户对象
customer1 = Customer("Alice", "123-456-7890", "123 Main St")
customer2 = Customer("Bob", "098-765-4321", "456 Elm St")
将客户对象存储在列表中
customer_list = [customer1, customer2]
显示所有客户信息
for customer in customer_list:
customer.display_info()
print("--------")
在这个示例中,我们创建了两个客户对象 customer1
和 customer2
,并将它们存储在 customer_list
列表中。然后,通过循环遍历列表中的每个客户对象,调用 display_info
方法显示客户信息。
三、使用 CSV 文件存储客户资料
有时候我们可能需要将客户资料存储在文件中,以便长期保存和读取。CSV 文件是一种常见的文本文件格式,适用于存储表格数据。我们可以使用 Python 的 csv
模块来读取和写入 CSV 文件。
import csv
写入客户资料到 CSV 文件
def write_customers_to_csv(customers, filename):
with open(filename, 'w', newline='') as csvfile:
fieldnames = ['Name', 'Contact', 'Address']
writer = csv.DictWriter(csvfile, fieldnames=fieldnames)
writer.writeheader()
for customer in customers:
writer.writerow({
'Name': customer.name,
'Contact': customer.contact,
'Address': customer.address
})
读取 CSV 文件中的客户资料
def read_customers_from_csv(filename):
customers = []
with open(filename, 'r') as csvfile:
reader = csv.DictReader(csvfile)
for row in reader:
customer = Customer(row['Name'], row['Contact'], row['Address'])
customers.append(customer)
return customers
示例:将客户资料写入 CSV 文件,然后读取并显示
write_customers_to_csv(customer_list, 'customers.csv')
loaded_customers = read_customers_from_csv('customers.csv')
for customer in loaded_customers:
customer.display_info()
print("--------")
在这段代码中,我们定义了两个函数 write_customers_to_csv
和 read_customers_from_csv
。第一个函数将客户对象列表写入 CSV 文件,第二个函数从 CSV 文件中读取客户数据并返回客户对象列表。
四、使用 SQLite 数据库存储客户资料
SQLite 是一种轻量级的关系型数据库,可以方便地嵌入到 Python 应用程序中。我们可以使用 SQLite 来存储和管理客户资料。
import sqlite3
创建数据库连接和表
def create_db_and_table(db_name):
conn = sqlite3.connect(db_name)
cursor = conn.cursor()
cursor.execute('''CREATE TABLE IF NOT EXISTS customers
(name TEXT, contact TEXT, address TEXT)''')
conn.commit()
conn.close()
插入客户资料到数据库
def insert_customer_to_db(db_name, customer):
conn = sqlite3.connect(db_name)
cursor = conn.cursor()
cursor.execute('''INSERT INTO customers (name, contact, address)
VALUES (?, ?, ?)''', (customer.name, customer.contact, customer.address))
conn.commit()
conn.close()
从数据库中读取客户资料
def read_customers_from_db(db_name):
conn = sqlite3.connect(db_name)
cursor = conn.cursor()
cursor.execute('SELECT * FROM customers')
rows = cursor.fetchall()
conn.close()
customers = []
for row in rows:
customer = Customer(row[0], row[1], row[2])
customers.append(customer)
return customers
示例:创建数据库和表,插入客户资料,然后读取并显示
db_name = 'customers.db'
create_db_and_table(db_name)
for customer in customer_list:
insert_customer_to_db(db_name, customer)
loaded_customers = read_customers_from_db(db_name)
for customer in loaded_customers:
customer.display_info()
print("--------")
在这段代码中,我们定义了三个函数 create_db_and_table
、insert_customer_to_db
和 read_customers_from_db
。第一个函数创建数据库连接并创建客户表,第二个函数将客户对象插入数据库,第三个函数从数据库中读取客户数据并返回客户对象列表。
五、使用 JSON 文件存储客户资料
JSON(JavaScript Object Notation)是一种轻量级的数据交换格式,易于人类阅读和编写,也易于机器解析和生成。我们可以使用 JSON 文件来存储客户资料。
import json
写入客户资料到 JSON 文件
def write_customers_to_json(customers, filename):
data = []
for customer in customers:
data.append({
'name': customer.name,
'contact': customer.contact,
'address': customer.address
})
with open(filename, 'w') as jsonfile:
json.dump(data, jsonfile)
从 JSON 文件中读取客户资料
def read_customers_from_json(filename):
customers = []
with open(filename, 'r') as jsonfile:
data = json.load(jsonfile)
for item in data:
customer = Customer(item['name'], item['contact'], item['address'])
customers.append(customer)
return customers
示例:将客户资料写入 JSON 文件,然后读取并显示
write_customers_to_json(customer_list, 'customers.json')
loaded_customers = read_customers_from_json('customers.json')
for customer in loaded_customers:
customer.display_info()
print("--------")
在这段代码中,我们定义了两个函数 write_customers_to_json
和 read_customers_from_json
。第一个函数将客户对象列表写入 JSON 文件,第二个函数从 JSON 文件中读取客户数据并返回客户对象列表。
六、总结
通过以上几个示例,我们展示了如何使用 Python 写客户资料的方法,包括使用类、CSV 文件、SQLite 数据库和 JSON 文件。每种方法都有其优点和适用场景,具体选择哪种方法取决于具体需求。
使用类的方式使代码更加模块化和易维护,适合较小规模的项目;使用 CSV 文件适合简单的文本数据存储,易于与其他应用程序交换数据;使用 SQLite 数据库适合需要进行复杂查询和管理的数据,适合中小型项目;使用 JSON 文件适合需要与 Web 应用程序进行数据交换的场景。
希望本文能帮助你更好地理解如何使用 Python 写客户资料,并选择最适合自己项目的方法。
相关问答FAQs:
如何在Python中创建客户资料的基本结构?
在Python中,您可以使用字典或类来创建客户资料的基本结构。使用字典的方式可以快速定义客户的各种信息,例如姓名、电话和地址。示例代码如下:
customer_profile = {
"name": "张三",
"phone": "123456789",
"address": "北京市朝阳区"
}
如果需要更复杂的功能,可以定义一个类来表示客户资料。这样可以通过方法来处理客户的相关操作。
如何存储和读取客户资料到文件中?
存储客户资料到文件中可以使用JSON格式,这样可以方便地进行读取和写入。可以通过json
模块实现,如下所示:
import json
# 存储客户资料
with open('customer.json', 'w') as f:
json.dump(customer_profile, f)
# 读取客户资料
with open('customer.json', 'r') as f:
loaded_profile = json.load(f)
这种方式既方便又易于管理,可以确保客户资料的持久化存储。
如何对客户资料进行基本的验证和更新?
在创建客户资料时,验证数据的有效性是非常重要的。可以编写简单的函数来检查客户的姓名和电话格式。更新客户资料时,可以直接修改字典中的内容或使用类的方法。示例代码如下:
def validate_customer(customer):
if not customer['name']:
return "姓名不能为空"
if len(customer['phone']) != 9:
return "电话格式不正确"
return "客户资料有效"
# 更新客户资料
customer_profile['phone'] = "987654321"
这种方法可以确保客户信息的准确性和完整性。
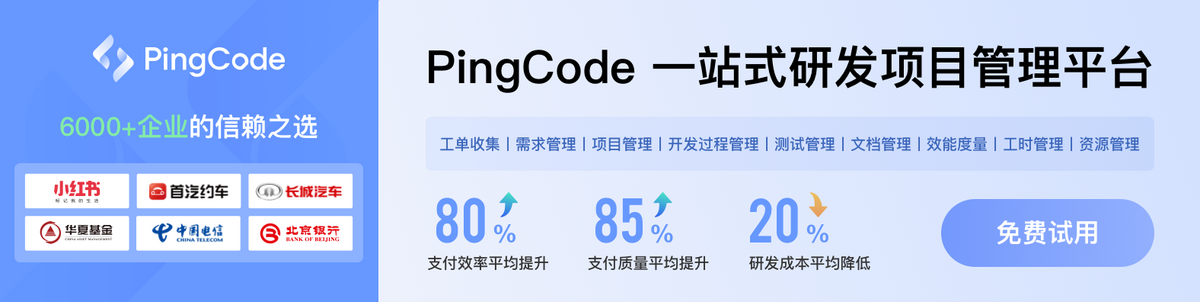