要对比Python列表中下标相同的图片,可以使用以下几种方法:逐像素比较、使用哈希值比较、计算图像特征向量等。其中,逐像素比较是最直接的方式,但效率较低;使用哈希值比较较为高效且简单;计算图像特征向量则适用于复杂图像的比较。本文将详细介绍逐像素比较和哈希值比较的方法,并以计算图像特征向量的方法作为补充。
一、逐像素比较
逐像素比较方法通过逐一比较两张图片的每一个像素值来判断它们是否相同。这种方法简单直观,但计算复杂度较高,适用于小尺寸图像的比较。
1.1 使用Pillow库逐像素比较
Pillow是Python中一个强大的图像处理库,能够方便地读取和处理图片。
from PIL import Image
import numpy as np
def compare_images_pixel_by_pixel(img1_path, img2_path):
img1 = Image.open(img1_path)
img2 = Image.open(img2_path)
# 将图片转换为numpy数组
arr1 = np.array(img1)
arr2 = np.array(img2)
# 判断两张图片的尺寸是否相同
if arr1.shape != arr2.shape:
return False
# 比较每一个像素值
comparison = np.all(arr1 == arr2)
return comparison
示例代码
img1_path = 'path_to_image1.jpg'
img2_path = 'path_to_image2.jpg'
print(compare_images_pixel_by_pixel(img1_path, img2_path))
这种方法对像素级别的差异非常敏感,适合完全相同的图片比较。
1.2 使用OpenCV逐像素比较
OpenCV是一个开源的计算机视觉库,功能强大,支持多种图像处理操作。
import cv2
def compare_images_pixel_by_pixel_opencv(img1_path, img2_path):
img1 = cv2.imread(img1_path)
img2 = cv2.imread(img2_path)
# 判断两张图片的尺寸是否相同
if img1.shape != img2.shape:
return False
# 比较每一个像素值
comparison = (img1 == img2).all()
return comparison
示例代码
img1_path = 'path_to_image1.jpg'
img2_path = 'path_to_image2.jpg'
print(compare_images_pixel_by_pixel_opencv(img1_path, img2_path))
二、哈希值比较
哈希值比较方法通过生成图片的哈希值来判断图片是否相同。常用的哈希算法有感知哈希(pHash)、差异哈希(dHash)和平均哈希(aHash)。
2.1 使用ImageHash库进行哈希值比较
ImageHash是一个专门用于生成图像哈希值的库,支持多种哈希算法。
import imagehash
from PIL import Image
def compare_images_with_hash(img1_path, img2_path, hash_func=imagehash.average_hash):
img1 = Image.open(img1_path)
img2 = Image.open(img2_path)
hash1 = hash_func(img1)
hash2 = hash_func(img2)
return hash1 == hash2
示例代码
img1_path = 'path_to_image1.jpg'
img2_path = 'path_to_image2.jpg'
print(compare_images_with_hash(img1_path, img2_path, imagehash.phash))
哈希值比较方法对图片的轻微变化(如压缩、旋转、缩放等)有较高的容忍度,适用于实际应用中图片相似性的比较。
三、计算图像特征向量
计算图像特征向量的方法通过提取图像的特征来判断图片是否相同。常用的特征提取方法有SIFT、SURF、ORB等。
3.1 使用OpenCV计算ORB特征向量
ORB(Oriented FAST and Rotated BRIEF)是一种快速且高效的特征点检测与描述方法,适用于实时应用。
import cv2
def compare_images_with_orb(img1_path, img2_path, threshold=30):
img1 = cv2.imread(img1_path, 0)
img2 = cv2.imread(img2_path, 0)
orb = cv2.ORB_create()
# 检测特征点并计算描述子
kp1, des1 = orb.detectAndCompute(img1, None)
kp2, des2 = orb.detectAndCompute(img2, None)
# 创建BFMatcher对象
bf = cv2.BFMatcher(cv2.NORM_HAMMING, crossCheck=True)
# 匹配描述子
matches = bf.match(des1, des2)
# 计算匹配的距离
distances = [m.distance for m in matches]
# 计算平均距离
avg_distance = sum(distances) / len(distances)
# 判断平均距离是否小于阈值
return avg_distance < threshold
示例代码
img1_path = 'path_to_image1.jpg'
img2_path = 'path_to_image2.jpg'
print(compare_images_with_orb(img1_path, img2_path, threshold=30))
四、整合多种方法进行图片比较
在实际应用中,可以根据需要选择合适的方法进行图片比较,甚至可以组合多种方法来提高比较的准确性和效率。
4.1 综合使用逐像素比较和哈希值比较
def comprehensive_compare_images(img1_path, img2_path, hash_func=imagehash.average_hash, threshold=30):
# 逐像素比较
pixel_comparison_result = compare_images_pixel_by_pixel_opencv(img1_path, img2_path)
# 哈希值比较
hash_comparison_result = compare_images_with_hash(img1_path, img2_path, hash_func)
# ORB特征比较
orb_comparison_result = compare_images_with_orb(img1_path, img2_path, threshold)
# 综合判断
return pixel_comparison_result or hash_comparison_result or orb_comparison_result
示例代码
img1_path = 'path_to_image1.jpg'
img2_path = 'path_to_image2.jpg'
print(comprehensive_compare_images(img1_path, img2_path, imagehash.phash, threshold=30))
通过综合使用多种方法,可以提高图片比较的准确性和鲁棒性,适用于不同场景下的图片相似性比较。
五、总结
本文详细介绍了Python中几种常用的图片比较方法,包括逐像素比较、哈希值比较和计算图像特征向量。逐像素比较适用于完全相同的图片比较,哈希值比较方法对图片的轻微变化有较高的容忍度,计算图像特征向量的方法适用于复杂图像的比较。在实际应用中,可以根据需要选择合适的方法,甚至可以综合使用多种方法来提高比较的准确性和效率。
核心观点:逐像素比较、哈希值比较、计算图像特征向量、综合使用多种方法。
相关问答FAQs:
如何在Python中识别列表中相同的图片?
在Python中,可以利用图像处理库如OpenCV或PIL(Pillow)来对比图片。首先,加载所有图片并将其转换为可比较的格式,例如缩放到相同的尺寸或转换为灰度图像。接着,使用哈希函数或其他相似度算法(如SSIM或MSE)来比较图像,找出相同的图片。
是否可以使用第三方库来简化图片对比过程?
确实有许多第三方库可以帮助简化图片对比的过程。例如,使用imagehash
库可以快速生成图像的哈希值,然后通过比较哈希值来判断图片是否相同。此外,face_recognition
库也可以用于识别和比较人脸图像,适合处理包含人脸的图片集。
如何处理列表中相似但不完全相同的图片?
对于相似但不完全相同的图片,可以使用特征匹配方法进行比较。OpenCV提供了一些强大的算法,如SIFT(尺度不变特征变换)和ORB(方向稳健特征),可以检测和匹配图像中的特征点。这种方法能够有效地识别出图片之间的相似性,即便它们在细节上有所不同。
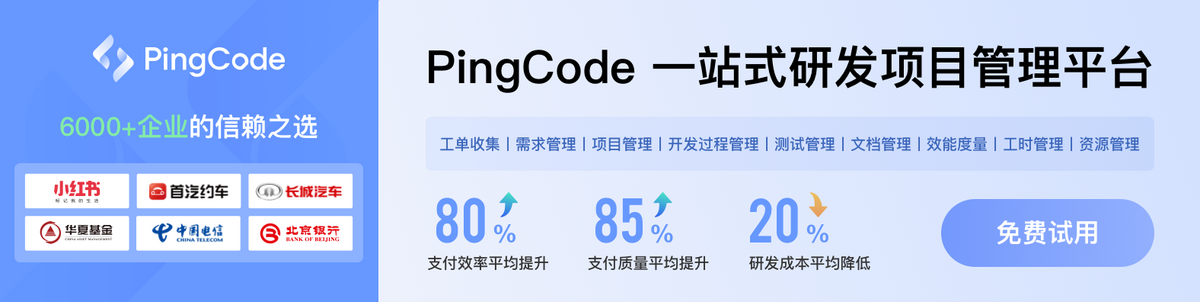