在Python中截取字符串的方法主要有:切片、正则表达式、字符串方法、find()和index()方法。本文将详细介绍这些方法,并提供实用的代码示例和最佳实践。
一、切片
Python中的切片操作是截取字符串的最常用方式。切片不仅简单直观,而且效率高。切片语法为string[start:end:step]
,其中start
是起始索引,end
是结束索引,step
是步长。
1. 基本切片操作
基本的切片操作包括从字符串的开头、中间或结尾截取子字符串。例如:
# 从字符串的开头到第5个字符
s = "Hello, World!"
print(s[:5]) # 输出: Hello
从字符串的第7个字符到结尾
print(s[7:]) # 输出: World!
从字符串的第2个字符到第8个字符
print(s[2:8]) # 输出: llo, W
2. 使用步长
步长参数允许你以固定的步长截取字符串。例如,每隔一个字符截取一次:
s = "Hello, World!"
print(s[::2]) # 输出: Hlo ol!
二、正则表达式
正则表达式是一种强大的字符串处理工具,适用于复杂的字符串匹配和截取。Python的re
模块提供了正则表达式的支持。
1. 基本匹配
通过正则表达式匹配子字符串并截取:
import re
s = "The price is $123.45"
pattern = r'\$\d+\.\d{2}'
match = re.search(pattern, s)
if match:
print(match.group()) # 输出: $123.45
2. 使用捕获组
捕获组允许你提取子字符串的特定部分:
s = "My phone number is 123-456-7890"
pattern = r'(\d{3})-(\d{3})-(\d{4})'
match = re.search(pattern, s)
if match:
print(match.group(1)) # 输出: 123
print(match.group(2)) # 输出: 456
print(match.group(3)) # 输出: 7890
三、字符串方法
Python内置的字符串方法如split()
、join()
、replace()
等,也可以用于截取字符串。
1. split()方法
split()
方法将字符串拆分为子字符串列表:
s = "apple,banana,cherry"
fruits = s.split(',')
print(fruits) # 输出: ['apple', 'banana', 'cherry']
2. partition()方法
partition()
方法根据指定的分隔符将字符串分为三部分:
s = "hello world"
first, sep, rest = s.partition(' ')
print(first) # 输出: hello
print(rest) # 输出: world
四、find()和index()方法
find()
和index()
方法用于查找子字符串在字符串中的位置,然后可以利用这些位置进行截取。
1. find()方法
find()
方法返回子字符串的第一个匹配位置:
s = "The quick brown fox"
pos = s.find('quick')
if pos != -1:
print(s[pos:pos+5]) # 输出: quick
2. index()方法
index()
方法类似于find()
,但在找不到匹配时会抛出异常:
s = "The quick brown fox"
try:
pos = s.index('brown')
print(s[pos:pos+5]) # 输出: brown
except ValueError:
print("Substring not found")
五、综合示例
最后,通过一个综合示例来展示如何结合多种方法截取字符串:
import re
s = "Contact us at support@example.com or call 123-456-7890"
使用正则表达式提取电子邮件
email_pattern = r'\b[A-Za-z0-9._%+-]+@[A-Za-z0-9.-]+\.[A-Z|a-z]{2,}\b'
email = re.search(email_pattern, s)
if email:
print("Email:", email.group())
使用find()和切片提取电话号码
phone_start = s.find('call') + len('call ')
phone_end = phone_start + 12 # 假设电话号码固定为12个字符
phone = s[phone_start:phone_end]
print("Phone:", phone)
通过以上方法和示例,相信你已经掌握了在Python中截取字符串的多种途径。每种方法都有其适用的场景,根据具体需求选择最合适的方法,能够提高代码的效率和可读性。
相关问答FAQs:
在Python中,如何使用切片来截取字符串?
切片是Python中处理字符串的一种强大功能。通过使用冒号运算符,可以轻松截取字符串的特定部分。例如,string[start:end]
可以提取从start
索引到end
索引之间的字符。如果想要从字符串中提取前五个字符,可以使用string[:5]
。要提取从第六个字符到最后一个字符,可以使用string[5:]
。
如何在Python中查找特定子字符串的索引位置?
使用str.find()
方法可以轻松获取特定子字符串的索引。如果子字符串存在于字符串中,返回其首次出现的位置;如果不存在,则返回-1。例如,string.find('子字符串')
会返回子字符串的起始索引。这在截取字符串时非常有用,因可以确定开始和结束位置。
Python中是否有其他方法可以截取字符串?
除了切片和str.find()
,可以使用str.split()
方法将字符串按指定分隔符拆分成列表。例如,string.split(',')
会将字符串按逗号拆分为多个部分。然后,可以根据需要从列表中选择特定部分进行截取。这种方法在处理复杂字符串时尤其有效。
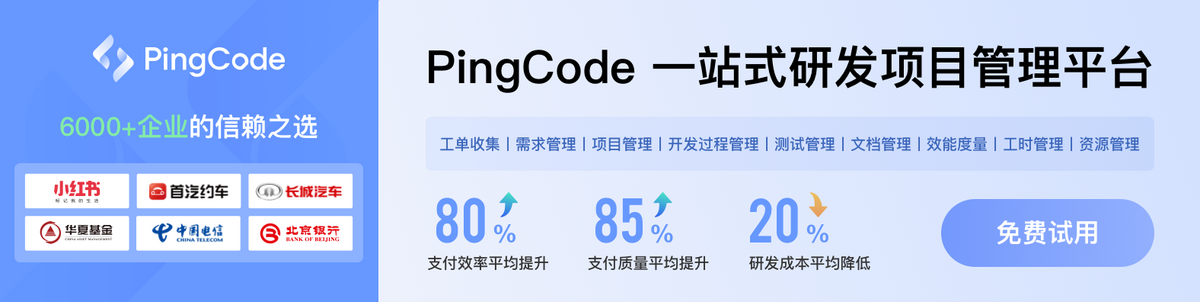