如何用Python爬上万条数据
使用Python爬上万条数据的关键在于掌握正确的爬虫工具、优化代码性能、处理反爬机制、和管理数据存储。 其中,选择合适的爬虫工具、如Scrapy或BeautifulSoup,能够显著提升爬虫效率。下面详细解释如何使用这些工具进行大规模数据爬取。
一、选择合适的爬虫工具
1. Scrapy
Scrapy 是一个功能强大且灵活的爬虫框架,非常适合大规模数据爬取。它支持多线程抓取,速度快,且有丰富的扩展功能,可以处理复杂的网页结构。
- 安装与配置:使用
pip install scrapy
进行安装,创建项目后通过编写spider来定义爬取逻辑。 - 示例代码:
import scrapy
class MySpider(scrapy.Spider):
name = 'my_spider'
start_urls = ['http://example.com']
def parse(self, response):
for item in response.css('div.item'):
yield {
'title': item.css('span.title::text').get(),
'link': item.css('a::attr(href)').get(),
}
2. BeautifulSoup
BeautifulSoup 是一个用于解析HTML和XML文档的库,适合小规模数据爬取或需要灵活处理网页内容的情况。它与requests库搭配使用,能够快速抓取并解析网页。
- 安装与配置:使用
pip install beautifulsoup4 requests
进行安装。 - 示例代码:
import requests
from bs4 import BeautifulSoup
response = requests.get('http://example.com')
soup = BeautifulSoup(response.content, 'html.parser')
for item in soup.select('div.item'):
title = item.select_one('span.title').get_text()
link = item.select_one('a')['href']
print({'title': title, 'link': link})
二、优化代码性能
1. 多线程与异步爬取
为了加快爬取速度,可以使用多线程或异步爬取工具如 concurrent.futures
模块或 aiohttp
库。
-
多线程示例:
from concurrent.futures import ThreadPoolExecutor
import requests
def fetch(url):
response = requests.get(url)
return response.content
urls = ['http://example.com/page1', 'http://example.com/page2']
with ThreadPoolExecutor(max_workers=5) as executor:
results = executor.map(fetch, urls)
-
异步爬取示例:
import aiohttp
import asyncio
async def fetch(session, url):
async with session.get(url) as response:
return await response.text()
async def main():
async with aiohttp.ClientSession() as session:
urls = ['http://example.com/page1', 'http://example.com/page2']
tasks = [fetch(session, url) for url in urls]
results = await asyncio.gather(*tasks)
asyncio.run(main())
2. 请求头与代理池
大规模爬取时,网站可能会有反爬机制,通过定期更改请求头和使用代理池可以有效规避这些问题。
-
请求头设置:
headers = {
'User-Agent': 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/58.0.3029.110 Safari/537.3'
}
response = requests.get('http://example.com', headers=headers)
-
代理池使用:
proxies = {
'http': 'http://10.10.10.10:8000',
'https': 'http://10.10.10.10:8000',
}
response = requests.get('http://example.com', proxies=proxies)
三、处理反爬机制
1. 模拟人类行为
通过增加延时、随机化请求顺序等方式模拟人类行为,可以有效规避部分反爬机制。
- 增加延时:
import time
import random
time.sleep(random.uniform(1, 3))
2. 使用验证码识别
对于需要验证码的网站,可以使用第三方验证码识别服务,如打码平台,或者使用OCR工具如Tesseract。
- 示例代码:
from PIL import Image
import pytesseract
image = Image.open('captcha.png')
text = pytesseract.image_to_string(image)
print(text)
四、管理数据存储
1. 使用数据库存储
大规模数据爬取后需要高效管理和存储数据,推荐使用关系型数据库(如MySQL)或NoSQL数据库(如MongoDB)。
-
MySQL示例:
import pymysql
connection = pymysql.connect(host='localhost',
user='user',
password='passwd',
db='db',
charset='utf8mb4')
with connection.cursor() as cursor:
sql = "INSERT INTO `table` (`title`, `link`) VALUES (%s, %s)"
cursor.execute(sql, ('title', 'http://example.com'))
connection.commit()
connection.close()
-
MongoDB示例:
from pymongo import MongoClient
client = MongoClient('localhost', 27017)
db = client['mydatabase']
collection = db['mycollection']
collection.insert_one({'title': 'title', 'link': 'http://example.com'})
2. 数据清洗与去重
确保数据的准确性和一致性,需要对爬取的数据进行清洗与去重。
-
数据清洗示例:
import pandas as pd
data = pd.read_csv('data.csv')
data.dropna(inplace=True)
-
数据去重示例:
data.drop_duplicates(subset=['link'], keep='first', inplace=True)
五、常见问题与解决方案
1. 网站反爬策略
大部分网站会有各种反爬策略,如IP封禁、验证码、动态内容加载等。针对这些问题,可以使用上述的请求头设置、代理池、模拟人类行为等方法。
2. 动态内容加载
对于使用JavaScript加载动态内容的网站,可以使用Selenium或Splash等工具来模拟浏览器行为,获取完整网页内容。
-
Selenium示例:
from selenium import webdriver
driver = webdriver.Chrome()
driver.get('http://example.com')
content = driver.page_source
driver.quit()
-
Splash示例:
import requests
splash_url = 'http://localhost:8050/render.html'
response = requests.get(splash_url, params={'url': 'http://example.com'})
print(response.text)
3. 数据量过大
当爬取的数据量过大时,可能会遇到内存不足等问题。可以通过分批次爬取、定期将数据存储到数据库等方式解决。
- 分批次爬取示例:
urls = ['http://example.com/page{}'.format(i) for i in range(1, 10001)]
for i in range(0, len(urls), 100):
batch_urls = urls[i:i+100]
# 爬取当前批次的URL
通过上述方法,可以高效地使用Python爬取上万条数据。选择合适的工具、优化代码性能、处理反爬机制、管理数据存储,都是成功的关键。希望这篇文章能够帮助你更好地理解和实施大规模数据爬取。
相关问答FAQs:
如何选择合适的Python库进行大规模数据爬取?
在进行大规模数据爬取时,选择合适的Python库是关键。常用的库包括Requests和BeautifulSoup,它们适合处理简单的网页数据提取。如果需要处理动态加载的内容,可以考虑使用Selenium或Scrapy。Scrapy是一个强大的框架,专为大规模抓取设计,支持异步请求和数据存储,非常适合需要抓取上万条数据的场景。
在爬取大量数据时,如何管理请求频率以避免被封禁?
为了避免被目标网站封禁,控制请求频率非常重要。可以通过设置请求间隔、使用代理、以及随机化请求时间来降低被检测的风险。使用time.sleep()函数可以在每次请求之间添加延迟。此外,使用旋转代理池可以帮助在多个IP地址之间分散请求,从而减少被封禁的可能性。
如何存储和处理爬取到的大量数据?
爬取到的大量数据需要有效的存储和处理方式。可以选择将数据存储在CSV文件、SQLite数据库或MongoDB等数据库中。对于结构化数据,CSV文件是一个简单易用的选择;而对于需要频繁查询和操作的数据,数据库则更为合适。在数据处理方面,可以使用Pandas库进行数据清洗和分析,提升数据的可用性和价值。
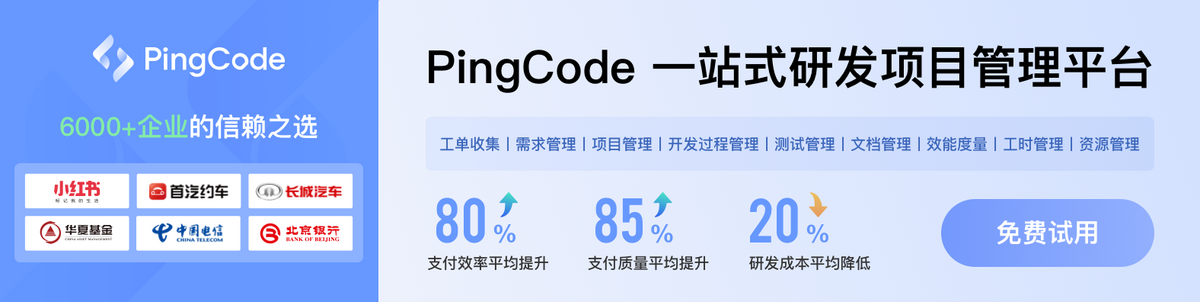