在Python中查找所有第组的字符串,可以通过正则表达式(Regular Expressions, regex)、字符串方法以及列表操作来实现。 其中,正则表达式是最强大和灵活的方法。通过使用Python的re
模块,可以实现复杂的字符串匹配和提取。以下是详细描述这一方法的步骤和示例。
一、正则表达式查找字符串
正则表达式是一种强大的工具,用于匹配字符串中的模式。Python的re
模块提供了多种方法,如findall()
, search()
, 和 match()
,可以用于查找和匹配字符串。
1、使用re.findall()查找所有匹配项
re.findall()
方法返回字符串中所有非重叠匹配项的列表。
import re
示例字符串
text = "Python is fun. Python is powerful. Python is easy to learn."
查找所有出现的 'Python'
pattern = r'Python'
matches = re.findall(pattern, text)
print(matches)
在这个示例中,re.findall()
返回所有出现的'Python',结果是一个包含所有匹配项的列表。
2、使用re.search()和re.finditer()查找第一个匹配项和所有匹配项
re.search()
方法用于查找字符串中第一个匹配项,而re.finditer()
方法返回一个迭代器,包含所有匹配项。
import re
示例字符串
text = "Python is fun. Python is powerful. Python is easy to learn."
查找第一个出现的 'Python'
pattern = r'Python'
match = re.search(pattern, text)
if match:
print(f"First match: {match.group()} at position {match.start()}")
查找所有出现的 'Python' 并打印其位置
matches = re.finditer(pattern, text)
for match in matches:
print(f"Match: {match.group()} at position {match.start()}")
二、字符串方法查找字符串
除了正则表达式,Python的字符串方法也可以用于查找子字符串。
1、使用str.find()和str.index()方法
str.find()
方法返回子字符串在字符串中的最低索引,如果没有找到则返回-1。str.index()
方法类似,但如果没有找到则会引发ValueError。
text = "Python is fun. Python is powerful. Python is easy to learn."
查找 'Python' 的所有位置
start = 0
while True:
start = text.find('Python', start)
if start == -1:
break
print(f"Found 'Python' at position {start}")
start += len('Python')
三、列表操作查找字符串
如果我们有一个字符串列表,可以使用列表操作来查找包含特定子字符串的所有元素。
1、使用列表推导查找字符串
列表推导是一种高效的方法来从列表中筛选数据。
texts = ["Python is fun", "Java is powerful", "Python is easy to learn", "C++ is complex"]
查找包含 'Python' 的所有字符串
matches = [s for s in texts if 'Python' in s]
print(matches)
四、综合示例
综合使用正则表达式、字符串方法和列表操作,创建一个完整的函数来查找字符串中的所有匹配项。
import re
def find_all_occurrences(text, pattern):
matches = re.findall(pattern, text)
return matches
def find_all_positions(text, pattern):
positions = []
start = 0
while True:
start = text.find(pattern, start)
if start == -1:
break
positions.append(start)
start += len(pattern)
return positions
def find_in_list(strings, pattern):
matches = [s for s in strings if pattern in s]
return matches
示例字符串
text = "Python is fun. Python is powerful. Python is easy to learn."
texts = ["Python is fun", "Java is powerful", "Python is easy to learn", "C++ is complex"]
使用正则表达式查找所有出现的 'Python'
print(find_all_occurrences(text, r'Python'))
使用字符串方法查找 'Python' 的所有位置
print(find_all_positions(text, 'Python'))
使用列表操作查找包含 'Python' 的所有字符串
print(find_in_list(texts, 'Python'))
结论
通过使用正则表达式、字符串方法和列表操作,Python提供了多种有效的方法来查找字符串中的所有匹配项。选择哪种方法取决于具体需求和数据的复杂性。如果需要处理复杂的匹配模式,正则表达式是最佳选择;如果只是简单的查找操作,字符串方法和列表操作也可以高效地完成任务。
相关问答FAQs:
如何在Python中查找字符串中的所有子字符串?
在Python中,可以使用字符串的find()
或index()
方法来查找子字符串的首次出现。如果需要查找所有出现的位置,可以结合while
循环和字符串切片来实现。此外,使用正则表达式的re
模块也可以有效地查找所有匹配项,方法是使用re.finditer()
。
在Python中使用正则表达式查找特定模式的字符串,该怎么做?
Python的re
模块提供了强大的功能,允许用户定义复杂的搜索模式。通过使用re.findall()
或re.finditer()
,用户可以查找所有符合特定正则表达式的字符串。这对于查找特定格式的字符串,如邮箱地址或电话号码等,非常有用。
在处理大型文本时,如何提高查找字符串的效率?
对大型文本进行字符串查找时,可以考虑使用更高效的数据结构,如字典或集合,以减少查找时间。此外,使用re
模块的编译功能可以提高效率,因为编译后的正则表达式可以重复使用。优化算法和数据结构的选择可以显著影响性能,尤其是在处理大量数据时。
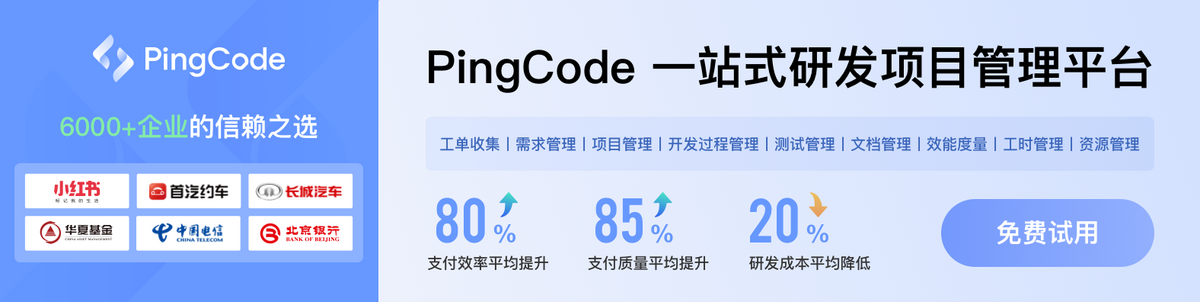