要让Python输出数字而不是表达式,可以通过使用适当的代码结构、函数和库来实现。例如,可以使用print
函数直接输出数值、使用eval
函数评估字符串表达式、或通过定义函数来处理和计算表达式。使用print
函数输出数值是最常见且简单的方法,下面将详细介绍如何实现这一点。
一、使用print
函数
在Python中,最简单和直接的方法来输出数字而不是表达式是使用print
函数。print
函数可以将结果直接输出到控制台。
number = 5
print(number) # 输出: 5
1.1 基础用法
print
函数可以输出各种数据类型,包括整数、浮点数和字符串。无论表达式多么复杂,print
函数都会计算并输出最终结果。
a = 10
b = 20
print(a + b) # 输出: 30
1.2 格式化输出
Python提供了多种格式化字符串的方法,使得输出更加美观和可读。
使用f-strings (Python 3.6及以上版本):
name = "Alice"
age = 30
print(f"{name} is {age} years old.") # 输出: Alice is 30 years old.
使用str.format()
方法:
name = "Bob"
age = 25
print("{} is {} years old.".format(name, age)) # 输出: Bob is 25 years old.
二、使用eval
函数
eval
函数可以将字符串形式的表达式评估为数值,并输出结果。这在处理用户输入或动态计算时非常有用。
expression = "3 + 4 * 2"
result = eval(expression)
print(result) # 输出: 11
2.1 安全性考虑
使用eval
函数时要注意安全性,特别是当处理不受信任的输入时。可以使用ast.literal_eval
来替代eval
,以避免安全问题。
import ast
expression = "3 + 4 * 2"
result = ast.literal_eval(expression)
print(result) # 输出: 11
三、定义函数来处理和计算表达式
通过定义函数,可以更灵活和结构化地处理和计算表达式。
def evaluate_expression(expr):
return eval(expr)
expression = "5 * (2 + 3)"
result = evaluate_expression(expression)
print(result) # 输出: 25
3.1 使用自定义函数进行复杂计算
可以定义更复杂的函数来处理特定类型的数学运算。
def calculate_area(radius):
pi = 3.141592653589793
return pi * radius * radius
radius = 5
area = calculate_area(radius)
print(f"The area of the circle is: {area}") # 输出: The area of the circle is: 78.53981633974483
四、使用Python的数学库
Python提供了丰富的数学库,如math
和numpy
,可以用来进行复杂的数学计算,并输出数值结果。
4.1 使用math
库
math
库提供了大量的数学函数,如三角函数、对数函数等。
import math
angle = math.radians(45)
sin_value = math.sin(angle)
print(f"The sine of 45 degrees is: {sin_value}") # 输出: The sine of 45 degrees is: 0.7071067811865475
4.2 使用numpy
库
numpy
库是进行科学计算的强大工具,特别适合处理大型数组和矩阵运算。
import numpy as np
array = np.array([1, 2, 3, 4, 5])
mean_value = np.mean(array)
print(f"The mean value of the array is: {mean_value}") # 输出: The mean value of the array is: 3.0
五、处理用户输入
在处理用户输入时,可以使用input
函数获取用户输入,并将其转换为数值或表达式进行计算。
user_input = input("Enter a number: ")
number = float(user_input)
print(f"You entered: {number}")
5.1 处理用户输入的表达式
通过结合input
和eval
函数,可以动态计算用户输入的表达式。
user_input = input("Enter an expression: ")
result = eval(user_input)
print(f"The result of the expression is: {result}")
六、总结
通过上述方法,可以确保Python输出的是数值而不是表达式。最常用的方法是直接使用print
函数输出计算结果,而对于更复杂的情况,可以使用eval
函数或定义自定义函数来处理表达式。使用math
和numpy
库可以方便地进行复杂的数学运算。同时,在处理用户输入时,要特别注意安全性,确保输入数据的合法性和安全性。
相关问答FAQs:
如何在Python中输出变量的值而不是表达式的字符串?
在Python中,如果你想输出变量的值而不是它的表达式,可以直接使用print()
函数。通过将变量作为参数传递给print()
,Python会输出该变量的当前值。例如,x = 5
后,使用print(x)
将输出5
。
在Python中如何控制输出的格式?
Python提供了多种格式化字符串的方法,例如f-string
、str.format()
和%
格式化。使用f-string
,你可以在字符串中直接嵌入变量,如print(f'The value is {x}')
,这样可以更灵活地控制输出格式。
如何在Python中处理多种数据类型的输出?
如果你希望在输出中包含多种数据类型,可以使用str()
函数将非字符串类型转换为字符串。比如,如果有一个整数和一个字符串,可以通过print('The number is ' + str(number) + ' and the text is ' + text)
来确保所有数据都被正确输出为字符串形式。
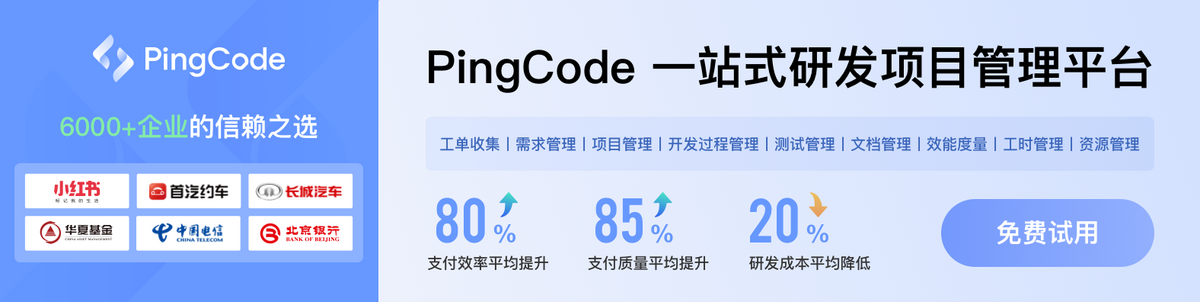