Python输出字符如何控制小数位数
在Python中控制字符的小数位数可以通过格式化字符串、使用f-string、decimal模块、round函数。其中,格式化字符串是最常用的一种方法。格式化字符串使得我们可以灵活地控制输出格式,下面将详细介绍这种方法。
在Python编程中,经常需要对浮点数进行格式化输出,特别是在涉及到金融、科学计算等领域时,对小数点后位数的控制尤为重要。为了满足这一需求,Python提供了多种方法来控制小数位数的输出。
一、格式化字符串
格式化字符串是最常用的一种方法,它可以通过指定格式来控制输出的精度。
1、旧式字符串格式化
在Python 2.x和3.x中,旧式字符串格式化使用百分号%
操作符。这种方法虽然古老,但依然在某些场景下非常有用。以下是一些示例:
value = 3.14159265
print("Formatted to 2 decimal places: %.2f" % value)
print("Formatted to 3 decimal places: %.3f" % value)
在这些示例中,%.2f
表示将浮点数格式化为小数点后2位,%.3f
表示小数点后3位。
2、新式字符串格式化
Python 3引入了新的字符串格式化方法,即str.format
。这种方法更加灵活和强大:
value = 3.14159265
print("Formatted to 2 decimal places: {:.2f}".format(value))
print("Formatted to 3 decimal places: {:.3f}".format(value))
在这些示例中,{:.2f}
表示将浮点数格式化为小数点后2位,{:.3f}
表示小数点后3位。
二、f-string格式化
Python 3.6引入了f-string(格式化字符串字面量),这是目前最推荐的字符串格式化方法。它不仅简洁,而且性能更好:
value = 3.14159265
print(f"Formatted to 2 decimal places: {value:.2f}")
print(f"Formatted to 3 decimal places: {value:.3f}")
在这些示例中,{value:.2f}
表示将浮点数格式化为小数点后2位,{value:.3f}
表示小数点后3位。
三、使用decimal模块
对于需要精确控制小数位数的场景,可以使用Python的decimal
模块。decimal
模块提供了对浮点数的精确控制,避免了浮点数运算中的精度问题:
from decimal import Decimal, ROUND_HALF_UP
value = Decimal('3.14159265')
formatted_value_2 = value.quantize(Decimal('0.00'), rounding=ROUND_HALF_UP)
formatted_value_3 = value.quantize(Decimal('0.000'), rounding=ROUND_HALF_UP)
print("Formatted to 2 decimal places:", formatted_value_2)
print("Formatted to 3 decimal places:", formatted_value_3)
在这些示例中,Decimal('0.00')
表示将浮点数格式化为小数点后2位,Decimal('0.000')
表示小数点后3位。ROUND_HALF_UP
指定了四舍五入的方式。
四、使用round函数
round
函数也是一种常用的方法,它可以对浮点数进行四舍五入操作:
value = 3.14159265
rounded_value_2 = round(value, 2)
rounded_value_3 = round(value, 3)
print("Rounded to 2 decimal places:", rounded_value_2)
print("Rounded to 3 decimal places:", rounded_value_3)
在这些示例中,round(value, 2)
表示将浮点数四舍五入到小数点后2位,round(value, 3)
表示小数点后3位。
五、结合使用多种方法
在实际应用中,常常需要结合使用多种方法来达到最佳效果。例如,结合使用f-string
和decimal
模块,可以既保持代码的简洁性,又确保结果的精确性:
from decimal import Decimal, ROUND_HALF_UP
value = Decimal('3.14159265')
formatted_value = value.quantize(Decimal('0.00'), rounding=ROUND_HALF_UP)
print(f"Formatted to 2 decimal places: {formatted_value}")
六、使用Numpy库进行科学计算
在科学计算领域,numpy
库提供了丰富的功能来控制小数位数的输出:
import numpy as np
value = np.pi
formatted_value_2 = np.round(value, 2)
formatted_value_3 = np.round(value, 3)
print("Numpy rounded to 2 decimal places:", formatted_value_2)
print("Numpy rounded to 3 decimal places:", formatted_value_3)
在这些示例中,np.round(value, 2)
表示将浮点数四舍五入到小数点后2位,np.round(value, 3)
表示小数点后3位。
七、使用Pandas库进行数据分析
在数据分析领域,pandas
库提供了许多功能来控制小数位数的输出,特别是在处理数据框时:
import pandas as pd
data = {'value': [3.14159265, 2.71828182, 1.61803398]}
df = pd.DataFrame(data)
df['formatted_value_2'] = df['value'].round(2)
df['formatted_value_3'] = df['value'].round(3)
print("Pandas DataFrame:")
print(df)
在这些示例中,df['value'].round(2)
表示将数据框中的浮点数四舍五入到小数点后2位,df['value'].round(3)
表示小数点后3位。
八、实战应用场景
1、金融领域
在金融领域,精确的小数位数控制至关重要。例如,在股票交易中,价格通常需要保留到小数点后两位:
stock_price = 123.456789
formatted_price = f"${stock_price:.2f}"
print("Stock price:", formatted_price)
在这个示例中,{stock_price:.2f}
表示将股票价格格式化为小数点后2位。
2、科学计算
在科学计算领域,结果的精度非常重要。例如,在物理实验中,测量数据通常需要保留到小数点后若干位:
measurement = 0.123456789
formatted_measurement = f"{measurement:.5f}"
print("Measurement:", formatted_measurement)
在这个示例中,{measurement:.5f}
表示将测量数据格式化为小数点后5位。
3、报表生成
在生成报表时,通常需要对数据进行格式化以便于阅读。例如,在生成财务报表时,金额需要保留到小数点后两位:
revenue = 987654.321
formatted_revenue = f"${revenue:,.2f}"
print("Revenue:", formatted_revenue)
在这个示例中,{revenue:,.2f}
表示将金额格式化为千分位分隔符和小数点后2位。
九、总结
在Python中控制字符的小数位数有多种方法,包括旧式字符串格式化、新式字符串格式化、f-string、decimal模块、round函数、numpy库和pandas库等。在不同的应用场景中,可以选择最合适的方法来确保结果的精度和代码的简洁性。
通过本文的详细介绍,相信读者已经掌握了多种控制小数位数的方法,并能够在实际项目中灵活应用这些方法。无论是在金融、科学计算还是数据分析领域,精确控制小数位数的输出都是一项基本且重要的技能。
相关问答FAQs:
如何在Python中格式化浮点数的输出?
在Python中,可以使用format()
函数或f-string来格式化浮点数的输出。例如,使用"{:.2f}".format(value)
可以将浮点数格式化为保留两位小数的字符串。在使用f-string时,语法为f"{value:.2f}"
。这样可以确保输出的数字在小数点后有固定的位数。
如果我只想在输出时控制小数位数,而不改变原始数据,应该怎么做?
可以通过格式化输出的方式来实现。使用format()
或f-string只会影响输出的结果,而不会改变原始的浮点数值。例如,value = 3.14159
,使用print("{:.2f}".format(value))
仅在打印时显示为3.14,原始数据仍保持为3.14159。
在Python中如何处理小数位数的四舍五入?
在进行小数位数的控制时,Python会自动执行四舍五入。例如,print("{:.2f}".format(2.675))
的输出将是2.68,因为Python在输出时会根据最后一位数字进行四舍五入。如果需要进行特定的四舍五入操作,可以使用round()
函数,round(value, 2)
会将数值四舍五入到小数点后两位。
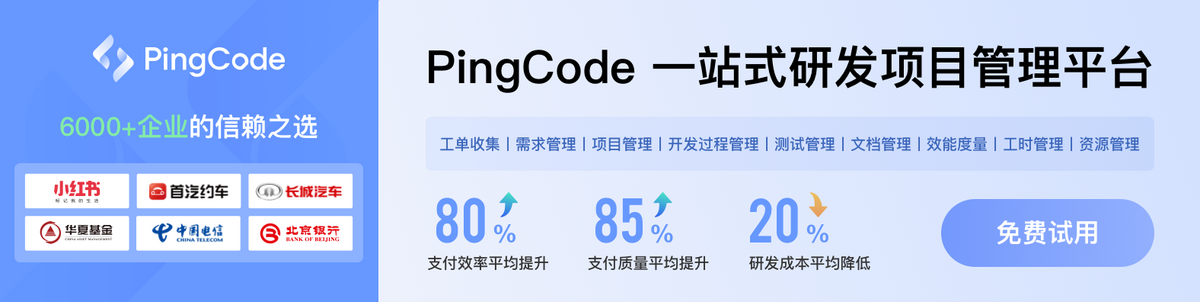