在Python中,停止当前线程的方式有多种,包括使用线程标志、线程池的管理、以及信号处理等方法。以下是详细的介绍:
使用线程标志、线程池的管理、信号处理是停止线程的常见方法。线程标志是一种简单而有效的方式,通过设置一个共享的标志位,线程在每次循环中检查该标志位来决定是否终止。线程池的管理则更为高级,通过concurrent.futures
模块,可以方便地管理多个线程,并在需要时取消未完成的任务。信号处理主要用于在需要处理外部中断信号时,通过捕获信号来终止线程。下面我们将详细探讨每种方法。
一、使用线程标志
使用线程标志是一种简单且灵活的方式来控制线程的终止。通过设置一个全局变量,线程在每次循环中检查这个变量的状态,如果发现变量指示需要终止,线程就会自行退出。
1.1 创建线程标志
首先,我们需要创建一个全局变量作为线程标志,这个标志将在多个线程之间共享。
import threading
import time
创建全局标志
stop_threads = False
def worker():
while not stop_threads:
print("Thread is running")
time.sleep(1)
启动线程
thread = threading.Thread(target=worker)
thread.start()
停止线程
time.sleep(5)
stop_threads = True
thread.join()
print("Thread has been stopped")
在这个例子中,线程每秒打印一次消息,并在5秒后通过设置stop_threads
标志来停止线程。
1.2 优化线程标志
为了提高线程的响应速度,我们可以使用线程事件来替代简单的布尔变量。threading.Event
提供了更高级的控制方式。
import threading
import time
创建线程事件
stop_event = threading.Event()
def worker():
while not stop_event.is_set():
print("Thread is running")
time.sleep(1)
启动线程
thread = threading.Thread(target=worker)
thread.start()
停止线程
time.sleep(5)
stop_event.set()
thread.join()
print("Thread has been stopped")
在这个优化后的例子中,线程通过检查stop_event
的状态来决定是否继续运行,从而可以更快速地响应终止请求。
二、使用线程池管理
通过concurrent.futures
模块,我们可以更方便地管理多个线程的生命周期,并在需要时取消未完成的任务。
2.1 创建线程池
首先,我们需要创建一个线程池,并提交多个任务。
from concurrent.futures import ThreadPoolExecutor
import time
def worker():
while True:
print("Thread is running")
time.sleep(1)
创建线程池
with ThreadPoolExecutor(max_workers=2) as executor:
future = executor.submit(worker)
time.sleep(5)
future.cancel()
print("Thread has been cancelled")
在这个例子中,我们创建了一个包含两个线程的线程池,并提交了一个无限循环的任务。5秒后,我们通过future.cancel()
方法来取消任务。
2.2 检查任务状态
为了确保任务已经被成功取消,我们可以检查任务的状态。
from concurrent.futures import ThreadPoolExecutor, as_completed
import time
def worker():
while True:
print("Thread is running")
time.sleep(1)
创建线程池
with ThreadPoolExecutor(max_workers=2) as executor:
future = executor.submit(worker)
time.sleep(5)
future.cancel()
# 检查任务状态
if future.cancelled():
print("Thread has been successfully cancelled")
else:
print("Thread cancellation failed")
在这个优化后的例子中,我们通过future.cancelled()
方法来检查任务是否已经被成功取消,并输出相应的消息。
三、使用信号处理
在某些情况下,我们需要处理外部中断信号来终止线程。通过捕获信号,我们可以在接收到中断信号时执行特定的操作,如终止线程。
3.1 捕获信号
首先,我们需要导入signal
模块,并定义信号处理函数。
import signal
import threading
import time
def signal_handler(sig, frame):
global stop_threads
stop_threads = True
print("Signal received, stopping threads")
signal.signal(signal.SIGINT, signal_handler)
创建全局标志
stop_threads = False
def worker():
while not stop_threads:
print("Thread is running")
time.sleep(1)
启动线程
thread = threading.Thread(target=worker)
thread.start()
等待线程终止
thread.join()
print("Thread has been stopped")
在这个例子中,我们定义了一个信号处理函数signal_handler
,当接收到SIGINT
信号(通常由Ctrl+C产生)时,设置stop_threads
标志并打印消息。线程在每次循环中检查stop_threads
标志,如果发现需要终止,线程就会自行退出。
3.2 处理多个信号
如果需要处理多个信号,我们可以为每个信号注册不同的处理函数。
import signal
import threading
import time
def signal_handler(sig, frame):
global stop_threads
stop_threads = True
print(f"Signal {sig} received, stopping threads")
signal.signal(signal.SIGINT, signal_handler)
signal.signal(signal.SIGTERM, signal_handler)
创建全局标志
stop_threads = False
def worker():
while not stop_threads:
print("Thread is running")
time.sleep(1)
启动线程
thread = threading.Thread(target=worker)
thread.start()
等待线程终止
thread.join()
print("Thread has been stopped")
在这个优化后的例子中,我们为SIGINT
和SIGTERM
信号都注册了相同的处理函数signal_handler
,当接收到任一信号时,设置stop_threads
标志并打印消息。线程在每次循环中检查stop_threads
标志,如果发现需要终止,线程就会自行退出。
四、总结
在Python中,停止当前线程的方式有多种,包括使用线程标志、线程池的管理、以及信号处理等方法。每种方法都有其优缺点,选择适合的方式取决于具体的应用场景和需求。
使用线程标志是一种简单而有效的方式,通过设置一个共享的标志位,线程在每次循环中检查该标志位来决定是否终止。线程池的管理则更为高级,通过concurrent.futures
模块,可以方便地管理多个线程,并在需要时取消未完成的任务。信号处理主要用于在需要处理外部中断信号时,通过捕获信号来终止线程。
通过以上介绍,我们可以根据具体需求选择合适的线程终止方式,从而更有效地管理线程的生命周期。
相关问答FAQs:
如何在Python中安全地停止当前线程?
在Python中,直接停止一个线程并不是一个推荐的做法。为了安全地停止线程,通常可以使用一个标志位(如一个全局变量或线程安全的对象)来控制线程的运行状态。线程在执行过程中定期检查这个标志位,如果检测到需要停止,就可以安全地结束自己的任务。
使用线程的join方法能否停止线程?join
方法并不直接停止线程,而是让调用线程等待目标线程执行完毕。如果想要停止线程,建议结合使用标志位来控制线程的循环,使其能够在适当的时机退出。
Python中是否有专门的库来管理线程的停止?
虽然Python标准库没有提供直接停止线程的功能,但可以使用threading
模块中的Event
对象来实现线程的控制。通过设置事件标志,线程可以在适当的时机检查事件状态并安全退出。这种方式更加优雅且符合Python的设计理念。
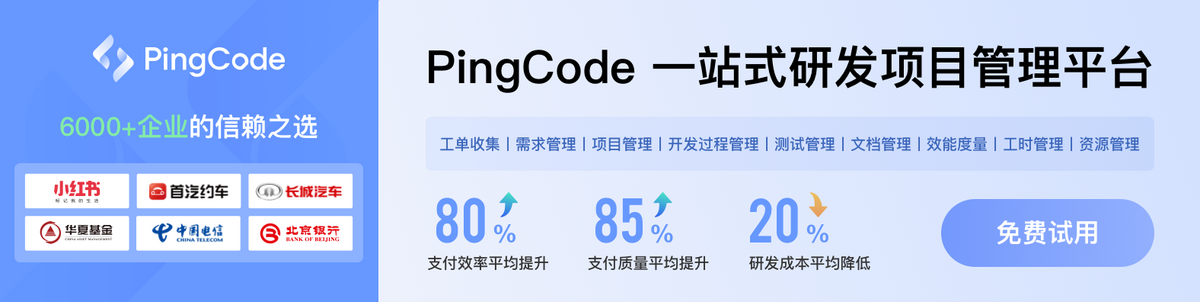