如何用Python做一个群发器
在使用Python制作一个群发器时,可以用Python的强大功能和丰富的库来实现。使用Selenium进行自动化操作、利用SMTP库发送电子邮件、使用Twilio发送短信,这些都是有效的方法。本文将详细介绍如何使用这些工具和方法来创建一个功能强大的群发器,并着重讲解Selenium自动化操作,帮助你轻松应对各种群发需求。
一、使用Selenium进行自动化操作
Selenium是一个强大的工具,用于自动化测试Web应用程序。它可以模拟用户的操作,比如填写表单、点击按钮等,非常适合用于群发信息的场景。
1、安装Selenium
在开始使用Selenium之前,你需要先安装它。你可以通过pip来安装Selenium:
pip install selenium
此外,你还需要下载对应的WebDriver,比如ChromeDriver或GeckoDriver,用于驱动浏览器。
2、设置WebDriver
以下是一个简单的示例,展示如何使用Selenium来启动一个Chrome浏览器并打开一个网页:
from selenium import webdriver
from selenium.webdriver.common.keys import Keys
设置WebDriver
driver = webdriver.Chrome(executable_path='path/to/chromedriver')
打开网页
driver.get("http://www.example.com")
找到元素并进行操作
element = driver.find_element_by_name("q")
element.send_keys("Hello, world!")
element.send_keys(Keys.RETURN)
3、模拟用户操作
为了实现群发功能,你需要根据实际需求来模拟用户操作。比如,登录某个社交媒体平台,找到发送消息的按钮,然后循环遍历联系人并发送消息。以下是一个简化的示例:
from selenium.webdriver.common.by import By
import time
假设已经登录到某个社交媒体平台
driver.get("http://www.socialmedia.com")
找到联系人列表
contacts = driver.find_elements(By.CLASS_NAME, "contact")
遍历联系人并发送消息
for contact in contacts:
contact.click()
time.sleep(1)
message_box = driver.find_element(By.CLASS_NAME, "message-box")
message_box.send_keys("Hello, this is a test message!")
send_button = driver.find_element(By.CLASS_NAME, "send-button")
send_button.click()
time.sleep(1)
以上代码只是一个简化的示例,实际操作中需要根据具体的网页结构进行调整。
二、利用SMTP库发送电子邮件
SMTP(Simple Mail Transfer Protocol)是发送电子邮件的标准协议。Python内置的smtplib
库使得发送电子邮件变得非常简单。
1、配置SMTP服务器
首先,你需要配置SMTP服务器信息,比如Gmail、Outlook等。以下是一个示例,展示如何使用Gmail的SMTP服务器发送电子邮件:
import smtplib
from email.mime.text import MIMEText
from email.mime.multipart import MIMEMultipart
配置SMTP服务器
smtp_server = "smtp.gmail.com"
smtp_port = 587
smtp_user = "your_email@gmail.com"
smtp_password = "your_password"
创建SMTP对象
server = smtplib.SMTP(smtp_server, smtp_port)
server.starttls()
server.login(smtp_user, smtp_password)
2、创建和发送电子邮件
接下来,创建一个电子邮件并发送:
# 创建邮件内容
msg = MIMEMultipart()
msg['From'] = smtp_user
msg['To'] = "recipient@example.com"
msg['Subject'] = "Test Email"
body = "This is a test email sent from Python."
msg.attach(MIMEText(body, 'plain'))
发送邮件
text = msg.as_string()
server.sendmail(smtp_user, "recipient@example.com", text)
关闭连接
server.quit()
3、群发邮件
为了实现群发功能,你只需将接收者列表进行遍历,并发送邮件:
recipients = ["recipient1@example.com", "recipient2@example.com", "recipient3@example.com"]
for recipient in recipients:
msg['To'] = recipient
text = msg.as_string()
server.sendmail(smtp_user, recipient, text)
三、使用Twilio发送短信
Twilio是一个强大的云通信平台,提供短信、语音和其他通信服务。你可以利用Twilio的API来实现短信群发功能。
1、安装Twilio库
首先,你需要安装Twilio库:
pip install twilio
2、配置Twilio客户端
接下来,配置Twilio客户端并发送短信:
from twilio.rest import Client
Twilio账户信息
account_sid = "your_account_sid"
auth_token = "your_auth_token"
twilio_number = "+1234567890"
client = Client(account_sid, auth_token)
发送短信
message = client.messages.create(
to="+0987654321",
from_=twilio_number,
body="Hello, this is a test message from Python!"
)
3、群发短信
与电子邮件类似,你只需将接收者列表进行遍历,并发送短信:
recipients = ["+0987654321", "+1123456789", "+1234567890"]
for recipient in recipients:
message = client.messages.create(
to=recipient,
from_=twilio_number,
body="Hello, this is a test message from Python!"
)
四、综合应用实例
为了将上述方法综合应用,以下是一个完整的示例,展示如何使用Selenium、SMTP和Twilio来实现一个多功能的群发器。
1、导入必要的库
from selenium import webdriver
from selenium.webdriver.common.keys import Keys
from selenium.webdriver.common.by import By
import smtplib
from email.mime.text import MIMEText
from email.mime.multipart import MIMEMultipart
from twilio.rest import Client
import time
2、定义发送邮件的函数
def send_email(smtp_user, smtp_password, recipients, subject, body):
smtp_server = "smtp.gmail.com"
smtp_port = 587
server = smtplib.SMTP(smtp_server, smtp_port)
server.starttls()
server.login(smtp_user, smtp_password)
msg = MIMEMultipart()
msg['From'] = smtp_user
msg['Subject'] = subject
msg.attach(MIMEText(body, 'plain'))
for recipient in recipients:
msg['To'] = recipient
text = msg.as_string()
server.sendmail(smtp_user, recipient, text)
server.quit()
3、定义发送短信的函数
def send_sms(account_sid, auth_token, twilio_number, recipients, body):
client = Client(account_sid, auth_token)
for recipient in recipients:
message = client.messages.create(
to=recipient,
from_=twilio_number,
body=body
)
4、定义使用Selenium发送消息的函数
def send_web_message(driver_path, url, contacts_class, message_box_class, send_button_class, message):
driver = webdriver.Chrome(executable_path=driver_path)
driver.get(url)
contacts = driver.find_elements(By.CLASS_NAME, contacts_class)
for contact in contacts:
contact.click()
time.sleep(1)
message_box = driver.find_element(By.CLASS_NAME, message_box_class)
message_box.send_keys(message)
send_button = driver.find_element(By.CLASS_NAME, send_button_class)
send_button.click()
time.sleep(1)
driver.quit()
5、整合所有功能
if __name__ == "__main__":
smtp_user = "your_email@gmail.com"
smtp_password = "your_password"
email_recipients = ["recipient1@example.com", "recipient2@example.com"]
email_subject = "Test Email"
email_body = "This is a test email sent from Python."
account_sid = "your_account_sid"
auth_token = "your_auth_token"
twilio_number = "+1234567890"
sms_recipients = ["+0987654321", "+1123456789"]
sms_body = "Hello, this is a test message from Python!"
driver_path = 'path/to/chromedriver'
web_url = "http://www.socialmedia.com"
contacts_class = "contact"
message_box_class = "message-box"
send_button_class = "send-button"
web_message = "Hello, this is a test message!"
send_email(smtp_user, smtp_password, email_recipients, email_subject, email_body)
send_sms(account_sid, auth_token, twilio_number, sms_recipients, sms_body)
send_web_message(driver_path, web_url, contacts_class, message_box_class, send_button_class, web_message)
结论
使用Python制作一个群发器并不难,关键在于选择适合自己的工具和方法。Selenium的强大自动化功能、SMTP库的邮件发送能力、Twilio的短信服务,这些都可以帮助你实现高效的群发功能。希望本文的内容能够帮助你更好地理解和应用这些工具,轻松应对各种群发需求。
相关问答FAQs:
群发器是如何工作的?
群发器通过将信息发送到多个接收者来实现高效的沟通。在Python中,可以使用各种库(如smtplib、requests等)来构建群发器,以便通过电子邮件、短信或社交媒体平台同时发送消息。具体实现需要掌握Python的基本语法和相应的API接口。
我需要哪些Python库来创建群发器?
常用的库包括smtplib(用于发送电子邮件)、requests(用于发送HTTP请求)、以及第三方库如Twilio(用于发送短信)。根据你选择的消息发送方式,可能还需要其他库。了解这些库的功能和使用方法将帮助你更好地构建群发器。
如何确保我的群发器不会被标记为垃圾邮件?
为了避免被标记为垃圾邮件,建议遵循一些最佳实践,例如:使用合法的联系人列表、避免使用误导性标题、合理安排发送频率等。此外,提供退订选项也可以增加用户信任,从而降低被标记的风险。确保遵循相关法规(如GDPR或CAN-SPAM法案)也是非常重要的。
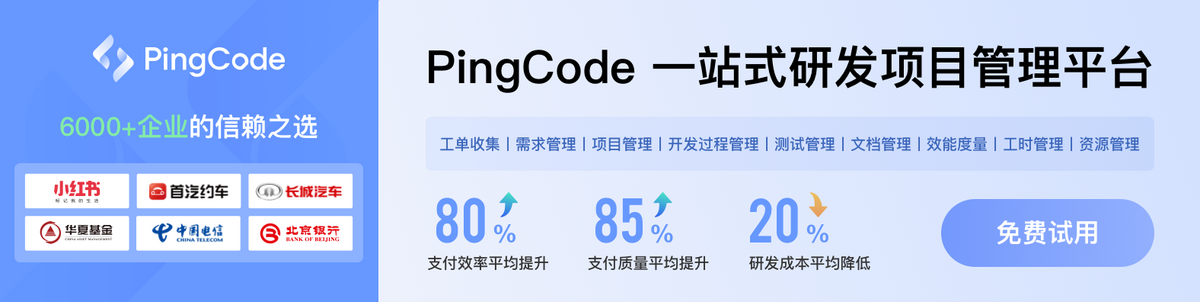