在Python中,提取字符串的子串可以通过多种方法实现,如切片、正则表达式、内置字符串方法等。其中,切片是最常用和直观的方法。通过切片,你可以轻松获取字符串的任意部分。以下将详细介绍如何使用这些方法来提取字符串的子串,并给出具体的代码示例。
一、使用切片提取字符串子串
切片是Python中最常见的字符串操作方法之一。它允许你通过指定开始和结束索引来获取字符串的特定部分。
1.1 基本切片操作
在Python中,切片操作符[:]
用于提取字符串的子串。
text = "Hello, World!"
sub_str = text[0:5]
print(sub_str) # 输出: Hello
1.2 省略起始或结束索引
你可以省略起始或结束索引,表示从开头或一直到结尾。
text = "Hello, World!"
sub_str = text[:5] # 从开头到索引5
print(sub_str) # 输出: Hello
sub_str = text[7:] # 从索引7到结尾
print(sub_str) # 输出: World!
1.3 使用负索引
负索引表示从字符串末尾开始计数。
text = "Hello, World!"
sub_str = text[-6:-1]
print(sub_str) # 输出: World
1.4 步长参数
切片还可以指定步长参数[start:end:step]
,用于按指定步长提取子串。
text = "Hello, World!"
sub_str = text[::2]
print(sub_str) # 输出: Hlo ol!
二、使用内置字符串方法
Python提供了一些内置字符串方法,如find()
、index()
等,可以用于提取子串。
2.1 使用find()方法
find()
方法返回子串在字符串中的首次出现位置。如果子串不存在,则返回-1。
text = "Hello, World!"
position = text.find("World")
if position != -1:
sub_str = text[position:position+len("World")]
print(sub_str) # 输出: World
2.2 使用index()方法
index()
方法与find()
类似,但如果子串不存在,会引发ValueError
。
text = "Hello, World!"
try:
position = text.index("World")
sub_str = text[position:position+len("World")]
print(sub_str) # 输出: World
except ValueError:
print("子串未找到")
三、使用正则表达式提取子串
正则表达式是一种强大的字符串操作工具,适用于复杂的字符串匹配和提取任务。Python的re
模块提供了丰富的正则表达式支持。
3.1 基本正则表达式匹配
使用re.search()
函数来查找子串。
import re
text = "Hello, World!"
match = re.search(r"World", text)
if match:
print(match.group()) # 输出: World
3.2 捕获组
正则表达式的捕获组可以提取特定模式的子串。
import re
text = "Hello, World!"
match = re.search(r"(World)", text)
if match:
print(match.group(1)) # 输出: World
3.3 查找所有匹配
使用re.findall()
来查找所有匹配的子串。
import re
text = "Hello, World! Welcome to the World of Python."
matches = re.findall(r"World", text)
print(matches) # 输出: ['World', 'World']
四、使用分割和连接方法
你可以使用字符串的分割和连接方法来提取子串。
4.1 使用split()方法
split()
方法根据指定分隔符将字符串拆分成列表,然后你可以根据需要提取子串。
text = "Hello, World!"
parts = text.split(", ")
print(parts) # 输出: ['Hello', 'World!']
sub_str = parts[1]
print(sub_str) # 输出: World!
4.2 使用join()方法
join()
方法用于连接字符串列表,可以用于重新组合子串。
text = "Hello, World!"
parts = text.split(", ")
sub_str = ", ".join(parts[:2])
print(sub_str) # 输出: Hello, World!
五、使用字符串模板
Python的字符串模板可以用于提取和替换子串。
5.1 使用format()方法
format()
方法可以通过占位符提取子串。
text = "Hello, {}!"
sub_str = text.format("World")
print(sub_str) # 输出: Hello, World!
5.2 使用f-strings
Python 3.6引入的f-strings提供了一种简洁的字符串格式化方法。
name = "World"
text = f"Hello, {name}!"
print(text) # 输出: Hello, World!
六、总结
通过以上方法,你可以在Python中灵活地提取字符串的子串。切片是最常用的方法,适合处理简单的字符串提取任务。内置字符串方法如find()
和index()
提供了更多的控制选项。正则表达式适用于复杂的字符串匹配和提取任务,而分割和连接方法以及字符串模板提供了额外的灵活性。根据具体需求选择合适的方法,能够帮助你更高效地处理字符串操作任务。
相关问答FAQs:
如何在Python中提取字符串中的特定子串?
在Python中,可以使用字符串的内置方法来提取特定的子串。例如,使用切片(slice)可以轻松获取字符串的某个部分。若要提取特定字符,可以结合使用find()
、index()
等方法来定位子串的起始和结束位置,之后通过切片获取需要的部分。
有哪些常用的方法可以提取字符串中的内容?
Python提供多种方法来提取字符串中的内容,包括split()
、join()
、replace()
和正则表达式模块re
。使用split()
可以根据指定分隔符将字符串切割成多个部分,而re
模块则允许更复杂的模式匹配,适合提取结构化信息,如邮箱地址或电话号码。
如何使用正则表达式提取字符串中的信息?
使用Python的re
模块,您可以通过编写正则表达式来匹配和提取字符串中的特定模式。例如,re.findall()
可以返回所有匹配项的列表,而re.search()
可以找到第一个匹配并返回一个匹配对象。通过这些工具,可以灵活地提取复杂的字符串信息。
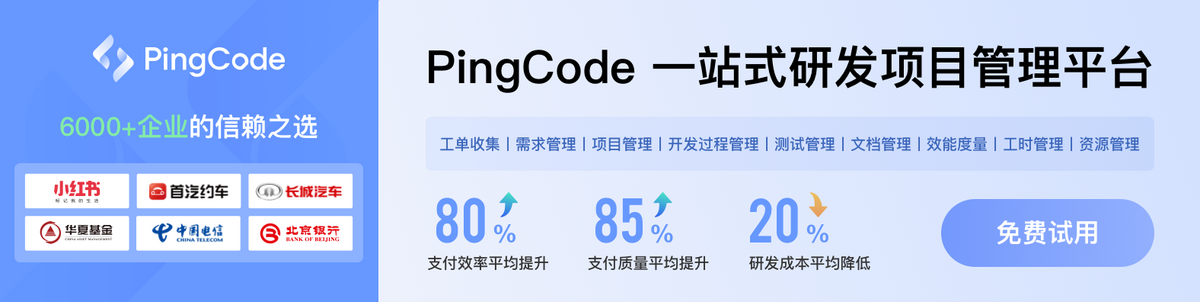