Python提取CSV文件最后一行数据的方法有多种,常见的包括:使用pandas库、使用csv模块、直接读取文件。其中,pandas库是最推荐的方法,因为它提供了强大的数据处理功能和简洁的API。接下来我们详细探讨使用pandas库的方法。
使用pandas库读取CSV文件的最后一行数据步骤如下:
- 安装pandas库
- 读取CSV文件
- 提取最后一行数据
import pandas as pd
读取CSV文件
df = pd.read_csv('your_file.csv')
提取最后一行数据
last_row = df.tail(1)
print(last_row)
一、PANDAS库的安装与使用
1. 安装pandas库
要使用pandas库,我们首先需要安装它。可以使用pip命令来安装:
pip install pandas
2. 读取CSV文件
pandas库提供了read_csv
函数,可以方便地读取CSV文件,并将其转换为DataFrame对象。这一步是所有后续操作的基础。
import pandas as pd
读取CSV文件
df = pd.read_csv('your_file.csv')
3. 提取最后一行数据
pandas库提供了tail
函数,可以用来提取最后n行数据。对于只需要最后一行数据的情况,我们可以使用tail(1)
:
last_row = df.tail(1)
print(last_row)
二、CSV模块的使用
1. 读取CSV文件
Python内置的csv模块也可以用来读取CSV文件。我们可以逐行读取文件,然后存储最后一行数据。
import csv
打开CSV文件
with open('your_file.csv', mode='r') as file:
reader = csv.reader(file)
last_row = None
# 逐行读取文件
for row in reader:
last_row = row
print(last_row)
2. 优化读取性能
对于大文件,逐行读取文件可能会比较慢。我们可以通过倒序读取文件来提高性能。以下是使用seek
和tell
方法优化读取性能的示例:
import csv
def read_last_line(file):
file.seek(0, 2) # 移动文件指针到文件末尾
position = file.tell()
while position >= 0:
file.seek(position)
next_char = file.read(1)
if next_char == '\n' and position != file.tell() - 1:
return file.readline()
position -= 1
file.seek(0)
return file.readline()
with open('your_file.csv', mode='r') as file:
last_line = read_last_line(file)
last_row = list(csv.reader([last_line]))[0]
print(last_row)
三、直接读取文件
1. 读取整个文件
我们还可以直接读取文件的所有内容,然后提取最后一行数据。这种方法适用于小文件。
# 读取文件的所有内容
with open('your_file.csv', mode='r') as file:
lines = file.readlines()
last_line = lines[-1].strip()
print(last_line)
2. 读取文件的最后几行
对于大文件,读取整个文件可能会占用大量内存。我们可以通过读取文件的最后几行来优化性能。
def read_last_lines(file_path, num_lines):
with open(file_path, 'rb') as file:
file.seek(0, 2)
buffer = bytearray()
position = file.tell()
while position >= 0 and len(buffer) < num_lines:
file.seek(position)
next_char = file.read(1)
buffer.extend(next_char)
if next_char == b'\n':
num_lines -= 1
position -= 1
buffer.reverse()
return buffer.decode().split('\n')[-1]
last_line = read_last_lines('your_file.csv', 1)
print(last_line)
四、综合对比与应用场景
1. 使用场景
- Pandas库:适用于需要进行复杂数据处理和分析的场景,推荐用于大多数数据处理任务。
- CSV模块:适用于简单的CSV文件读取任务,不需要额外安装库。
- 直接读取文件:适用于需要快速读取文件最后几行数据的场景,特别是大文件的处理。
2. 性能比较
- Pandas库:功能强大,但可能占用较多内存。
- CSV模块:性能中等,适合小文件和简单任务。
- 直接读取文件:性能较好,适合大文件的快速读取。
总结
在Python中提取CSV文件最后一行数据的方法有多种,选择合适的方法取决于具体应用场景。Pandas库是最推荐的方法,因为它提供了强大的数据处理功能和简洁的API。对于大文件的处理,可以考虑使用直接读取文件的方法,以提高性能。通过合理选择方法,可以有效提高数据处理的效率。
相关问答FAQs:
如何使用Python读取CSV文件中的最后一行数据?
可以使用Python的内置csv模块或pandas库来读取CSV文件。使用csv模块时,可以逐行读取文件并存储最后一行的数据。使用pandas库则可以更简便地通过iloc
方法提取最后一行。例如,使用pandas的代码如下:
import pandas as pd
data = pd.read_csv('your_file.csv')
last_row = data.iloc[-1]
print(last_row)
在提取CSV最后一行数据时,如何处理大文件以提高效率?
对于大型CSV文件,逐行读取可能会导致性能问题。可以考虑使用pandas的read_csv
函数中的chunksize
参数,以分块读取文件,从而减少内存占用。同时,利用tail
方法可以直接获取最后几行数据,优化性能。例如:
for chunk in pd.read_csv('your_large_file.csv', chunksize=10000):
last_chunk = chunk
last_row = last_chunk.tail(1)
print(last_row)
如果CSV文件为空,如何确保代码不会报错?
在处理CSV文件时,确保文件非空是很重要的。可以在读取文件之前先进行检查,判断文件是否有内容。使用以下代码可以有效避免空文件导致的错误:
import os
import pandas as pd
file_path = 'your_file.csv'
if os.path.getsize(file_path) > 0:
data = pd.read_csv(file_path)
last_row = data.iloc[-1]
print(last_row)
else:
print("CSV文件为空。")
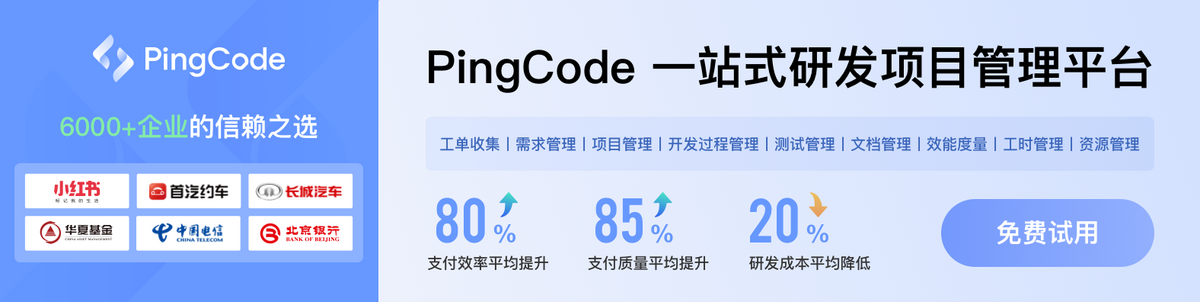