在Python中,使用适当的库和方法可以实现逐一下载文件。核心步骤包括:使用requests
库下载文件、处理文件保存、使用循环逐一下载多个文件。以下将详细描述如何实现这些步骤。
一、安装和导入必要的库
在进行文件下载之前,首先需要确保已经安装了所需的库。常用的库包括requests
和os
。requests
库用于处理HTTP请求,而os
库用于处理文件和目录操作。
import requests
import os
如果你尚未安装这些库,可以使用以下命令来安装:
pip install requests
二、创建文件保存目录
在下载文件之前,通常需要指定一个保存文件的目录。如果目录不存在,需要先创建它。使用os
库可以轻松实现这一点:
def create_directory(directory):
if not os.path.exists(directory):
os.makedirs(directory)
三、下载单个文件
使用requests
库的get
方法可以下载单个文件,并通过文件流保存到本地。以下是一个示例函数,用于下载单个文件:
def download_file(url, save_path):
response = requests.get(url, stream=True)
with open(save_path, 'wb') as file:
for chunk in response.iter_content(chunk_size=8192):
if chunk:
file.write(chunk)
四、逐一下载多个文件
在下载多个文件时,可以使用循环遍历一个包含文件URL的列表,并调用之前定义的download_file
函数来逐一下载每个文件。
def download_files(url_list, save_directory):
create_directory(save_directory)
for url in url_list:
file_name = os.path.basename(url)
save_path = os.path.join(save_directory, file_name)
download_file(url, save_path)
print(f"Downloaded: {file_name}")
五、示例代码
以下是一个完整的示例代码,展示了如何逐一下载多个文件并保存到指定目录:
import requests
import os
def create_directory(directory):
if not os.path.exists(directory):
os.makedirs(directory)
def download_file(url, save_path):
response = requests.get(url, stream=True)
with open(save_path, 'wb') as file:
for chunk in response.iter_content(chunk_size=8192):
if chunk:
file.write(chunk)
def download_files(url_list, save_directory):
create_directory(save_directory)
for url in url_list:
file_name = os.path.basename(url)
save_path = os.path.join(save_directory, file_name)
download_file(url, save_path)
print(f"Downloaded: {file_name}")
示例URL列表
url_list = [
"https://example.com/file1.zip",
"https://example.com/file2.zip",
"https://example.com/file3.zip"
]
保存目录
save_directory = "downloads"
逐一下载文件
download_files(url_list, save_directory)
六、处理异常情况
在实际应用中,下载过程可能会遇到各种异常情况,例如网络问题、文件不存在等。为了提高代码的鲁棒性,可以添加异常处理:
def download_file(url, save_path):
try:
response = requests.get(url, stream=True)
response.raise_for_status()
with open(save_path, 'wb') as file:
for chunk in response.iter_content(chunk_size=8192):
if chunk:
file.write(chunk)
except requests.exceptions.RequestException as e:
print(f"Failed to download {url}: {e}")
七、并发下载(可选)
对于需要下载大量文件的情况,可以考虑使用并发下载来提高效率。Python提供了多线程和多进程的支持,可以使用concurrent.futures
库实现并发下载:
import concurrent.futures
def download_files_concurrently(url_list, save_directory):
create_directory(save_directory)
with concurrent.futures.ThreadPoolExecutor(max_workers=5) as executor:
futures = []
for url in url_list:
file_name = os.path.basename(url)
save_path = os.path.join(save_directory, file_name)
futures.append(executor.submit(download_file, url, save_path))
for future in concurrent.futures.as_completed(futures):
try:
future.result()
except Exception as e:
print(f"Download failed: {e}")
使用并发下载
download_files_concurrently(url_list, save_directory)
通过以上步骤,你可以使用Python逐一下载文件,并且可以根据需要扩展到并发下载,提高效率。
相关问答FAQs:
如何使用Python实现逐个文件下载?
要逐个下载文件,您可以使用Python的requests库结合循环来处理每个文件的下载。首先,确保您已安装requests库。然后,可以通过以下步骤实现逐个下载:定义文件的URL列表,使用for循环遍历这些URL,并在每次迭代中下载文件。代码示例如下:
import requests
urls = ['http://example.com/file1.jpg', 'http://example.com/file2.jpg'] # 文件链接列表
for url in urls:
response = requests.get(url)
with open(url.split('/')[-1], 'wb') as file:
file.write(response.content)
如果下载的文件很大,怎样保证下载的稳定性?
对于大文件下载,可以通过设置流下载来提高稳定性。使用stream=True
参数,使得文件内容逐步写入到本地。这样可以避免内存占用过高,代码示例如下:
response = requests.get(url, stream=True)
with open('filename', 'wb') as file:
for chunk in response.iter_content(chunk_size=8192):
file.write(chunk)
如何处理下载过程中的异常情况?
在下载文件时,异常情况时有发生。例如网络中断或URL无效。使用try-except块可以有效捕获异常,并采取相应措施,比如重试下载或记录错误信息。下面是一个简单的异常处理示例:
try:
response = requests.get(url)
response.raise_for_status() # 检查请求是否成功
with open('filename', 'wb') as file:
file.write(response.content)
except requests.exceptions.RequestException as e:
print(f"下载失败: {e}")
通过这些方法,您可以在Python中实现逐个下载文件,同时确保下载过程的稳定性和异常处理能力。
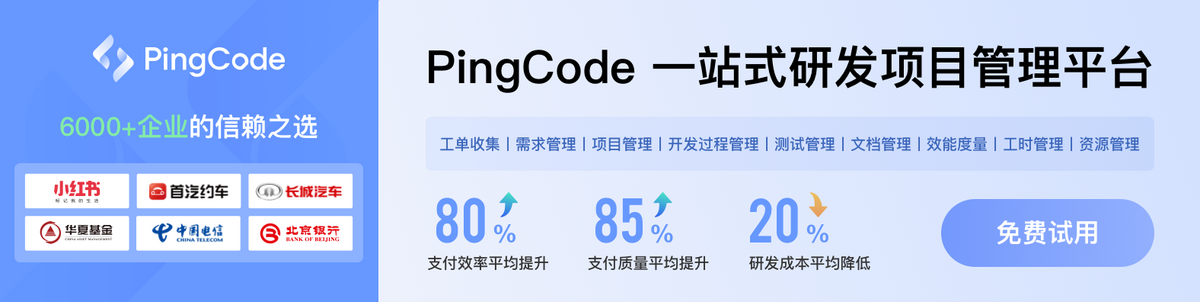