在Python中,定义及调用一个函数的步骤包括:使用def
关键字定义函数、为函数命名、添加参数、编写函数体、通过函数名和参数调用函数。其中,函数参数是定义和调用函数的关键部分。通过合理设计参数,可以使函数更加灵活和通用。接下来,我们将详细探讨Python中定义及调用函数的各个方面。
一、函数的定义
在Python中,函数是一组相关语句的集合,它们一起执行一个任务。函数可以帮助我们将代码组织成模块化的结构,使代码更易读、易维护。
1.1 使用def
关键字定义函数
定义函数的第一步是使用def
关键字。def
关键字告诉Python我们正在定义一个函数。函数名称应该具有描述性,能够反映函数的用途。
def function_name(parameters):
"""docstring"""
statement(s)
1.2 添加函数参数
函数参数允许我们向函数传递信息。参数可以是任何合法的Python变量名。函数参数是在函数定义时指定的,在调用函数时传递给函数。
def greet(name):
"""This function greets the person passed in as a parameter"""
print(f"Hello, {name}!")
在这个例子中,name
是函数的参数。
1.3 编写函数体
函数体是函数执行的代码块。它由一组缩进的语句组成。函数体中可以包含任何有效的Python语句。
def add(a, b):
"""This function returns the sum of two numbers"""
return a + b
二、函数的调用
定义函数之后,我们需要通过函数名和参数来调用它。调用函数的过程就是执行函数定义中的代码。
2.1 基本函数调用
函数调用包括函数名和一组括号,括号中包含参数。
greet('Alice')
2.2 函数返回值
函数可以返回一个值,使用return
语句来指定返回值。
result = add(2, 3)
print(result) # 输出 5
2.3 参数传递
函数可以接受不同类型的参数,包括位置参数、关键字参数和默认参数。
位置参数
位置参数是最常见的参数类型。它们根据参数在函数定义中的位置进行传递。
def multiply(a, b):
return a * b
result = multiply(4, 5)
print(result) # 输出 20
关键字参数
关键字参数允许我们在函数调用时显式地指定参数的名称。这使得函数调用更具可读性。
def divide(a, b):
return a / b
result = divide(a=10, b=2)
print(result) # 输出 5.0
默认参数
默认参数允许我们为函数参数指定默认值。如果在函数调用时未提供参数,则使用默认值。
def power(base, exponent=2):
return base exponent
print(power(3)) # 输出 9
print(power(2, 3)) # 输出 8
三、函数的高级特性
Python函数具有许多高级特性,使其更加灵活和强大。
3.1 可变参数
Python允许我们定义接受可变数量参数的函数。使用*args
和kwargs
可以实现这一功能。
*args
*args
用于传递不定数量的位置参数。
def sum_all(*args):
return sum(args)
print(sum_all(1, 2, 3, 4)) # 输出 10
kwargs
kwargs
用于传递不定数量的关键字参数。
def print_info(kwargs):
for key, value in kwargs.items():
print(f"{key}: {value}")
print_info(name='Alice', age=25, city='New York')
3.2 Lambda函数
Lambda函数是一种匿名函数,使用lambda
关键字定义。它们通常用于需要一个简单函数的地方。
add = lambda x, y: x + y
print(add(3, 5)) # 输出 8
3.3 函数作为参数
在Python中,函数可以作为参数传递给其他函数。这使得我们可以创建高阶函数。
def apply_func(func, value):
return func(value)
def square(x):
return x * x
print(apply_func(square, 4)) # 输出 16
3.4 闭包
闭包是一种特殊的函数,它能够捕获并记住其所在环境中的变量。闭包通常用于实现装饰器和回调函数。
def outer_function(msg):
def inner_function():
print(msg)
return inner_function
hello_func = outer_function('Hello, World!')
hello_func() # 输出 Hello, World!
四、函数的应用场景
函数在编程中有着广泛的应用,它们可以帮助我们实现代码的模块化、复用和抽象。
4.1 模块化编程
通过将代码分解成函数,我们可以更容易地管理和维护代码。每个函数完成特定的任务,使得代码结构更加清晰。
def read_file(file_path):
"""Read and return the content of a file"""
with open(file_path, 'r') as file:
return file.read()
def process_data(data):
"""Process the data and return the result"""
# 处理数据的逻辑
return processed_data
def write_file(file_path, data):
"""Write the processed data to a file"""
with open(file_path, 'w') as file:
file.write(data)
使用函数实现文件处理流程
data = read_file('input.txt')
processed_data = process_data(data)
write_file('output.txt', processed_data)
4.2 代码复用
通过定义函数,我们可以在不同的地方重复使用相同的代码。这减少了代码的冗余,提高了代码的可维护性。
def calculate_area(radius):
"""Calculate the area of a circle given its radius"""
return 3.14159 * (radius 2)
area1 = calculate_area(5)
area2 = calculate_area(10)
4.3 抽象和封装
函数可以帮助我们实现代码的抽象和封装。通过隐藏实现细节,我们可以简化代码的使用和理解。
def send_email(to, subject, body):
"""Send an email with the given details"""
# 邮件发送的实现细节
pass
使用函数发送邮件
send_email('example@example.com', 'Subject', 'Email body')
五、函数的最佳实践
为了编写高质量的函数,我们需要遵循一些最佳实践。这些实践可以帮助我们编写出更清晰、可维护的代码。
5.1 函数命名
函数名称应该具有描述性,能够反映函数的用途。使用动词短语命名函数是一个常见的做法。
def calculate_total(price, quantity):
"""Calculate the total price given the unit price and quantity"""
return price * quantity
5.2 函数长度
函数应该尽量短小,每个函数只完成一个任务。如果函数过长,可以考虑将其拆分成多个较小的函数。
def process_order(order):
"""Process an order"""
validate_order(order)
calculate_total(order)
update_inventory(order)
send_confirmation(order)
5.3 文档字符串
为每个函数编写文档字符串(docstring),描述函数的功能、参数和返回值。这有助于提高代码的可读性和可维护性。
def calculate_discount(price, discount_rate):
"""
Calculate the discounted price.
Args:
price (float): The original price.
discount_rate (float): The discount rate (e.g., 0.1 for 10%).
Returns:
float: The discounted price.
"""
return price * (1 - discount_rate)
5.4 参数数量
尽量减少函数的参数数量。如果参数过多,可以考虑使用类或字典来封装参数。
class Order:
def __init__(self, price, quantity, discount_rate):
self.price = price
self.quantity = quantity
self.discount_rate = discount_rate
def calculate_order_total(order):
"""Calculate the total price of an order"""
return order.price * order.quantity * (1 - order.discount_rate)
5.5 测试
为函数编写测试用例,确保函数的正确性。可以使用Python的unittest
模块或其他测试框架进行测试。
import unittest
def add(a, b):
return a + b
class TestMathFunctions(unittest.TestCase):
def test_add(self):
self.assertEqual(add(2, 3), 5)
self.assertEqual(add(-1, 1), 0)
if __name__ == '__main__':
unittest.main()
六、总结
Python中的函数是强大且灵活的工具,它们在编程中扮演着重要角色。通过定义和调用函数,我们可以实现代码的模块化、复用和抽象,提高代码的可维护性和可读性。在编写函数时,遵循最佳实践可以帮助我们编写出高质量的代码。无论是处理简单的任务,还是实现复杂的逻辑,函数都是不可或缺的编程工具。
相关问答FAQs:
如何在Python中定义一个简单的函数?
在Python中,定义一个函数非常简单。可以使用def
关键字,后跟函数名和括号内的参数列表。例如:
def greet(name):
return f"Hello, {name}!"
这个函数接收一个参数name
,并返回一个问候语。
调用函数时需要注意哪些事项?
调用函数时,需要确保提供了正确数量和类型的参数。例如,使用前面定义的greet
函数时:
message = greet("Alice")
print(message) # 输出: Hello, Alice!
确保在调用时传入与定义时相同的数据类型。
如何定义具有默认参数的函数?
在Python中,可以为函数的参数设置默认值,这样在调用时可以选择性地传入参数。例如:
def greet(name="Guest"):
return f"Hello, {name}!"
如果在调用时没有提供name
参数,函数将使用默认值"Guest"
。这样可以使函数更加灵活。调用时:
print(greet()) # 输出: Hello, Guest!
print(greet("Bob")) # 输出: Hello, Bob!
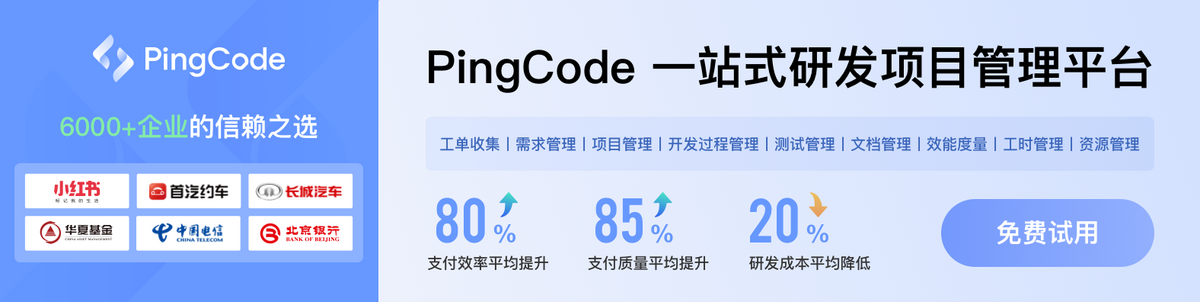