Python 输出 txt 中某一行的方法有多种,包括使用文件读取、列表索引、正则表达式等方式。其中,最常用的方式是通过逐行读取文件并存储到列表中,然后根据索引提取特定行。接下来,我们将详细介绍如何在 Python 中实现这一功能,并提供一些专业的经验见解。
一、文件读取与列表索引
1、逐行读取文件
Python 提供了多种读取文件的方法,其中最常用的是使用 open
函数和 readlines
方法。以下是一个简单的示例:
def read_specific_line(file_path, line_number):
with open(file_path, 'r') as file:
lines = file.readlines()
return lines[line_number - 1].strip()
这段代码中,我们首先打开文件,然后使用 readlines
方法将文件内容逐行读取并存储在一个列表中。通过列表索引,我们可以很方便地提取特定行。
2、使用异常处理确保稳健性
在实际应用中,文件可能不存在或行号超出范围,为了使程序更加健壮,我们可以添加异常处理:
def read_specific_line(file_path, line_number):
try:
with open(file_path, 'r') as file:
lines = file.readlines()
return lines[line_number - 1].strip()
except FileNotFoundError:
return "Error: File not found."
except IndexError:
return "Error: Line number out of range."
通过捕捉 FileNotFoundError
和 IndexError
异常,我们可以提供更加友好的错误提示。
二、逐行读取文件,减少内存占用
对于大文件,逐行读取并存储到列表中可能会占用大量内存。此时,可以考虑逐行读取并进行处理:
def read_specific_line(file_path, line_number):
try:
with open(file_path, 'r') as file:
for current_line_number, line in enumerate(file, start=1):
if current_line_number == line_number:
return line.strip()
return "Error: Line number out of range."
except FileNotFoundError:
return "Error: File not found."
这种方法通过使用 enumerate
函数逐行读取文件,并在找到目标行时立即返回结果,从而避免了将整个文件内容存储到内存中的问题。
三、使用正则表达式匹配特定行
在某些情况下,我们可能需要根据特定的模式匹配某一行内容。此时,可以使用正则表达式:
import re
def read_line_with_pattern(file_path, pattern):
try:
with open(file_path, 'r') as file:
for line in file:
if re.search(pattern, line):
return line.strip()
return "Error: No matching pattern found."
except FileNotFoundError:
return "Error: File not found."
这种方法通过在逐行读取文件时使用 re.search
匹配特定模式,可以实现更加灵活的行提取。
四、结合多种方法提高灵活性
在实际应用中,我们可能需要结合多种方法,以提高程序的灵活性和功能性。以下是一个结合了逐行读取、列表索引和正则表达式的示例:
import re
def read_specific_line(file_path, line_number=None, pattern=None):
try:
with open(file_path, 'r') as file:
if line_number is not None:
lines = file.readlines()
if line_number <= len(lines):
return lines[line_number - 1].strip()
else:
return "Error: Line number out of range."
elif pattern is not None:
for line in file:
if re.search(pattern, line):
return line.strip()
return "Error: No matching pattern found."
else:
return "Error: No line number or pattern provided."
except FileNotFoundError:
return "Error: File not found."
这种方法通过参数 line_number
和 pattern
提供了两种不同的行提取方式,并根据输入参数选择合适的提取方法。
五、使用 pandas 读取特定行
对于结构化数据文件(如 CSV 文件),可以使用 pandas
库来读取特定行:
import pandas as pd
def read_csv_line(file_path, line_number):
try:
df = pd.read_csv(file_path)
if line_number <= len(df):
return df.iloc[line_number - 1].to_dict()
else:
return "Error: Line number out of range."
except FileNotFoundError:
return "Error: File not found."
except pd.errors.EmptyDataError:
return "Error: No data in file."
pandas
库提供了强大的数据处理功能,通过 read_csv
方法可以方便地读取 CSV 文件,并通过 iloc
方法提取特定行的数据。
六、总结
通过上述多种方法,我们可以根据具体需求灵活选择合适的行提取方式。逐行读取文件并存储到列表中、逐行读取减少内存占用、使用正则表达式匹配特定行、结合多种方法提高灵活性、使用 pandas 读取结构化数据,这些方法各有优缺点,适用于不同的应用场景。
在实际项目中,根据具体需求和数据规模,选择合适的方法可以提高程序的健壮性和性能。例如,对于大文件,逐行读取并进行处理可以有效减少内存占用;对于结构化数据,使用 pandas 可以提供更为方便的数据操作接口。
相关问答FAQs:
如何在Python中读取特定的文本行?
在Python中,可以使用文件对象的readlines()
方法将文本文件的每一行读取到一个列表中。然后,可以通过列表索引来访问特定的行。例如,如果你想获取文件中的第二行,可以这样做:
with open('yourfile.txt', 'r') as file:
lines = file.readlines()
specific_line = lines[1] # 注意索引从0开始
print(specific_line)
在Python中如何处理大文件以提高效率?
处理大文件时,直接读取所有行可能会导致内存不足。可以使用enumerate()
函数结合for
循环逐行读取文件,这样可以在处理每一行时检查行号,从而找到你需要的行。例如:
with open('yourfile.txt', 'r') as file:
for index, line in enumerate(file):
if index == 1: # 获取第二行
print(line)
break
如何在Python中输出特定行的内容而不加载整个文件?
如果你只想输出某一行而不加载整个文件,可以使用文件对象的seek()
方法和readline()
方法。这种方法适合于已知行长度的情况,但需要注意行长度的变化可能会影响结果。以下是一个示例:
with open('yourfile.txt', 'r') as file:
for _ in range(1): # 跳过第一行
file.readline()
specific_line = file.readline() # 读取第二行
print(specific_line)
通过这些方法,你可以灵活地在Python中读取和输出文本文件中的特定行,优化你的代码并提高性能。
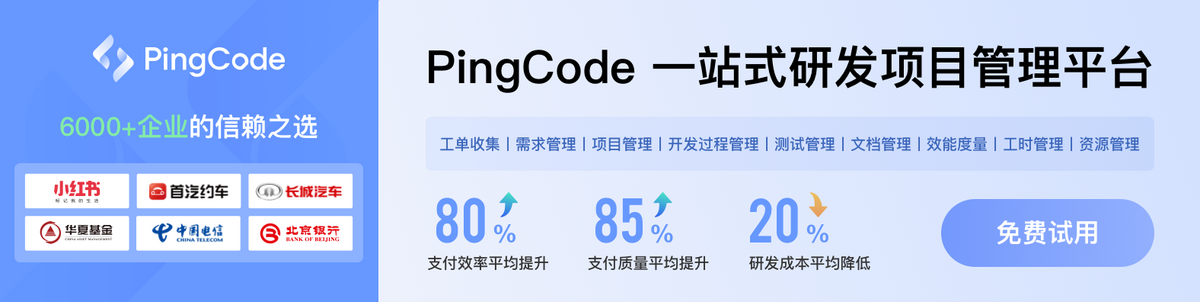