通过Python将txt文件转换成csv有多种方法,其中包括使用内置的csv模块、pandas库、手动字符串处理等方法。本文将详细介绍如何使用这些方法进行txt文件到csv文件的转换,并探讨每种方法的优缺点。
一、使用内置的csv模块
Python的csv模块提供了对CSV文件的读写支持。对于简单的txt文件到csv文件转换,csv模块是一个很好的选择。
1. 读取txt文件并写入csv文件
使用csv模块的核心在于其reader
和writer
对象。以下是一个基本示例,展示如何使用这些对象来完成txt到csv的转换:
import csv
假设txt文件中的数据是以空格分隔的
input_file = 'input.txt'
output_file = 'output.csv'
with open(input_file, 'r') as txt_file, open(output_file, 'w', newline='') as csv_file:
csv_writer = csv.writer(csv_file)
for line in txt_file:
# 分割行中的数据
row = line.strip().split()
csv_writer.writerow(row)
2. 处理复杂的txt文件格式
有时候,txt文件中的数据可能不那么简单,需要一些预处理。比如,行中的数据可能由不同的分隔符分隔,或者数据中包含需要过滤的无效字符。
import csv
import re
input_file = 'complex_input.txt'
output_file = 'output.csv'
with open(input_file, 'r') as txt_file, open(output_file, 'w', newline='') as csv_file:
csv_writer = csv.writer(csv_file)
for line in txt_file:
# 预处理行数据
line = re.sub(r'[^\w\s]', '', line) # 删除非字母数字字符
row = line.strip().split() # 根据空格分割
csv_writer.writerow(row)
二、使用pandas库
pandas是一个功能强大的数据处理库,能够轻松地进行txt文件到csv文件的转换。它的read_csv
和to_csv
方法非常便捷。
1. 读取txt文件并写入csv文件
pandas的read_csv
方法不仅能读取csv文件,还能读取以特定分隔符分隔的txt文件。
import pandas as pd
input_file = 'input.txt'
output_file = 'output.csv'
假设txt文件中的数据是以空格分隔的
df = pd.read_csv(input_file, delim_whitespace=True)
df.to_csv(output_file, index=False)
2. 处理复杂的txt文件格式
对于复杂的txt文件,可以使用pandas的更多功能,比如自定义分隔符、处理缺失值等。
import pandas as pd
input_file = 'complex_input.txt'
output_file = 'output.csv'
读取txt文件并处理分隔符
df = pd.read_csv(input_file, delimiter='|', engine='python') # 使用自定义分隔符
df.fillna('missing', inplace=True) # 处理缺失值
df.to_csv(output_file, index=False)
三、手动字符串处理
有时候,txt文件的格式非常特殊或复杂,无法直接使用csv模块或pandas库进行转换。这时,可以考虑手动处理字符串。
1. 读取txt文件并处理字符串
通过手动处理字符串,可以实现更为灵活的格式转换。
input_file = 'special_input.txt'
output_file = 'output.csv'
with open(input_file, 'r') as txt_file, open(output_file, 'w') as csv_file:
for line in txt_file:
# 自定义字符串处理逻辑
line = line.replace('|', ',') # 将竖线替换为逗号
csv_file.write(line)
2. 处理复杂的字符串格式
对于更加复杂的格式,可以使用正则表达式或其他字符串处理方法。
import re
input_file = 'complex_input.txt'
output_file = 'output.csv'
with open(input_file, 'r') as txt_file, open(output_file, 'w') as csv_file:
for line in txt_file:
# 使用正则表达式处理字符串
line = re.sub(r'\s+', ',', line) # 将多个空白字符替换为逗号
csv_file.write(line)
四、性能与优化
在处理大文件时,性能可能成为一个问题。以下是一些优化建议:
1. 使用生成器
生成器可以帮助减少内存使用,特别是在处理大文件时。
import csv
def read_large_file(file_object):
while True:
data = file_object.readline()
if not data:
break
yield data
input_file = 'large_input.txt'
output_file = 'output.csv'
with open(input_file, 'r') as txt_file, open(output_file, 'w', newline='') as csv_file:
csv_writer = csv.writer(csv_file)
for line in read_large_file(txt_file):
row = line.strip().split()
csv_writer.writerow(row)
2. 并行处理
对于非常大的文件,可以考虑使用多线程或多进程进行并行处理。
from concurrent.futures import ProcessPoolExecutor
import csv
def process_line(line):
return line.strip().split()
input_file = 'large_input.txt'
output_file = 'output.csv'
with open(input_file, 'r') as txt_file:
lines = txt_file.readlines()
with ProcessPoolExecutor() as executor, open(output_file, 'w', newline='') as csv_file:
csv_writer = csv.writer(csv_file)
for row in executor.map(process_line, lines):
csv_writer.writerow(row)
五、总结
通过本文的介绍,我们了解了如何使用Python将txt文件转换成csv文件,主要方法包括使用内置的csv模块、pandas库和手动字符串处理。每种方法都有其优缺点,选择合适的方法可以提高效率和简化代码。对于简单的txt文件,csv模块已经足够;对于复杂的文件格式,pandas库提供了更多的功能和灵活性;对于非常特殊的格式,可以通过手动处理字符串来实现。在处理大文件时,优化性能的方法如使用生成器和并行处理也非常重要。
希望这篇文章能帮助你更好地理解和实现txt文件到csv文件的转换。如果你有任何问题或建议,欢迎在评论区留言。
相关问答FAQs:
如何使用Python读取txt文件并将其转换为csv格式?
可以使用Python中的pandas库来轻松实现这一转换。首先,使用pandas.read_csv()
函数读取txt文件,指定分隔符,然后使用DataFrame.to_csv()
方法将其保存为csv格式。确保在读取时正确设定分隔符,比如制表符或空格,以便正确解析数据。
在转换过程中如何处理缺失值或异常数据?
在转换txt文件为csv时,可以使用pandas的fillna()
方法来填充缺失值,或使用dropna()
方法删除包含缺失值的行。此外,可以使用DataFrame.replace()
方法来处理异常数据,确保转换后的csv文件数据的准确性和完整性。
转换后如何验证csv文件的正确性?
可以通过使用pandas的read_csv()
函数重新读取生成的csv文件,然后与原始数据进行比较来验证。也可以使用DataFrame.info()
和DataFrame.describe()
方法检查数据的结构和统计信息,确保转换后数据的完整性和一致性。
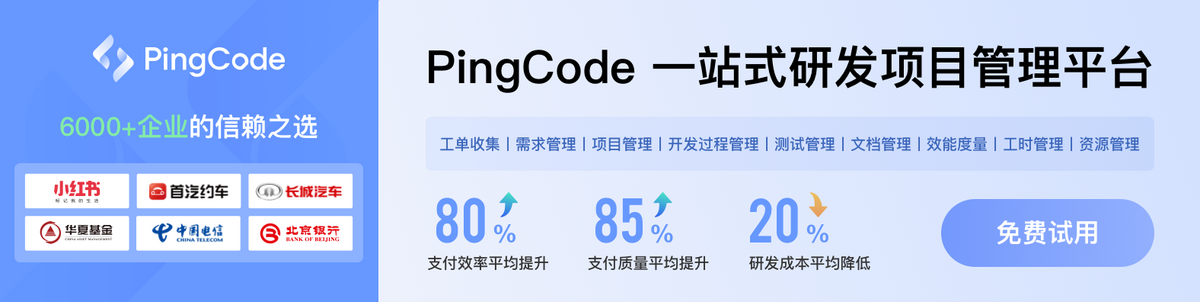