如何用Python编写一个简单的爬虫
编写一个简单的Python爬虫涉及到多个关键步骤:选择合适的库、发送HTTP请求、解析HTML内容、处理和存储数据。其中,选择合适的库非常重要,因为不同的库有不同的功能和性能。在本文中,我们将详细介绍每个步骤,并用实例来演示如何实现一个基本的Python爬虫。
一、选择合适的库
Python提供了多个库来帮助我们编写爬虫。最常用的库包括Requests、BeautifulSoup和Scrapy。
1、Requests库
Requests库是一个简单易用的HTTP库,适合初学者使用。它可以帮助我们发送HTTP请求并获取响应内容。
import requests
response = requests.get('https://example.com')
print(response.text)
2、BeautifulSoup库
BeautifulSoup库是一个HTML解析库,它可以帮助我们从HTML文档中提取数据。配合Requests库使用非常高效。
from bs4 import BeautifulSoup
html_content = '<html><head><title>Example</title></head><body><p>Hello World</p></body></html>'
soup = BeautifulSoup(html_content, 'html.parser')
print(soup.title.string)
3、Scrapy框架
Scrapy是一个功能强大的爬虫框架,适合处理复杂的爬虫任务。它具有高效的数据提取和存储功能,适合大规模数据抓取。
import scrapy
class ExampleSpider(scrapy.Spider):
name = 'example'
start_urls = ['https://example.com']
def parse(self, response):
title = response.css('title::text').get()
yield {'title': title}
二、发送HTTP请求
发送HTTP请求是爬虫的第一步。我们需要使用Requests库来发送请求并获取响应内容。
1、基本的GET请求
GET请求是最常见的HTTP请求方法,用于从服务器获取数据。
import requests
response = requests.get('https://example.com')
print(response.status_code)
print(response.text)
2、处理响应内容
响应内容可以是HTML、JSON、XML等格式。我们需要根据具体情况选择合适的解析方法。
import requests
response = requests.get('https://api.example.com/data')
if response.headers['Content-Type'] == 'application/json':
data = response.json()
print(data)
else:
print(response.text)
三、解析HTML内容
解析HTML内容是爬虫的核心步骤之一。我们可以使用BeautifulSoup库来解析HTML文档并提取所需的数据。
1、解析HTML文档
使用BeautifulSoup库可以轻松解析HTML文档并提取数据。
from bs4 import BeautifulSoup
html_content = '<html><head><title>Example</title></head><body><p>Hello World</p></body></html>'
soup = BeautifulSoup(html_content, 'html.parser')
print(soup.title.string)
print(soup.p.text)
2、选择器和查找方法
BeautifulSoup提供了多种选择器和查找方法,帮助我们从HTML文档中提取数据。
from bs4 import BeautifulSoup
html_content = '''
<html>
<head><title>Example</title></head>
<body>
<p class="content">Hello World</p>
<p class="content">Hello Python</p>
</body>
</html>
'''
soup = BeautifulSoup(html_content, 'html.parser')
paragraphs = soup.find_all('p', class_='content')
for p in paragraphs:
print(p.text)
四、处理和存储数据
处理和存储数据是爬虫的最后一步。我们可以将数据存储到数据库、文件或其他数据存储系统中。
1、存储到文件
将数据存储到文件是最简单的方法之一。我们可以使用Python内置的文件操作方法来实现。
data = ['Hello World', 'Hello Python']
with open('data.txt', 'w') as file:
for item in data:
file.write(item + '\n')
2、存储到数据库
将数据存储到数据库可以更方便地进行查询和管理。我们可以使用SQLite数据库来存储数据。
import sqlite3
data = [('Hello World',), ('Hello Python',)]
conn = sqlite3.connect('data.db')
cursor = conn.cursor()
cursor.execute('CREATE TABLE IF NOT EXISTS example (text TEXT)')
cursor.executemany('INSERT INTO example (text) VALUES (?)', data)
conn.commit()
conn.close()
五、实例演示
下面是一个完整的示例,演示了如何编写一个简单的Python爬虫,抓取网页内容并存储到文件中。
import requests
from bs4 import BeautifulSoup
发送HTTP请求
response = requests.get('https://example.com')
解析HTML内容
soup = BeautifulSoup(response.text, 'html.parser')
titles = soup.find_all('h1')
处理和存储数据
with open('titles.txt', 'w') as file:
for title in titles:
file.write(title.text + '\n')
print('Data has been saved to titles.txt')
六、处理常见问题
在编写爬虫的过程中,我们可能会遇到一些常见问题,如IP被封禁、反爬虫机制等。
1、处理IP封禁
为了防止IP被封禁,我们可以使用代理IP或设置请求头信息。
import requests
proxies = {
'http': 'http://10.10.1.10:3128',
'https': 'http://10.10.1.10:1080',
}
response = requests.get('https://example.com', proxies=proxies)
print(response.text)
2、处理反爬虫机制
为了绕过反爬虫机制,我们可以模拟浏览器行为或使用随机延迟。
import requests
import random
import time
headers = {
'User-Agent': 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/91.0.4472.124 Safari/537.36'
}
response = requests.get('https://example.com', headers=headers)
time.sleep(random.uniform(1, 3))
print(response.text)
七、提高爬虫效率
提高爬虫效率可以让我们更快地获取数据。我们可以使用多线程或异步IO来提高爬虫的并发能力。
1、多线程爬虫
使用多线程可以同时发送多个请求,从而提高爬虫效率。
import requests
from bs4 import BeautifulSoup
import threading
def fetch_url(url):
response = requests.get(url)
soup = BeautifulSoup(response.text, 'html.parser')
print(soup.title.string)
urls = ['https://example.com/page1', 'https://example.com/page2', 'https://example.com/page3']
threads = []
for url in urls:
thread = threading.Thread(target=fetch_url, args=(url,))
threads.append(thread)
thread.start()
for thread in threads:
thread.join()
2、异步IO爬虫
使用异步IO可以更高效地处理I/O操作,从而进一步提高爬虫效率。
import aiohttp
import asyncio
from bs4 import BeautifulSoup
async def fetch_url(session, url):
async with session.get(url) as response:
text = await response.text()
soup = BeautifulSoup(text, 'html.parser')
print(soup.title.string)
async def main():
urls = ['https://example.com/page1', 'https://example.com/page2', 'https://example.com/page3']
async with aiohttp.ClientSession() as session:
tasks = [fetch_url(session, url) for url in urls]
await asyncio.gather(*tasks)
asyncio.run(main())
八、总结
编写一个简单的Python爬虫包括选择合适的库、发送HTTP请求、解析HTML内容、处理和存储数据等步骤。我们可以使用Requests库发送HTTP请求,使用BeautifulSoup库解析HTML内容,并将数据存储到文件或数据库中。同时,为了提高爬虫效率,我们可以使用多线程或异步IO技术。在实际应用中,我们还需要处理常见问题,如IP被封禁和反爬虫机制等。通过合理的设计和优化,我们可以编写出高效、稳定的Python爬虫,满足各种数据抓取需求。
相关问答FAQs:
如何选择合适的Python库来编写爬虫?
在编写Python爬虫时,选择合适的库至关重要。常用的库包括Requests和BeautifulSoup,前者用于发送网络请求,后者则用于解析HTML文档。如果你需要处理JavaScript生成的内容,可以考虑使用Selenium或Playwright。此外,Scrapy是一个功能强大的框架,适合构建更复杂的爬虫项目。
编写爬虫时需要注意哪些法律和道德问题?
在编写爬虫时,应遵守网站的robots.txt文件中规定的爬取规则,确保不违反网站的使用条款。同时,避免过于频繁的请求,以免给网站带来负担,导致IP被封禁。尊重数据隐私和版权,确保在使用爬取的数据时遵循相关法律法规。
如何处理爬虫过程中可能遇到的反爬虫机制?
许多网站采用反爬虫机制来防止自动化请求。为了应对这些挑战,可以使用随机的User-Agent头部、设置请求间隔、使用代理IP等方法。此外,模拟用户行为(如处理Cookies和Session)也能提高爬虫的成功率。在必要时,可以考虑使用更高级的工具,如Selenium,来处理动态加载的页面内容。
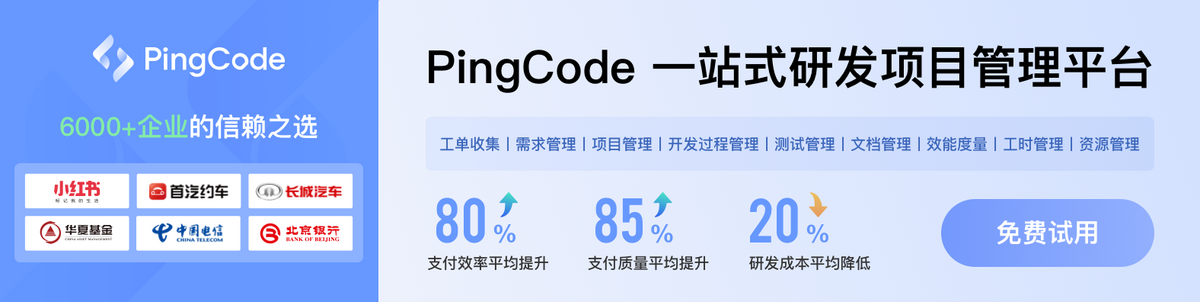