Python中字符串匹配字符串长度的方法有多种,包括使用内置函数len()、正则表达式、以及其他字符串处理方法。 在实际应用中,len()函数 是最常用的工具,因为它简单、直观,并且性能优越。下面将详细描述如何使用这些方法,以及它们的优缺点。
一、len()函数
len()函数是Python内置函数,用于获取字符串的长度。使用非常简单,通常是解决字符串长度匹配问题的第一选择。
示例代码:
string = "Hello, World!"
length = len(string)
print(f"The length of the string is: {length}")
在这个例子中,len()
函数直接返回字符串的长度,并且将其打印出来。这个方法的优点是简洁明了,缺点是无法处理复杂的匹配条件。
二、使用正则表达式
正则表达式(Regular Expressions,简称Regex)是一种强大的字符串匹配工具。通过正则表达式,我们可以实现更复杂的字符串长度匹配。
示例代码:
import re
string = "Hello, World!"
pattern = "^.{5,10}$" # 匹配长度在5到10之间的字符串
if re.match(pattern, string):
print("The string matches the length criteria.")
else:
print("The string does not match the length criteria.")
在这个例子中,正则表达式^.{5,10}$
用来匹配长度在5到10之间的字符串。正则表达式的优点是灵活,可以处理各种复杂的匹配条件。缺点是语法较为复杂,不易理解。
三、字符串分割和切片
除了len()函数和正则表达式,Python还提供了字符串分割和切片的方法来处理字符串长度匹配。这些方法通常用于更复杂的字符串处理任务。
示例代码:
string = "Hello, World!"
substring = string[:5] # 获取前5个字符
if len(substring) == 5:
print("The substring length is 5.")
else:
print("The substring length is not 5.")
在这个例子中,我们使用切片操作获取字符串的前5个字符,然后使用len()函数进行长度匹配。这种方法的优点是灵活,可以处理各种复杂的字符串操作任务。缺点是需要多步操作,代码较为冗长。
四、字符串操作中的其他方法
在实际项目中,有时我们需要结合多种字符串操作方法来实现复杂的字符串长度匹配任务。以下是一些常用的方法:
1. 字符串拼接
字符串拼接是将多个字符串合并成一个字符串的操作。通过拼接,我们可以创建新的字符串,并进行长度匹配。
string1 = "Hello"
string2 = ", World!"
combined_string = string1 + string2
if len(combined_string) == 13:
print("The combined string length is 13.")
else:
print("The combined string length is not 13.")
2. 字符串替换
字符串替换是将字符串中的某些子字符串替换为其他子字符串的操作。通过替换,我们可以修改字符串的长度,并进行长度匹配。
string = "Hello, World!"
modified_string = string.replace("World", "Python")
if len(modified_string) == 13:
print("The modified string length is 13.")
else:
print("The modified string length is not 13.")
3. 字符串查找
字符串查找是查找字符串中某个子字符串的位置的操作。通过查找,我们可以确定子字符串的长度,并进行长度匹配。
string = "Hello, World!"
position = string.find("World")
if position != -1:
print(f"The substring 'World' starts at position {position}.")
else:
print("The substring 'World' was not found.")
五、实战应用
在实际项目中,字符串长度匹配常常与其他字符串操作结合使用。以下是几个常见的实战应用场景:
1. 用户输入验证
在用户输入验证中,我们需要检查用户输入的字符串长度是否符合要求。可以使用len()函数或正则表达式进行验证。
def validate_input(user_input):
if len(user_input) < 5 or len(user_input) > 10:
return False
return True
user_input = input("Enter a string: ")
if validate_input(user_input):
print("Valid input.")
else:
print("Invalid input. Please enter a string between 5 and 10 characters.")
2. 文件处理
在文件处理中,我们可能需要读取文件中的每一行,并检查其长度是否符合要求。可以使用len()函数或其他字符串操作方法进行处理。
with open("example.txt", "r") as file:
for line in file:
if len(line.strip()) > 80:
print(f"Line exceeds 80 characters: {line.strip()}")
3. 数据清洗
在数据清洗过程中,我们需要对原始数据进行处理,确保每个字段的长度符合要求。可以使用len()函数或正则表达式进行数据清洗。
data = ["Hello", "World", "Python", "Data Science"]
cleaned_data = [item for item in data if len(item) > 3]
print(cleaned_data)
六、性能优化
在处理大规模字符串数据时,性能是一个重要的考虑因素。以下是一些性能优化的建议:
1. 使用生成器
生成器是一种高效的迭代器,可以在处理大规模数据时节省内存。通过使用生成器,我们可以提高字符串长度匹配的性能。
def string_generator(file_path):
with open(file_path, "r") as file:
for line in file:
yield line.strip()
for string in string_generator("example.txt"):
if len(string) > 80:
print(f"Line exceeds 80 characters: {string}")
2. 并行处理
在处理大规模数据时,可以使用多线程或多进程进行并行处理。通过并行处理,我们可以提高字符串长度匹配的性能。
import concurrent.futures
def process_line(line):
if len(line.strip()) > 80:
return f"Line exceeds 80 characters: {line.strip()}"
return None
with open("example.txt", "r") as file:
lines = file.readlines()
with concurrent.futures.ThreadPoolExecutor() as executor:
results = executor.map(process_line, lines)
for result in results:
if result:
print(result)
七、总结
通过本文的介绍,我们学习了Python中字符串匹配字符串长度的多种方法,包括使用len()函数、正则表达式、字符串分割和切片、以及其他字符串操作方法。我们还探讨了这些方法在实际项目中的应用场景,并给出了性能优化的建议。
在实际应用中,选择合适的方法取决于具体的需求和数据规模。len()函数适用于简单的长度匹配任务,而正则表达式和其他字符串操作方法则适用于复杂的匹配条件和字符串处理任务。通过合理选择和组合这些方法,我们可以高效地解决字符串长度匹配问题。
相关问答FAQs:
如何在Python中判断字符串的长度?
在Python中,可以使用内置的len()
函数来判断字符串的长度。只需将字符串作为参数传递给该函数,便会返回字符串中字符的数量。例如,len("hello")
将返回5,因为“hello”包含5个字符。
在Python中,如何匹配特定长度的字符串?
要匹配特定长度的字符串,可以结合使用len()
函数与条件语句。可以这样写:if len(my_string) == desired_length:
,其中my_string
是待检查的字符串,desired_length
是你希望匹配的长度。如果条件成立,说明字符串的长度符合要求。
是否可以使用正则表达式来匹配字符串长度?
正则表达式是一个强大的工具,可以用于匹配字符串的模式,包括长度。使用re
模块,可以编写一个正则表达式,如r'^.{n}$'
,其中n
是你想要匹配的长度。例如,re.match(r'^.{5}$', my_string)
将匹配长度为5的字符串。如果匹配成功,返回的对象将是一个匹配结果;如果不匹配,将返回None
。
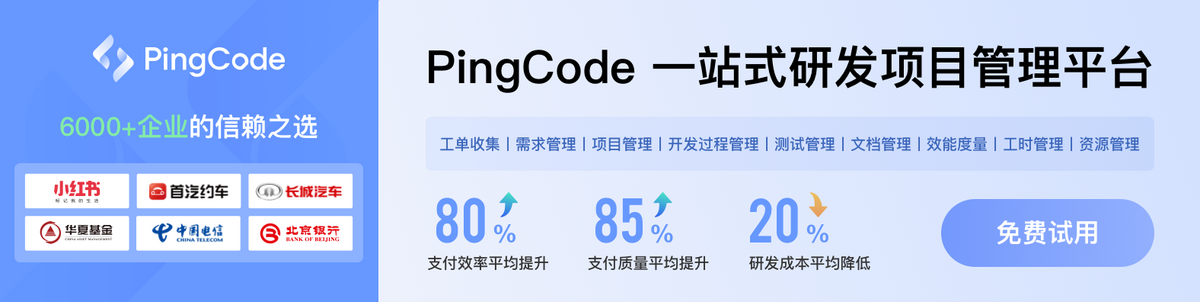