如何利用Python识别摄像头采集的图片
利用Python识别摄像头采集的图片可以通过使用OpenCV库、结合深度学习框架、进行图像预处理来实现。其中,OpenCV库提供了强大的图像处理功能,深度学习框架如TensorFlow和PyTorch可以用于模型训练和推理,图像预处理则有助于提高识别的准确性。以下将详细描述如何使用这些方法来实现图像识别。
一、安装和配置相关工具
1、安装Python及相关库
要使用Python识别摄像头采集的图片,首先需要安装Python及相关的库。推荐使用Anaconda来管理Python环境,因为它可以方便地处理依赖关系。
# 安装Anaconda
https://www.anaconda.com/products/individual
创建一个新的Python环境
conda create -n image_recognition python=3.8
激活环境
conda activate image_recognition
接下来,安装OpenCV、TensorFlow和其他必要的库:
pip install opencv-python
pip install tensorflow
pip install numpy
pip install matplotlib
2、设置摄像头
确保计算机已连接摄像头,并且摄像头驱动程序已正确安装。可以使用OpenCV来检测摄像头是否正常工作。
import cv2
打开摄像头
cap = cv2.VideoCapture(0)
检查摄像头是否成功打开
if not cap.isOpened():
print("无法打开摄像头")
exit()
读取并显示一帧图像
ret, frame = cap.read()
if ret:
cv2.imshow('frame', frame)
cv2.waitKey(0)
释放摄像头
cap.release()
cv2.destroyAllWindows()
二、图像采集与预处理
1、采集图像
使用OpenCV从摄像头采集图像。可以根据需要调整图像的分辨率和采集频率。
import cv2
打开摄像头
cap = cv2.VideoCapture(0)
while True:
ret, frame = cap.read()
if not ret:
break
# 显示图像
cv2.imshow('frame', frame)
# 按下 'q' 键退出
if cv2.waitKey(1) & 0xFF == ord('q'):
break
释放摄像头
cap.release()
cv2.destroyAllWindows()
2、图像预处理
图像预处理是提高识别准确性的重要步骤。常见的预处理方法包括灰度化、二值化、去噪、缩放等。
import cv2
def preprocess_image(image):
# 灰度化
gray_image = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY)
# 高斯模糊去噪
blurred_image = cv2.GaussianBlur(gray_image, (5, 5), 0)
# 二值化
_, binary_image = cv2.threshold(blurred_image, 128, 255, cv2.THRESH_BINARY)
# 缩放图像
resized_image = cv2.resize(binary_image, (224, 224))
return resized_image
测试预处理函数
cap = cv2.VideoCapture(0)
ret, frame = cap.read()
if ret:
processed_image = preprocess_image(frame)
cv2.imshow('Processed Image', processed_image)
cv2.waitKey(0)
cap.release()
cv2.destroyAllWindows()
三、使用深度学习模型进行识别
1、加载预训练模型
可以使用TensorFlow加载预训练的深度学习模型,如ResNet、MobileNet等。
import tensorflow as tf
加载预训练的MobileNetV2模型
model = tf.keras.applications.MobileNetV2(weights='imagenet', include_top=True)
显示模型结构
model.summary()
2、图像识别
使用预训练模型对预处理后的图像进行识别。
import numpy as np
from tensorflow.keras.applications.mobilenet_v2 import preprocess_input, decode_predictions
def recognize_image(image):
# 预处理图像
preprocessed_image = preprocess_input(image)
# 添加批量维度
preprocessed_image = np.expand_dims(preprocessed_image, axis=0)
# 预测
predictions = model.predict(preprocessed_image)
# 解码预测结果
decoded_predictions = decode_predictions(predictions, top=5)[0]
return decoded_predictions
测试识别函数
cap = cv2.VideoCapture(0)
ret, frame = cap.read()
if ret:
processed_image = preprocess_image(frame)
predictions = recognize_image(processed_image)
for pred in predictions:
print(f"{pred[1]}: {pred[2]*100:.2f}%")
cap.release()
四、优化与增强
1、提升识别速度
在实际应用中,识别速度是一个重要的考虑因素。可以通过以下方法提升识别速度:
- 使用GPU加速:TensorFlow和PyTorch都支持GPU加速,可以显著提升模型推理速度。
- 模型优化:使用TensorFlow Lite或ONNX等工具对模型进行优化。
- 降低分辨率:在保证识别精度的前提下,降低输入图像的分辨率。
# 使用GPU加速
gpus = tf.config.experimental.list_physical_devices('GPU')
if gpus:
try:
for gpu in gpus:
tf.config.experimental.set_memory_growth(gpu, True)
except RuntimeError as e:
print(e)
2、提高识别准确性
提高识别准确性的方法包括:
- 增加训练数据量:更多的训练数据通常可以提升模型的泛化能力。
- 数据增强:通过数据增强技术(如旋转、缩放、翻转等)生成更多的训练样本。
- 模型微调:在预训练模型的基础上,使用特定任务的数据进行微调。
from tensorflow.keras.preprocessing.image import ImageDataGenerator
数据增强
datagen = ImageDataGenerator(
rotation_range=20,
width_shift_range=0.2,
height_shift_range=0.2,
horizontal_flip=True
)
模型微调
base_model = tf.keras.applications.MobileNetV2(weights='imagenet', include_top=False)
x = base_model.output
x = tf.keras.layers.GlobalAveragePooling2D()(x)
x = tf.keras.layers.Dense(1024, activation='relu')(x)
predictions = tf.keras.layers.Dense(10, activation='softmax')(x)
model = tf.keras.models.Model(inputs=base_model.input, outputs=predictions)
冻结预训练模型的层
for layer in base_model.layers:
layer.trainable = False
model.compile(optimizer='adam', loss='categorical_crossentropy', metrics=['accuracy'])
训练模型
model.fit(datagen.flow(train_images, train_labels, batch_size=32), epochs=10)
五、部署与应用
1、将模型部署到边缘设备
可以将优化后的模型部署到边缘设备,如Raspberry Pi、NVIDIA Jetson等,以实现实时图像识别。
# 使用TensorFlow Lite转换模型
converter = tf.lite.TFLiteConverter.from_keras_model(model)
tflite_model = converter.convert()
保存模型
with open('model.tflite', 'wb') as f:
f.write(tflite_model)
2、集成到应用程序
将图像识别功能集成到实际应用程序中,如安全监控系统、智能家居等。
import cv2
import tensorflow as tf
加载TensorFlow Lite模型
interpreter = tf.lite.Interpreter(model_path='model.tflite')
interpreter.allocate_tensors()
获取输入和输出张量
input_details = interpreter.get_input_details()
output_details = interpreter.get_output_details()
打开摄像头
cap = cv2.VideoCapture(0)
while True:
ret, frame = cap.read()
if not ret:
break
# 预处理图像
processed_image = preprocess_image(frame)
# 添加批量维度
processed_image = np.expand_dims(processed_image, axis=0)
# 设置张量
interpreter.set_tensor(input_details[0]['index'], processed_image)
# 推理
interpreter.invoke()
# 获取预测结果
predictions = interpreter.get_tensor(output_details[0]['index'])
# 解析预测结果
decoded_predictions = decode_predictions(predictions, top=5)[0]
# 显示预测结果
for pred in decoded_predictions:
print(f"{pred[1]}: {pred[2]*100:.2f}%")
# 显示图像
cv2.imshow('frame', frame)
# 按下 'q' 键退出
if cv2.waitKey(1) & 0xFF == ord('q'):
break
cap.release()
cv2.destroyAllWindows()
六、总结
通过以上步骤,可以利用Python实现对摄像头采集图片的识别。关键步骤包括安装和配置相关工具、图像采集与预处理、使用深度学习模型进行识别、优化与增强、部署与应用。在实际应用中,可以根据具体需求对各个步骤进行调整和优化。
Python结合OpenCV和深度学习框架提供了强大的图像识别能力,适用于各种实际应用场景,如安全监控、智能家居、自动驾驶等。通过不断优化和调整,可以实现高效、准确的图像识别系统。
相关问答FAQs:
如何使用Python识别摄像头采集的图片?
要识别摄像头采集的图片,可以使用OpenCV库。首先,确保安装了OpenCV(使用命令pip install opencv-python
)。接着,通过摄像头捕捉图像,并将其传入识别模型,例如预训练的深度学习模型,如TensorFlow或PyTorch中的模型。使用这些模型可以实现对象检测、人脸识别等功能。
使用Python进行图像识别的最佳库有哪些?
在Python中,常用的图像识别库包括OpenCV、Pillow、TensorFlow和PyTorch。OpenCV是一个强大的计算机视觉库,适合实时图像处理;Pillow用于图像操作和处理;TensorFlow和PyTorch则提供了丰富的深度学习功能,适合训练和应用复杂的识别模型。
如何提高摄像头图像识别的准确性?
提高摄像头图像识别的准确性可以从多个方面着手。首先,确保光线充足,减少图像噪声;其次,使用高质量的摄像头以获取更清晰的图像;此外,可以通过数据增强方法来扩充训练数据集,或使用更复杂的深度学习模型来提升识别效果。同时,定期更新和优化模型也是非常重要的。
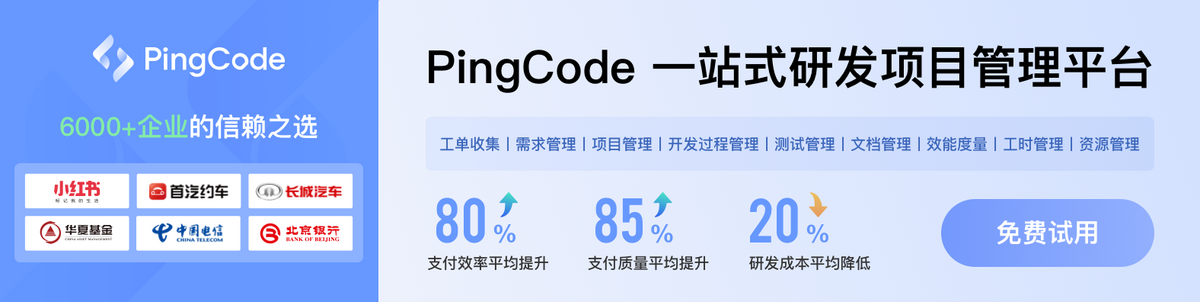