在Python中依次呈现四张图片的主要方法包括使用matplotlib、Pillow、OpenCV。其中,matplotlib 是最常用且高效的工具,因为它不仅可以处理图像,还可以进行数据可视化。下面将详细介绍如何使用这几种方法来呈现四张图片。
一、使用 Matplotlib
Matplotlib 是一个非常强大的绘图库,特别适合用于数据可视化和呈现图像。它的 pyplot
模块提供了便捷的接口来显示和操作图像。
1.1、安装和导入库
首先,需要确保已经安装了 Matplotlib 库。如果没有安装,可以使用以下命令进行安装:
pip install matplotlib
然后,在代码中导入所需的模块:
import matplotlib.pyplot as plt
import matplotlib.image as mpimg
1.2、读取和显示图像
使用 Matplotlib 读取和显示图像非常简单。假设有四张图片:image1.jpg
, image2.jpg
, image3.jpg
, image4.jpg
,我们可以将它们依次显示出来:
# 读取图像
img1 = mpimg.imread('image1.jpg')
img2 = mpimg.imread('image2.jpg')
img3 = mpimg.imread('image3.jpg')
img4 = mpimg.imread('image4.jpg')
创建一个包含4个子图的图形
fig, axs = plt.subplots(1, 4, figsize=(20, 5))
显示每张图像
axs[0].imshow(img1)
axs[0].set_title('Image 1')
axs[1].imshow(img2)
axs[1].set_title('Image 2')
axs[2].imshow(img3)
axs[2].set_title('Image 3')
axs[3].imshow(img4)
axs[3].set_title('Image 4')
去掉坐标轴
for ax in axs:
ax.axis('off')
显示图像
plt.show()
二、使用 Pillow
Pillow 是 Python Imaging Library(PIL)的分支,提供了强大的图像处理功能。使用 Pillow 可以非常方便地加载和显示图像。
2.1、安装和导入库
首先,确保安装了 Pillow 库:
pip install pillow
然后,在代码中导入所需的模块:
from PIL import Image
import matplotlib.pyplot as plt
2.2、读取和显示图像
使用 Pillow 读取和显示图像也非常简单:
# 读取图像
img1 = Image.open('image1.jpg')
img2 = Image.open('image2.jpg')
img3 = Image.open('image3.jpg')
img4 = Image.open('image4.jpg')
创建一个包含4个子图的图形
fig, axs = plt.subplots(1, 4, figsize=(20, 5))
显示每张图像
axs[0].imshow(img1)
axs[0].set_title('Image 1')
axs[1].imshow(img2)
axs[1].set_title('Image 2')
axs[2].imshow(img3)
axs[2].set_title('Image 3')
axs[3].imshow(img4)
axs[3].set_title('Image 4')
去掉坐标轴
for ax in axs:
ax.axis('off')
显示图像
plt.show()
三、使用 OpenCV
OpenCV 是一个开源计算机视觉库,广泛应用于图像处理和计算机视觉领域。它也可以用来读取和显示图像。
3.1、安装和导入库
首先,确保安装了 OpenCV 库:
pip install opencv-python
然后,在代码中导入所需的模块:
import cv2
import matplotlib.pyplot as plt
3.2、读取和显示图像
使用 OpenCV 读取和显示图像:
# 读取图像
img1 = cv2.imread('image1.jpg')
img2 = cv2.imread('image2.jpg')
img3 = cv2.imread('image3.jpg')
img4 = cv2.imread('image4.jpg')
OpenCV 读取的图像是 BGR 格式,需要转换为 RGB 格式
img1 = cv2.cvtColor(img1, cv2.COLOR_BGR2RGB)
img2 = cv2.cvtColor(img2, cv2.COLOR_BGR2RGB)
img3 = cv2.cvtColor(img3, cv2.COLOR_BGR2RGB)
img4 = cv2.cvtColor(img4, cv2.COLOR_BGR2RGB)
创建一个包含4个子图的图形
fig, axs = plt.subplots(1, 4, figsize=(20, 5))
显示每张图像
axs[0].imshow(img1)
axs[0].set_title('Image 1')
axs[1].imshow(img2)
axs[1].set_title('Image 2')
axs[2].imshow(img3)
axs[2].set_title('Image 3')
axs[3].imshow(img4)
axs[3].set_title('Image 4')
去掉坐标轴
for ax in axs:
ax.axis('off')
显示图像
plt.show()
四、总结
在 Python 中依次呈现四张图片可以通过多种方法实现,其中 Matplotlib、Pillow 和 OpenCV 是最常用的工具。这些工具各有优劣,可以根据具体需求选择合适的工具。Matplotlib 适合用于数据可视化和图像处理,Pillow 提供了强大的图像处理功能,而 OpenCV 则在计算机视觉领域有广泛应用。通过合理运用这些工具,可以轻松实现图像的读取和显示。
相关问答FAQs:
如何在Python中加载和显示多张图片?
在Python中,可以使用多个库来加载和显示图片,例如PIL(Pillow)、OpenCV和Matplotlib等。Pillow是一个强大的图像处理库,允许您轻松地打开和显示图片。使用Matplotlib,您可以将多张图片组合在一起显示。以下是一个简单的示例代码,使用Matplotlib加载并显示四张图片:
import matplotlib.pyplot as plt
import matplotlib.image as mpimg
# 读取图片
img1 = mpimg.imread('image1.jpg')
img2 = mpimg.imread('image2.jpg')
img3 = mpimg.imread('image3.jpg')
img4 = mpimg.imread('image4.jpg')
# 创建一个图形和子图
fig, axs = plt.subplots(2, 2)
# 显示图片
axs[0, 0].imshow(img1)
axs[0, 0].axis('off') # 关闭坐标轴
axs[0, 1].imshow(img2)
axs[0, 1].axis('off')
axs[1, 0].imshow(img3)
axs[1, 0].axis('off')
axs[1, 1].imshow(img4)
axs[1, 1].axis('off')
# 显示图形
plt.show()
如何在Python中按顺序显示图片的时间间隔?
如果您希望在Python中按顺序显示图片并指定时间间隔,可以使用Pillow和时间模块。通过设置时间延迟,您可以在展示每张图片后暂停一段时间。示例如下:
from PIL import Image
import time
images = ['image1.jpg', 'image2.jpg', 'image3.jpg', 'image4.jpg']
for img_path in images:
img = Image.open(img_path)
img.show()
time.sleep(2) # 暂停2秒
在Python中使用Tkinter展示图片有什么好处?
Tkinter是Python的标准GUI库,适合于构建简单的图形用户界面。使用Tkinter展示图片可以让您创建一个交互式应用程序,用户可以通过按钮或其他控件来浏览图片。以下是一个简单的Tkinter应用程序示例:
import tkinter as tk
from PIL import Image, ImageTk
class ImageViewer:
def __init__(self, master):
self.master = master
self.images = ['image1.jpg', 'image2.jpg', 'image3.jpg', 'image4.jpg']
self.index = 0
self.label = tk.Label(master)
self.label.pack()
self.show_image()
self.button = tk.Button(master, text='下一张', command=self.next_image)
self.button.pack()
def show_image(self):
img = Image.open(self.images[self.index])
img = ImageTk.PhotoImage(img)
self.label.config(image=img)
self.label.image = img
def next_image(self):
self.index = (self.index + 1) % len(self.images)
self.show_image()
root = tk.Tk()
viewer = ImageViewer(root)
root.mainloop()
这些方法为在Python中展示多张图片提供了多样化的选择,您可以根据自己的需求和使用场景选择适合的方式。
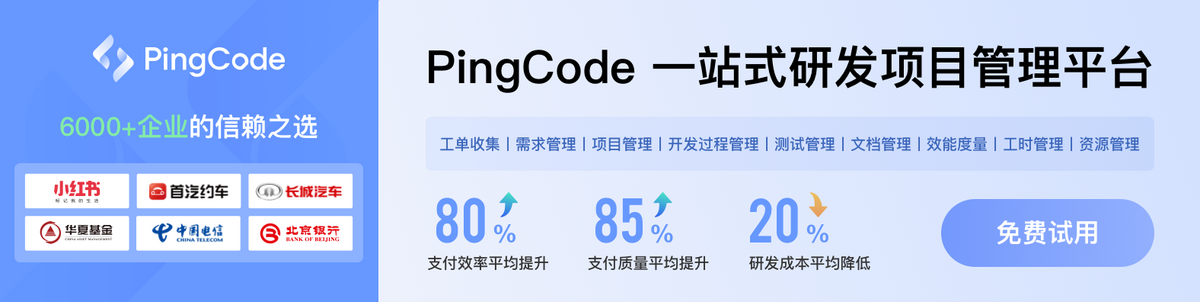