将多个文件汇总到一个文件中,主要涉及到文件的读取、内容的合并以及最终的写入操作。关键步骤包括:读取文件内容、合并内容、写入新文件。这些操作可以通过Python的内置函数和模块如open()
、os
和glob
轻松实现。下面将详细介绍如何使用Python实现这一操作。
一、读取文件内容
在合并多个文件之前,首先需要读取这些文件的内容。Python 提供了多种方法来读取文件内容,最常用的方式是使用 open()
函数。以下是基本步骤:
-
使用 open() 函数读取文件内容:
open()
函数用于打开文件,并返回一个文件对象。可以选择以不同模式打开文件,例如只读模式('r')、写入模式('w')和追加模式('a')。 -
读取文件内容:利用文件对象的
read()
或readlines()
方法读取文件内容。read()
方法一次性读取整个文件,而readlines()
方法按行读取文件,并返回一个列表,每个元素是文件的一行。
def read_file(file_path):
with open(file_path, 'r') as file:
return file.read()
二、合并文件内容
读取文件内容之后,下一步是将这些内容合并在一起。可以将读取的内容存储在一个列表中,然后通过字符串的拼接或列表的连接操作将内容合并。
-
存储文件内容:使用一个列表来存储每个文件的内容。
-
合并内容:可以使用字符串的
join()
方法,将列表中的内容合并成一个字符串。
def merge_files(file_paths):
contents = []
for file_path in file_paths:
contents.append(read_file(file_path))
return '\n'.join(contents)
三、写入新文件
最后,将合并后的内容写入一个新的文件。使用 open()
函数以写入模式('w')打开目标文件,并利用文件对象的 write()
方法将内容写入文件。
-
打开目标文件:使用
open()
函数以写入模式打开文件。如果文件不存在,会自动创建。 -
写入内容:使用文件对象的
write()
方法将合并后的内容写入目标文件。
def write_to_file(content, output_file_path):
with open(output_file_path, 'w') as file:
file.write(content)
四、综合示例
结合以上步骤,可以编写一个完整的示例,将多个文件的内容合并到一个新文件中。
import os
import glob
def read_file(file_path):
with open(file_path, 'r') as file:
return file.read()
def merge_files(file_paths):
contents = []
for file_path in file_paths:
contents.append(read_file(file_path))
return '\n'.join(contents)
def write_to_file(content, output_file_path):
with open(output_file_path, 'w') as file:
file.write(content)
def main(input_directory, output_file_path):
file_paths = glob.glob(os.path.join(input_directory, '*'))
merged_content = merge_files(file_paths)
write_to_file(merged_content, output_file_path)
if __name__ == "__main__":
main('path/to/input/directory', 'path/to/output/file.txt')
五、文件操作的最佳实践
-
异常处理:在文件操作过程中,可能会遇到各种异常情况,例如文件不存在、权限不足等。为了提高代码的健壮性,应在文件操作时进行异常处理。
-
路径处理:在处理文件路径时,建议使用
os.path
模块中的方法,例如os.path.join()
和os.path.exists()
,以确保路径的正确性和平台的兼容性。 -
批量处理:如果需要处理大量文件,可以考虑使用多线程或多进程,以提高处理效率。
import os
import glob
import threading
def read_file(file_path):
try:
with open(file_path, 'r') as file:
return file.read()
except Exception as e:
print(f"Error reading {file_path}: {e}")
return ""
def merge_files(file_paths):
contents = []
for file_path in file_paths:
contents.append(read_file(file_path))
return '\n'.join(contents)
def write_to_file(content, output_file_path):
try:
with open(output_file_path, 'w') as file:
file.write(content)
except Exception as e:
print(f"Error writing to {output_file_path}: {e}")
def main(input_directory, output_file_path):
file_paths = glob.glob(os.path.join(input_directory, '*'))
merged_content = merge_files(file_paths)
write_to_file(merged_content, output_file_path)
if __name__ == "__main__":
main('path/to/input/directory', 'path/to/output/file.txt')
六、使用多线程处理
为了提高处理效率,可以使用多线程来并行读取文件。
import os
import glob
from concurrent.futures import ThreadPoolExecutor
def read_file(file_path):
try:
with open(file_path, 'r') as file:
return file.read()
except Exception as e:
print(f"Error reading {file_path}: {e}")
return ""
def merge_files(file_paths):
with ThreadPoolExecutor() as executor:
contents = list(executor.map(read_file, file_paths))
return '\n'.join(contents)
def write_to_file(content, output_file_path):
try:
with open(output_file_path, 'w') as file:
file.write(content)
except Exception as e:
print(f"Error writing to {output_file_path}: {e}")
def main(input_directory, output_file_path):
file_paths = glob.glob(os.path.join(input_directory, '*'))
merged_content = merge_files(file_paths)
write_to_file(merged_content, output_file_path)
if __name__ == "__main__":
main('path/to/input/directory', 'path/to/output/file.txt')
通过上述步骤,可以高效地将多个文件的内容合并到一个新文件中。无论是处理少量文件还是大量文件,这种方法都能满足需求。希望这些内容能对你有所帮助。
相关问答FAQs:
如何在Python中读取多个文件并将其内容合并成一个文件?
在Python中,可以使用内置的文件操作功能读取多个文件的内容并将其写入一个新文件。常见的方法是使用open()
函数逐个打开文件,读取内容后再写入到目标文件中。可以使用with
语句确保文件在操作后正确关闭。以下是一个简单的示例代码:
file_names = ['file1.txt', 'file2.txt', 'file3.txt'] # 要合并的文件列表
with open('merged_file.txt', 'w') as outfile:
for fname in file_names:
with open(fname) as infile:
outfile.write(infile.read())
这个代码会将file1.txt
,file2.txt
和file3.txt
的内容合并到merged_file.txt
中。
在合并文件时应该注意哪些事项?
在合并多个文件时,确保文件的格式一致非常重要。如果文件包含不同类型的数据,可能会导致合并后内容混乱。此外,要处理文件路径的问题,确保所有文件路径都是正确的。还需要考虑文件的编码格式,确保使用相同的编码以避免出现乱码。
可以使用哪些库来简化文件合并的过程?
除了使用内置的文件操作功能,Python的pandas
库也可以轻松处理文件合并,尤其是当文件是表格格式时。使用pandas
的concat()
函数可以快速将多个CSV文件合并为一个DataFrame,并导出为新的CSV文件。示例代码如下:
import pandas as pd
files = ['file1.csv', 'file2.csv', 'file3.csv']
dataframes = [pd.read_csv(file) for file in files]
merged_df = pd.concat(dataframes)
merged_df.to_csv('merged_file.csv', index=False)
这种方法在处理数据时更为高效,尤其是当文件数量较多时。
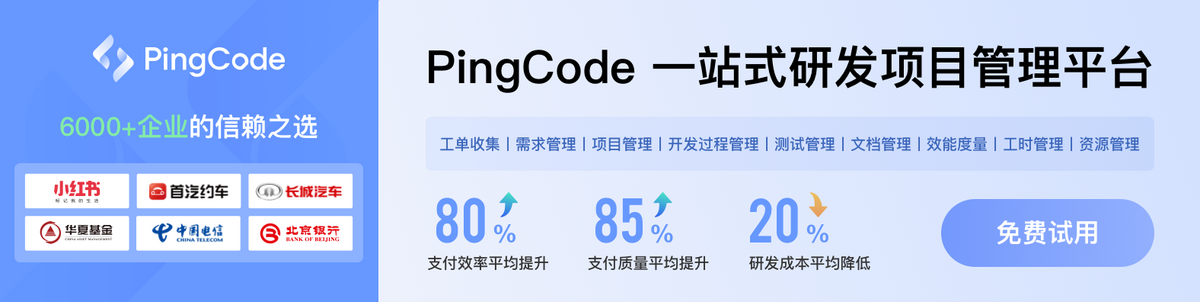