要用Python运行一个文件夹中的所有Python文件,可以通过以下几种方法:使用os模块遍历文件夹、使用subprocess模块执行文件、结合异常处理保证连续执行。 其中,使用os模块遍历文件夹是一种常见的方法,通过这个方法可以轻松地获取文件夹中的所有Python文件,然后再逐一运行它们。
一、使用os模块遍历文件夹
os模块是Python标准库的一部分,提供了与操作系统进行交互的功能。使用os模块可以轻松地遍历文件夹并获取其中的所有文件。
1. 获取文件列表
首先,我们需要获取文件夹中的所有文件。可以使用os.listdir()函数来获取文件夹中的文件列表。
import os
def get_python_files(directory):
files = os.listdir(directory)
python_files = [f for f in files if f.endswith('.py')]
return python_files
2. 运行Python文件
获取文件列表后,我们可以使用os.system()函数来运行这些Python文件。os.system()函数可以执行操作系统命令,包括运行Python脚本。
import os
def run_python_files(directory):
python_files = get_python_files(directory)
for python_file in python_files:
file_path = os.path.join(directory, python_file)
os.system(f'python {file_path}')
二、使用subprocess模块执行文件
subprocess模块提供了更加强大的功能来创建和管理子进程。相比于os.system(),subprocess模块更灵活、更安全。
1. 运行单个Python文件
使用subprocess.run()函数可以执行单个Python文件,并且可以捕获输出和错误信息。
import subprocess
def run_python_file(file_path):
result = subprocess.run(['python', file_path], capture_output=True, text=True)
print(result.stdout)
if result.stderr:
print(result.stderr)
2. 运行多个Python文件
可以结合os模块和subprocess模块,遍历文件夹并运行其中的所有Python文件。
import os
import subprocess
def run_python_files(directory):
python_files = get_python_files(directory)
for python_file in python_files:
file_path = os.path.join(directory, python_file)
run_python_file(file_path)
三、结合异常处理保证连续执行
在运行多个Python文件时,如果其中一个文件出现错误,可能会导致程序中断。为了保证即使某个文件出错,其他文件仍能继续执行,可以使用try-except语句进行异常处理。
import os
import subprocess
def run_python_file(file_path):
try:
result = subprocess.run(['python', file_path], capture_output=True, text=True)
print(result.stdout)
if result.stderr:
print(result.stderr)
except Exception as e:
print(f'Error running {file_path}: {e}')
def run_python_files(directory):
python_files = get_python_files(directory)
for python_file in python_files:
file_path = os.path.join(directory, python_file)
run_python_file(file_path)
四、处理文件夹中的子文件夹
如果文件夹中包含子文件夹,并且子文件夹中也有Python文件需要运行,可以递归处理这些子文件夹。
1. 获取所有Python文件,包括子文件夹中的文件
可以使用os.walk()函数遍历文件夹及其子文件夹。
import os
def get_all_python_files(directory):
python_files = []
for root, dirs, files in os.walk(directory):
for file in files:
if file.endswith('.py'):
python_files.append(os.path.join(root, file))
return python_files
2. 运行所有Python文件
获取所有Python文件后,可以使用前面介绍的方法逐一运行它们。
import subprocess
def run_python_file(file_path):
try:
result = subprocess.run(['python', file_path], capture_output=True, text=True)
print(result.stdout)
if result.stderr:
print(result.stderr)
except Exception as e:
print(f'Error running {file_path}: {e}')
def run_all_python_files(directory):
python_files = get_all_python_files(directory)
for python_file in python_files:
run_python_file(python_file)
五、优化和扩展
1. 并行执行Python文件
如果文件夹中的Python文件较多,可以考虑并行执行这些文件以提高效率。可以使用concurrent.futures模块来实现并行执行。
import os
import subprocess
from concurrent.futures import ThreadPoolExecutor
def run_python_file(file_path):
try:
result = subprocess.run(['python', file_path], capture_output=True, text=True)
print(result.stdout)
if result.stderr:
print(result.stderr)
except Exception as e:
print(f'Error running {file_path}: {e}')
def run_all_python_files_parallel(directory):
python_files = get_all_python_files(directory)
with ThreadPoolExecutor() as executor:
executor.map(run_python_file, python_files)
2. 记录执行日志
在运行多个Python文件时,记录每个文件的执行日志可以帮助排查问题。可以将输出和错误信息写入日志文件。
import os
import subprocess
from concurrent.futures import ThreadPoolExecutor
def run_python_file(file_path, log_file):
try:
result = subprocess.run(['python', file_path], capture_output=True, text=True)
with open(log_file, 'a') as f:
f.write(f'Running {file_path}\n')
f.write(result.stdout)
if result.stderr:
f.write(result.stderr)
except Exception as e:
with open(log_file, 'a') as f:
f.write(f'Error running {file_path}: {e}\n')
def run_all_python_files_parallel(directory, log_file):
python_files = get_all_python_files(directory)
with ThreadPoolExecutor() as executor:
executor.map(run_python_file, python_files, [log_file]*len(python_files))
六、总结
用Python运行一个文件夹中的所有Python文件,可以使用os模块遍历文件夹、使用subprocess模块执行文件,结合异常处理保证连续执行。为了处理文件夹中的子文件夹,可以使用递归方法获取所有Python文件,并使用并行执行和记录执行日志等方法进行优化和扩展。通过以上方法,可以高效地运行一个文件夹中的所有Python文件,并记录执行过程中的输出和错误信息。这些方法不仅适用于简单的脚本执行,还可以扩展到更复杂的应用场景,如自动化测试、批量数据处理等。
相关问答FAQs:
如何用Python批量运行文件夹中的所有Python脚本?
可以使用os
模块遍历文件夹中的所有文件,并结合subprocess
模块来运行每个Python脚本。以下是一个简单的示例代码:
import os
import subprocess
folder_path = 'your_folder_path' # 替换为你的文件夹路径
for file_name in os.listdir(folder_path):
if file_name.endswith('.py'):
full_path = os.path.join(folder_path, file_name)
subprocess.run(['python', full_path])
该代码将遍历指定文件夹中的所有.py文件,并依次运行它们。
在Python中如何处理运行脚本时可能出现的错误?
为了处理运行时错误,可以在调用脚本时使用try-except
语句来捕获异常。以下是一个示例:
try:
subprocess.run(['python', full_path], check=True)
except subprocess.CalledProcessError as e:
print(f"运行 {file_name} 时出错: {e}")
这样,如果某个脚本在运行时出现错误,程序不会终止,而是会输出错误信息,便于后续的调试。
如何在Python中设置文件夹路径为动态输入?
可以使用input()
函数让用户在运行程序时输入文件夹路径。示例代码如下:
folder_path = input("请输入要运行的Python文件夹路径: ")
这样,用户在执行脚本时可以动态指定要处理的文件夹,提高了脚本的灵活性。
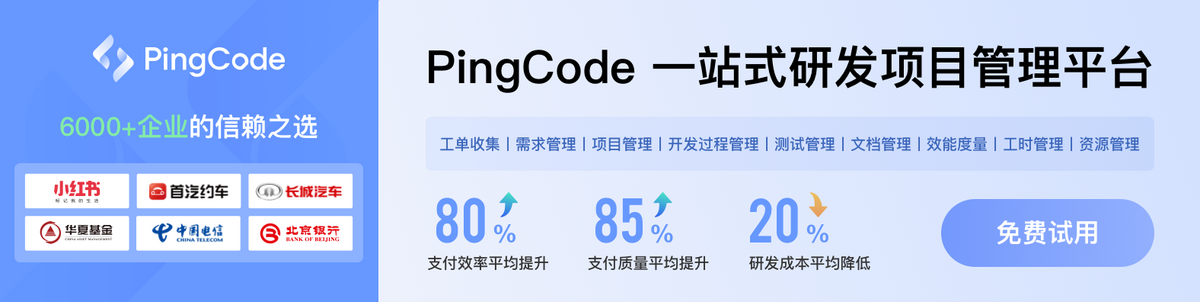