Python如何同时输入一组数据库
在Python中,使用多线程、利用批量插入、使用事务、优化SQL语句是实现同时输入一组数据库的有效方法。多线程可以显著提高数据插入的效率。我们可以通过Python的threading
模块创建多个线程,并将数据插入任务分配给这些线程,从而实现并发插入。下面将详细介绍多线程的实现方法。
一、多线程
什么是多线程
多线程是一种并发执行多个任务的技术。在Python中,多线程可以通过threading
模块来实现。多线程技术的优点在于可以有效利用多核CPU的处理能力,提高程序的运行效率。
如何在Python中实现多线程插入数据库
- 导入必要的模块
import threading
import psycopg2
- 定义线程类
class DatabaseThread(threading.Thread):
def __init__(self, conn_params, data):
threading.Thread.__init__(self)
self.conn_params = conn_params
self.data = data
def run(self):
conn = psycopg2.connect(self.conn_params)
cursor = conn.cursor()
for record in self.data:
cursor.execute("INSERT INTO table_name (column1, column2) VALUES (%s, %s)", record)
conn.commit()
cursor.close()
conn.close()
- 启动多个线程
conn_params = {
'dbname': 'your_db',
'user': 'your_user',
'password': 'your_password',
'host': 'your_host',
'port': 'your_port'
}
data_chunks = [chunk1, chunk2, chunk3] # 分块后的数据
threads = []
for chunk in data_chunks:
thread = DatabaseThread(conn_params, chunk)
thread.start()
threads.append(thread)
for thread in threads:
thread.join()
二、利用批量插入
什么是批量插入
批量插入是指一次性插入多条记录,这样可以减少数据库连接的开销,提高数据插入的效率。
如何在Python中实现批量插入
- 使用
executemany
方法
import psycopg2
conn = psycopg2.connect(conn_params)
cursor = conn.cursor()
data = [(val1, val2), (val3, val4), (val5, val6)]
cursor.executemany("INSERT INTO table_name (column1, column2) VALUES (%s, %s)", data)
conn.commit()
cursor.close()
conn.close()
- 使用
COPY
命令
with open('data.csv', 'w') as f:
for record in data:
f.write(','.join(map(str, record)) + '\n')
conn = psycopg2.connect(conn_params)
cursor = conn.cursor()
with open('data.csv', 'r') as f:
cursor.copy_from(f, 'table_name', sep=',')
conn.commit()
cursor.close()
conn.close()
三、使用事务
什么是事务
事务是一组操作的集合,要么全部成功,要么全部失败。使用事务可以保证数据的一致性和完整性。
如何在Python中使用事务
- 开启事务
conn = psycopg2.connect(conn_params)
cursor = conn.cursor()
try:
cursor.execute("BEGIN")
for record in data:
cursor.execute("INSERT INTO table_name (column1, column2) VALUES (%s, %s)", record)
cursor.execute("COMMIT")
except Exception as e:
cursor.execute("ROLLBACK")
print(f"Error: {e}")
finally:
cursor.close()
conn.close()
四、优化SQL语句
为什么需要优化SQL语句
优化SQL语句可以显著提高数据库的性能,减少数据插入的时间。
如何优化SQL语句
- 使用预编译语句
conn = psycopg2.connect(conn_params)
cursor = conn.cursor()
stmt = "INSERT INTO table_name (column1, column2) VALUES (%s, %s)"
data = [(val1, val2), (val3, val4), (val5, val6)]
cursor.executemany(stmt, data)
conn.commit()
cursor.close()
conn.close()
- 减少不必要的索引
在插入大量数据之前,可以暂时删除不必要的索引,等数据插入完成后再重新创建索引。这可以显著提高插入效率。
-- 删除索引
DROP INDEX IF EXISTS index_name;
-- 插入数据
-- 重新创建索引
CREATE INDEX index_name ON table_name (column1);
- 分批次插入
如果数据量非常大,可以将数据分成多个批次进行插入,这样可以减少单次插入的数据量,降低数据库的压力。
batch_size = 1000
for i in range(0, len(data), batch_size):
batch = data[i:i + batch_size]
cursor.executemany("INSERT INTO table_name (column1, column2) VALUES (%s, %s)", batch)
五、数据库连接池
什么是数据库连接池
数据库连接池是一种用于管理数据库连接的技术,可以有效地复用数据库连接,减少连接的创建和销毁的开销。
如何在Python中使用数据库连接池
- 安装
psycopg2
和psycopg2_pool
pip install psycopg2 psycopg2_pool
- 创建连接池
from psycopg2 import pool
conn_params = {
'dbname': 'your_db',
'user': 'your_user',
'password': 'your_password',
'host': 'your_host',
'port': 'your_port'
}
connection_pool = psycopg2.pool.SimpleConnectionPool(1, 20, conn_params)
- 使用连接池
conn = connection_pool.getconn()
cursor = conn.cursor()
try:
for record in data:
cursor.execute("INSERT INTO table_name (column1, column2) VALUES (%s, %s)", record)
conn.commit()
finally:
cursor.close()
connection_pool.putconn(conn)
六、错误处理与日志记录
为什么需要错误处理与日志记录
在批量插入数据的过程中,可能会遇到各种各样的错误。通过错误处理和日志记录,可以及时发现和解决问题,保证数据插入的顺利进行。
如何在Python中实现错误处理与日志记录
- 使用
try-except
进行错误处理
try:
cursor.execute("INSERT INTO table_name (column1, column2) VALUES (%s, %s)", record)
except Exception as e:
print(f"Error: {e}")
logging.error(f"Error: {e}")
- 使用
logging
模块记录日志
import logging
logging.basicConfig(filename='database.log', level=logging.ERROR)
try:
cursor.execute("INSERT INTO table_name (column1, column2) VALUES (%s, %s)", record)
except Exception as e:
logging.error(f"Error: {e}")
七、数据验证与清洗
为什么需要数据验证与清洗
在插入数据之前,需要对数据进行验证和清洗,确保数据的准确性和一致性。这样可以避免由于数据问题导致的插入失败或数据错误。
如何在Python中进行数据验证与清洗
- 数据验证
def validate_data(record):
if not isinstance(record[0], int):
return False
if not isinstance(record[1], str):
return False
return True
valid_data = [record for record in data if validate_data(record)]
- 数据清洗
def clean_data(record):
return (record[0], record[1].strip().lower())
cleaned_data = [clean_data(record) for record in valid_data]
八、数据库备份与恢复
为什么需要数据库备份与恢复
在进行批量数据插入之前,进行数据库备份是非常重要的。这样可以在出现问题时,及时恢复数据,避免数据丢失。
如何在Python中进行数据库备份与恢复
- 数据库备份
import subprocess
backup_cmd = "pg_dump -U your_user -h your_host your_db > backup.sql"
subprocess.run(backup_cmd, shell=True, check=True)
- 数据库恢复
restore_cmd = "psql -U your_user -h your_host your_db < backup.sql"
subprocess.run(restore_cmd, shell=True, check=True)
九、性能监控与优化
为什么需要性能监控与优化
在进行批量数据插入的过程中,对性能进行监控与优化,可以及时发现性能瓶颈,并进行相应的优化,提高插入效率。
如何在Python中进行性能监控与优化
- 使用
time
模块进行性能监控
import time
start_time = time.time()
数据插入操作
end_time = time.time()
print(f"Time taken: {end_time - start_time} seconds")
- 使用数据库自带的性能监控工具
大多数数据库都自带性能监控工具,如PostgreSQL的
pg_stat_activity
视图,可以通过查询这些视图获取性能数据。
SELECT * FROM pg_stat_activity;
- 优化数据库配置
根据性能监控的数据,可以对数据库配置进行优化,如调整缓存大小、增加连接数等。
ALTER SYSTEM SET shared_buffers = '256MB';
ALTER SYSTEM SET max_connections = '200';
通过以上方法,我们可以在Python中实现高效的批量数据插入。在实际应用中,可以根据具体的需求和数据规模,选择合适的方法和策略,保证数据插入的效率和可靠性。
相关问答FAQs:
在Python中,如何同时连接多个数据库?
在Python中,可以使用多种数据库连接库,如sqlite3
、MySQLdb
、psycopg2
等。要同时连接多个数据库,可以为每个数据库实例化一个连接对象,然后使用多个线程或异步编程来处理数据交互。确保各个连接的配置参数(如用户名、密码、数据库名等)正确无误。
如何在Python中批量插入数据到多个数据库表?
批量插入数据通常可以通过使用事务来提高效率。在Python中,可以通过使用executemany()
方法来将多条记录一次性插入到数据库表中。确保在插入数据之前,已经建立好连接并选择了正确的数据库。对于多个数据库,可以循环遍历每个数据库的连接,逐个执行插入操作。
如果在同时输入多个数据库时发生错误,该如何处理?
在同时进行多个数据库操作时,可能会遇到连接超时、数据格式不匹配等问题。可以使用异常处理机制来捕捉这些错误。例如,使用try-except
语句块来捕获异常,并在发生错误时进行适当的回滚操作。同时,记录日志可以帮助你追踪问题的来源。
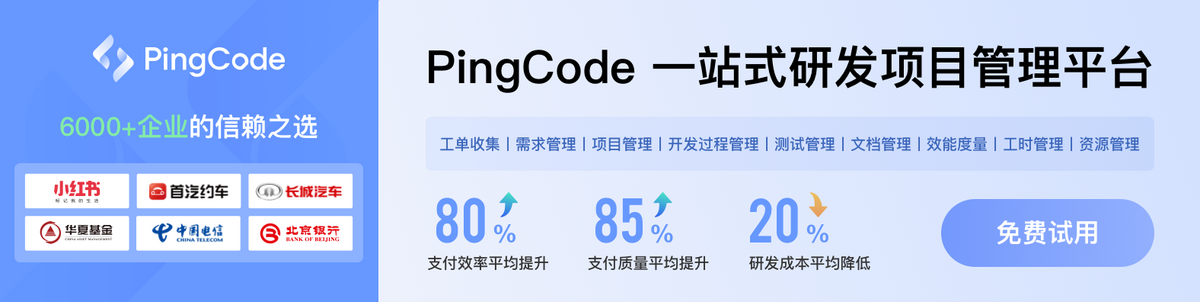