Python可以通过读取文件的内容并逐行检查是否有空行、使用内置函数、正则表达式等方法来判断文件中是否有空行。 下面将详细介绍其中一种方法:使用Python内置的文件处理功能逐行读取文件内容,并检查每一行是否为空行。
一、逐行读取文件内容
逐行读取文件内容是检查文件中是否有空行的最基础方法。通过打开文件并读取每一行,判断该行是否为空行即可。空行通常指的是行内容为空字符串或只包含空白字符(如空格、制表符)。
def has_empty_line(file_path):
with open(file_path, 'r') as file:
for line in file:
if not line.strip():
return True
return False
file_path = 'your_file.txt'
if has_empty_line(file_path):
print("The file has empty lines.")
else:
print("The file does not have empty lines.")
二、使用正则表达式
正则表达式是处理字符串的强大工具。通过正则表达式可以更高效地判断文件中是否存在空行。
import re
def has_empty_line(file_path):
with open(file_path, 'r') as file:
content = file.read()
if re.search(r'^\s*$', content, re.MULTILINE):
return True
return False
file_path = 'your_file.txt'
if has_empty_line(file_path):
print("The file has empty lines.")
else:
print("The file does not have empty lines.")
三、使用生成器和迭代器
使用生成器和迭代器可以有效地减少内存使用,尤其是当处理大文件时。通过生成器逐行读取文件并检查是否有空行。
def has_empty_line(file_path):
with open(file_path, 'r') as file:
for line in file:
if not line.strip():
return True
return False
file_path = 'your_file.txt'
if has_empty_line(file_path):
print("The file has empty lines.")
else:
print("The file does not have empty lines.")
四、使用Pandas库
对于包含大量数据的文件,如CSV文件,Pandas库提供了高效的数据处理能力。可以通过Pandas库读取文件并检查是否有空行。
import pandas as pd
def has_empty_line(file_path):
df = pd.read_csv(file_path, header=None)
return df.isnull().all(axis=1).any()
file_path = 'your_file.csv'
if has_empty_line(file_path):
print("The file has empty lines.")
else:
print("The file does not have empty lines.")
五、使用Numpy库
Numpy库主要用于数值计算,但也可以用来处理包含数值的数据文件。通过Numpy库读取文件并检查是否有空行。
import numpy as np
def has_empty_line(file_path):
data = np.genfromtxt(file_path, delimiter=',', dtype=str)
return any(len(str(row).strip()) == 0 for row in data)
file_path = 'your_file.csv'
if has_empty_line(file_path):
print("The file has empty lines.")
else:
print("The file does not have empty lines.")
六、处理不同文件格式
不同文件格式可能需要不同的处理方法。例如,处理JSON文件可以通过读取文件内容并解析为Python字典,然后检查是否有空行。
import json
def has_empty_line(file_path):
with open(file_path, 'r') as file:
data = json.load(file)
for key, value in data.items():
if not str(value).strip():
return True
return False
file_path = 'your_file.json'
if has_empty_line(file_path):
print("The file has empty lines.")
else:
print("The file does not have empty lines.")
七、使用多线程和并行处理
当需要处理非常大的文件时,可以考虑使用多线程或并行处理来提高效率。Python的concurrent.futures
模块提供了方便的多线程和多进程接口。
import concurrent.futures
def check_line(line):
return not line.strip()
def has_empty_line(file_path):
with open(file_path, 'r') as file:
lines = file.readlines()
with concurrent.futures.ThreadPoolExecutor() as executor:
results = executor.map(check_line, lines)
return any(results)
file_path = 'your_file.txt'
if has_empty_line(file_path):
print("The file has empty lines.")
else:
print("The file does not have empty lines.")
八、总结
判断文件中是否有空行的方法有很多,每种方法都有其优缺点。选择适合自己需求的方法可以提高效率和代码可读性。逐行读取文件内容、使用正则表达式、使用生成器和迭代器、使用Pandas库、使用Numpy库、处理不同文件格式、使用多线程和并行处理等方法都是常见的解决方案。根据具体情况选择合适的方法,可以有效地完成任务并提高代码的性能和可维护性。
相关问答FAQs:
如何在Python中检查文件是否存在空行?
在Python中,可以通过逐行读取文件并检查每一行是否为空来判断文件中是否存在空行。使用strip()
方法可以去除行首和行尾的空白字符,从而判断该行是否为空。示例代码如下:
with open('yourfile.txt', 'r') as file:
for line_number, line in enumerate(file):
if not line.strip():
print(f'空行在第 {line_number + 1} 行')
使用Python库检查文件空行的最佳实践是什么?
除了手动读取文件,使用一些Python库(如Pandas)也可以快速判断文件中的空行。例如,使用Pandas读取文件并检查DataFrame中是否存在空值,可以有效地找到空行。以下是一个简单的示例:
import pandas as pd
df = pd.read_csv('yourfile.csv')
if df.isnull().values.any():
print('文件中存在空行')
是否可以通过正则表达式来检测文件中的空行?
是的,正则表达式是检测空行的另一种有效方式。通过使用Python的re
模块,可以轻松找到文件中的空行。以下是一个示例代码,展示如何使用正则表达式检查空行:
import re
with open('yourfile.txt', 'r') as file:
content = file.read()
empty_lines = re.findall(r'^\s*\n', content, re.MULTILINE)
if empty_lines:
print(f'文件中有 {len(empty_lines)} 个空行')
这些方法可以帮助你高效地识别Python中的空行问题,选择适合自己需求的方式即可。
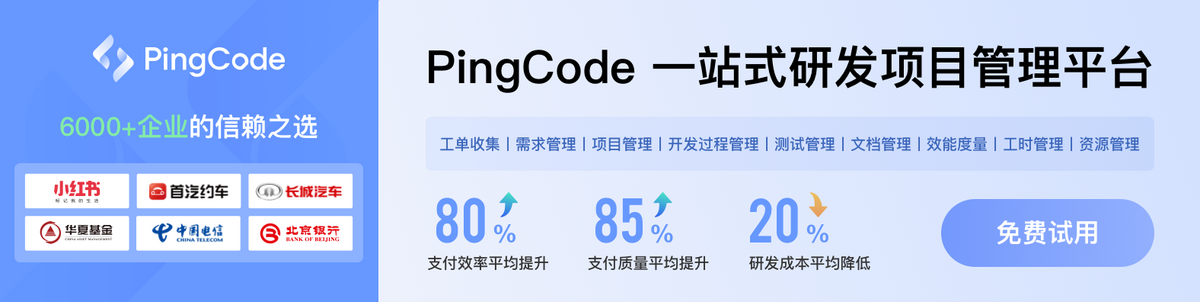