Python在检索一个字母的方法包括使用字符串方法、正则表达式、以及列表解析等。 使用字符串方法如.find()
、.index()
可以轻松定位字母的位置;正则表达式提供了更灵活的匹配方式;列表解析则可以进行更复杂的条件检索。本文将详细探讨这些方法,帮助你更好地理解和应用Python进行字母检索。
一、字符串方法
在Python中,字符串方法是检索特定字母的最直接方式。以下是一些常用的方法。
1.1 .find()
方法
.find()
方法用于返回指定子字符串在字符串中首次出现的位置。如果找不到,则返回 -1。
text = "Hello, World!"
position = text.find('o')
print(position) # 输出:4
1.2 .index()
方法
.index()
方法与 .find()
类似,但如果找不到子字符串,会引发 ValueError
。
text = "Hello, World!"
try:
position = text.index('o')
print(position) # 输出:4
except ValueError:
print("Not Found")
1.3 .rfind()
方法
.rfind()
方法用于从右向左搜索字符串,返回最后一次出现的位置。
text = "Hello, World!"
position = text.rfind('o')
print(position) # 输出:8
1.4 .count()
方法
.count()
方法用于返回指定子字符串在字符串中出现的次数。
text = "Hello, World!"
count = text.count('o')
print(count) # 输出:2
二、正则表达式
正则表达式是一种强大的字符串匹配工具,Python 的 re
模块提供了对正则表达式的支持。
2.1 re.search()
方法
re.search()
方法用于搜索字符串中首次出现的匹配项,返回一个匹配对象。
import re
text = "Hello, World!"
match = re.search('o', text)
if match:
print(match.start()) # 输出:4
else:
print("Not Found")
2.2 re.findall()
方法
re.findall()
方法用于返回所有匹配的子字符串列表。
matches = re.findall('o', text)
print(matches) # 输出:['o', 'o']
2.3 re.finditer()
方法
re.finditer()
方法返回一个迭代器,产生匹配对象。
for match in re.finditer('o', text):
print(match.start()) # 输出:4 和 8
三、列表解析
列表解析是Python中一个非常强大的功能,能够在一行代码中完成复杂的操作。
3.1 基本列表解析
通过列表解析,我们可以在字符串中查找所有出现特定字母的位置。
text = "Hello, World!"
positions = [i for i, letter in enumerate(text) if letter == 'o']
print(positions) # 输出:[4, 8]
3.2 条件列表解析
我们还可以通过条件列表解析来筛选出满足特定条件的字母。
text = "Hello, World!"
positions = [i for i, letter in enumerate(text) if letter in 'aeiou']
print(positions) # 输出:[1, 4, 7]
四、综合应用
在实际应用中,我们通常需要结合多种方法来实现复杂的字母检索任务。
4.1 字母统计
统计字符串中每个字母出现的次数,可以使用 collections.Counter
。
from collections import Counter
text = "Hello, World!"
counter = Counter(text)
print(counter) # 输出:Counter({'l': 3, 'o': 2, 'H': 1, 'e': 1, ' ': 1, 'W': 1, 'r': 1, 'd': 1, '!': 1})
4.2 多条件筛选
结合字符串方法和列表解析,可以实现更复杂的多条件筛选。
text = "Hello, World!"
positions = [i for i, letter in enumerate(text) if letter in 'aeiou' and i % 2 == 0]
print(positions) # 输出:[4]
五、性能优化
在处理大数据时,性能是一个需要重点考虑的问题。以下是一些优化建议。
5.1 使用生成器
生成器比列表解析更节省内存,适合处理大数据。
text = "Hello, World!"
positions = (i for i, letter in enumerate(text) if letter == 'o')
for pos in positions:
print(pos) # 输出:4 和 8
5.2 分段处理
对于超大字符串,可以将其分段处理,以减少内存占用。
def find_letter_in_chunks(text, letter, chunk_size=1000):
positions = []
for start in range(0, len(text), chunk_size):
chunk = text[start:start+chunk_size]
positions.extend([start + i for i, char in enumerate(chunk) if char == letter])
return positions
text = "Hello, World!" * 1000
positions = find_letter_in_chunks(text, 'o')
print(positions)
六、实战案例
6.1 文本分析
在文本分析中,字母检索是一个基本但非常重要的步骤。以下是一个简单的文本分析案例。
text = """
Natural language processing (NLP) is a field of artificial intelligence that focuses on the interaction between computers and humans through natural language. The ultimate goal of NLP is to enable computers to understand, interpret, and generate human language in a way that is both meaningful and useful.
"""
统计每个字母出现的次数
counter = Counter(text.lower())
print(counter)
查找特定字母的位置
positions = [i for i, letter in enumerate(text) if letter == 'a']
print(positions)
6.2 数据清洗
在数据清洗过程中,字母检索可以帮助我们过滤掉不需要的字符。
text = "Hello123, World!@#"
只保留字母
cleaned_text = ''.join([char for char in text if char.isalpha()])
print(cleaned_text) # 输出:HelloWorld
七、总结
Python提供了多种方法来检索字符串中的字母,包括字符串方法、正则表达式和列表解析等。通过灵活运用这些方法,我们可以高效地完成各种字母检索任务。在实际应用中,结合多种方法和性能优化技术,可以更好地应对复杂的字母检索需求。
希望这篇文章能帮助你更好地理解和应用Python进行字母检索。如果你有任何疑问或需要进一步的帮助,请随时留言交流。
相关问答FAQs:
如何在Python中检索字符串中的特定字母?
在Python中,可以使用字符串的内置方法,如in
关键字,来检查一个字母是否存在于字符串中。例如,if 'a' in 'apple':
会返回True,表示字母'a'在'apple'中存在。此外,str.find()
和str.index()
方法也可以用来找到字母的位置,前者返回-1表示未找到,而后者则会抛出异常。
在Python中如何获取字母出现的所有位置?
要获取特定字母在字符串中所有出现的位置,可以使用循环配合str.find()
或enumerate()
。例如,通过遍历字符串并记录每次匹配的索引,可以得到字母的所有位置。这种方法非常有效,尤其是处理长字符串时。
如何使用正则表达式在Python中检索字母?
Python的re
模块提供了强大的正则表达式功能,可以用来进行复杂的字母检索。通过re.search()
或re.findall()
方法,可以找到字母的匹配项。这种方法不仅可以检索单个字母,还可以根据模式匹配多个字母或组合,提高了检索的灵活性和效率。
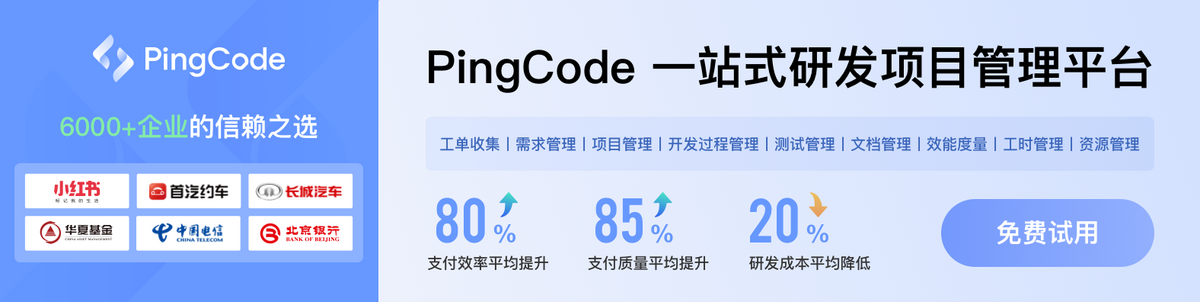