如何用Python从微信保存图片大小
在微信中,保存图片大小涉及几个重要步骤:获取图片数据、解析图片信息、计算图片大小、并进行保存。使用Python可以通过微信的API、PIL库、os模块来实现这些功能。其中,解析图片信息和计算图片大小是关键步骤。本文将详细介绍如何使用Python实现这一过程。
一、获取微信图片数据
要从微信中获取图片数据,通常需要利用微信的API接口或者通过第三方库来获取。微信的API接口可以提供消息和媒体文件的读取功能。
1. 微信API接口
微信提供了多种API接口来获取消息和媒体文件,例如微信公众平台API、微信企业号API等。这些API接口可以用来获取消息内容和媒体文件的URL。
2. 第三方库
使用第三方库如WeChaty、itchat等,可以更方便地获取微信消息和媒体文件。
import itchat
登陆微信
itchat.login()
获取所有好友列表
friends = itchat.get_friends()
获取图片消息
@itchat.msg_register(itchat.content.PICTURE)
def download_files(msg):
msg.download(msg.fileName)
print(f"Image {msg.fileName} saved")
运行
itchat.run()
二、解析图片信息
获取图片数据后,需要解析图片信息以获取其大小。Python的PIL(Pillow)库可以方便地解析图片信息。
1. 安装Pillow库
在使用Pillow库之前,需要先安装它:
pip install Pillow
2. 读取图片信息
使用Pillow库可以读取图片的尺寸和格式等信息。
from PIL import Image
def get_image_info(image_path):
with Image.open(image_path) as img:
width, height = img.size
format = img.format
return width, height, format
示例
image_path = "example.jpg"
width, height, format = get_image_info(image_path)
print(f"Width: {width}, Height: {height}, Format: {format}")
三、计算图片大小
图片大小通常指的是文件大小,可以通过os模块获取文件的字节大小。
import os
def get_file_size(file_path):
return os.path.getsize(file_path)
示例
file_size = get_file_size("example.jpg")
print(f"File Size: {file_size} bytes")
四、保存图片和大小信息
将图片和其大小信息保存到本地文件系统中,可以选择保存为文本文件或数据库。
1. 保存为文本文件
def save_image_info(image_path, info):
with open("image_info.txt", "a") as file:
file.write(f"Image: {image_path}, Info: {info}\n")
示例
image_info = f"Width: {width}, Height: {height}, Format: {format}, Size: {file_size} bytes"
save_image_info(image_path, image_info)
2. 保存到数据库
使用SQLite数据库保存图片信息,可以更方便地进行查询和管理。
import sqlite3
def save_image_info_db(image_path, width, height, format, file_size):
conn = sqlite3.connect('image_info.db')
cursor = conn.cursor()
cursor.execute('''CREATE TABLE IF NOT EXISTS images
(path TEXT, width INTEGER, height INTEGER, format TEXT, size INTEGER)''')
cursor.execute("INSERT INTO images (path, width, height, format, size) VALUES (?, ?, ?, ?, ?)",
(image_path, width, height, format, file_size))
conn.commit()
conn.close()
示例
save_image_info_db(image_path, width, height, format, file_size)
五、处理批量图片
在实际应用中,可能需要处理批量图片。可以通过循环来处理多个图片文件。
import glob
def process_images(image_folder):
for image_path in glob.glob(f"{image_folder}/*.jpg"):
width, height, format = get_image_info(image_path)
file_size = get_file_size(image_path)
save_image_info_db(image_path, width, height, format, file_size)
示例
process_images("/path/to/image/folder")
六、优化和异常处理
在处理图片时,需要考虑优化和异常处理,以确保代码的鲁棒性和效率。
1. 异常处理
处理文件读取和数据库操作时,可能会遇到各种异常,需要进行捕获和处理。
def get_image_info(image_path):
try:
with Image.open(image_path) as img:
width, height = img.size
format = img.format
return width, height, format
except Exception as e:
print(f"Error reading image {image_path}: {e}")
return None, None, None
def save_image_info_db(image_path, width, height, format, file_size):
try:
conn = sqlite3.connect('image_info.db')
cursor = conn.cursor()
cursor.execute('''CREATE TABLE IF NOT EXISTS images
(path TEXT, width INTEGER, height INTEGER, format TEXT, size INTEGER)''')
cursor.execute("INSERT INTO images (path, width, height, format, size) VALUES (?, ?, ?, ?, ?)",
(image_path, width, height, format, file_size))
conn.commit()
conn.close()
except sqlite3.Error as e:
print(f"Database error: {e}")
示例
image_path = "example.jpg"
width, height, format = get_image_info(image_path)
if width and height and format:
file_size = get_file_size(image_path)
save_image_info_db(image_path, width, height, format, file_size)
2. 优化读取速度
对于大量图片,可以使用多线程或多进程来提高处理速度。
from concurrent.futures import ThreadPoolExecutor
def process_image(image_path):
width, height, format = get_image_info(image_path)
if width and height and format:
file_size = get_file_size(image_path)
save_image_info_db(image_path, width, height, format, file_size)
def process_images(image_folder):
image_paths = glob.glob(f"{image_folder}/*.jpg")
with ThreadPoolExecutor() as executor:
executor.map(process_image, image_paths)
示例
process_images("/path/to/image/folder")
七、总结
通过使用Python,可以方便地从微信中获取图片数据、解析图片信息、计算图片大小并保存。本文介绍了使用微信API接口获取图片数据,使用Pillow库解析图片信息,使用os模块计算图片大小,并将图片信息保存到本地文件或数据库的方法。同时,介绍了处理批量图片、优化和异常处理的技巧。掌握这些技巧,可以帮助你更高效地处理和管理微信中的图片数据。
相关问答FAQs:
如何使用Python处理微信保存的图片?
要处理微信保存的图片,可以使用Python的图像处理库,如PIL(Pillow)或OpenCV。通过这些库,可以轻松地打开、编辑和保存图片。首先确保安装了相关库,然后使用适当的函数读取微信保存的图片文件,进行需要的处理或分析。
在Python中如何获取图片的文件大小?
获取图片文件大小非常简单。可以使用Python内置的os
模块来获取文件的字节大小。通过os.path.getsize()
函数传入图片的路径,可以返回文件的大小(以字节为单位),从而帮助你了解图片的存储需求。
如何优化微信保存的图片以减小文件大小?
优化图片可以通过多种方式实现,例如调整分辨率、改变图片格式或压缩图片质量。在Python中,可以使用Pillow库中的Image.save()
方法,设置不同的压缩参数,或者使用Image.resize()
方法来改变图片的尺寸,从而有效减小文件大小。选择合适的优化方法可以在保持视觉质量的同时,减少文件占用的存储空间。
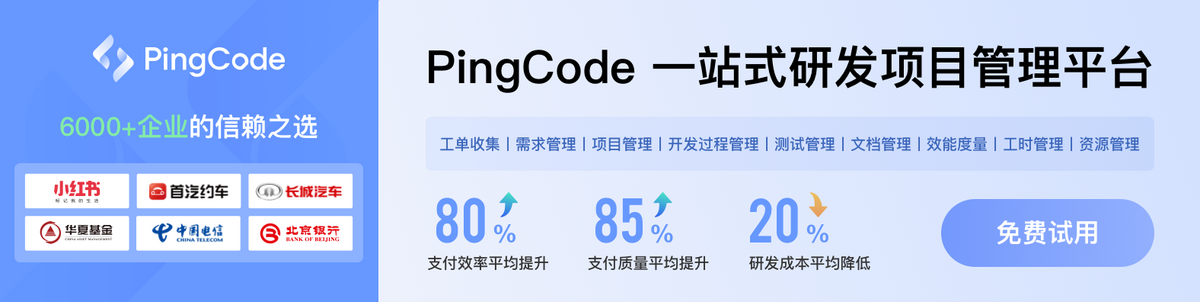