如何在 Python 里画五角星
在 Python 中绘制五角星,可以使用多个方法和库。使用 turtle 库、使用 matplotlib 库、使用 pygame 库,其中最常用和直观的方法是利用 turtle
库。Turtle
库是一个非常适合初学者的绘图工具,它提供了一个简单易用的界面,可以让你轻松地绘制各种图形。在这篇文章中,我们将重点介绍如何使用 turtle
库来绘制五角星,并详细解释每一步的操作。
一、使用 turtle 库绘制五角星
1. turtle 库简介
turtle
库是 Python 的标准库之一,专门用于绘图。它使用一个“海龟”在屏幕上移动和绘制图形。通过简单的命令,你可以控制这只海龟的方向和移动距离,从而绘制出各种图形。
2. 安装和导入 turtle 库
turtle
库是 Python 的内置库,因此无需额外安装。你只需要在代码中导入它即可:
import turtle
3. 基本绘图命令
在使用 turtle
库绘图时,有几个基本命令需要了解:
turtle.forward(distance)
: 向前移动指定距离。turtle.backward(distance)
: 向后移动指定距离。turtle.right(angle)
: 向右转动指定角度。turtle.left(angle)
: 向左转动指定角度。turtle.penup()
: 提起画笔,移动时不绘图。turtle.pendown()
: 放下画笔,开始绘图。
4. 绘制五角星的步骤
绘制五角星的关键在于每个顶点之间的角度和移动距离。五角星的每个内角是 36 度,外角是 144 度。下面是绘制五角星的代码示例:
import turtle
创建一个屏幕对象
screen = turtle.Screen()
screen.title("Draw a Star")
创建一个海龟对象
star = turtle.Turtle()
定义五角星的边长
edge_length = 100
绘制五角星
for _ in range(5):
star.forward(edge_length)
star.right(144)
隐藏海龟
star.hideturtle()
保持窗口打开,直到用户关闭
turtle.done()
在这段代码中,我们首先创建一个屏幕对象和一个海龟对象。然后,通过一个循环控制海龟前进和转动,绘制出五角星的五个边。
二、使用 matplotlib 库绘制五角星
1. matplotlib 库简介
matplotlib
是一个强大的 2D 绘图库,常用于生成图表和其他二维图形。虽然它主要用于数据可视化,但也可以用来绘制几何图形。
2. 安装和导入 matplotlib 库
如果尚未安装 matplotlib
库,可以使用以下命令进行安装:
pip install matplotlib
然后在代码中导入它:
import matplotlib.pyplot as plt
import numpy as np
3. 使用 matplotlib 绘制五角星
绘制五角星需要计算每个顶点的坐标。我们可以使用极坐标系来简化这个过程:
import matplotlib.pyplot as plt
import numpy as np
定义五角星的顶点数量
num_vertices = 5
计算每个顶点的角度
angles = np.linspace(0, 2 * np.pi, num_vertices + 1)[:num_vertices]
计算每个顶点的坐标
x_coords = np.cos(angles)
y_coords = np.sin(angles)
连接顶点形成五角星
star_coords = np.column_stack([x_coords, y_coords])
star_coords = np.vstack([star_coords, star_coords[0]])
绘制五角星
plt.figure()
plt.plot(star_coords[:, 0], star_coords[:, 1], marker='o')
plt.gca().set_aspect('equal')
plt.title('Five-Pointed Star')
plt.show()
在这段代码中,我们使用 numpy
来计算每个顶点的坐标,然后使用 matplotlib
来绘制这些顶点并连接它们形成五角星。
三、使用 pygame 库绘制五角星
1. pygame 库简介
pygame
是一个跨平台的游戏开发库,提供了图形、声音和输入处理等功能。尽管它主要用于游戏开发,但也可以用于绘制图形。
2. 安装和导入 pygame 库
如果尚未安装 pygame
库,可以使用以下命令进行安装:
pip install pygame
然后在代码中导入它:
import pygame
import math
3. 使用 pygame 绘制五角星
绘制五角星需要计算每个顶点的坐标,并使用 pygame
绘制这些顶点:
import pygame
import math
初始化 Pygame
pygame.init()
设置屏幕大小
screen = pygame.display.set_mode((400, 400))
定义颜色
WHITE = (255, 255, 255)
BLACK = (0, 0, 0)
定义五角星的顶点数量
num_vertices = 5
radius = 100
center = (200, 200)
计算每个顶点的角度
angles = [2 * math.pi * i / num_vertices for i in range(num_vertices)]
计算每个顶点的坐标
points = [(center[0] + radius * math.cos(angle), center[1] + radius * math.sin(angle)) for angle in angles]
连接顶点形成五角星
points = points + points[0:1]
主循环
running = True
while running:
for event in pygame.event.get():
if event.type == pygame.QUIT:
running = False
screen.fill(WHITE)
pygame.draw.polygon(screen, BLACK, points, 1)
pygame.display.flip()
pygame.quit()
在这段代码中,我们使用 pygame
初始化屏幕,计算五角星的顶点坐标,并在主循环中绘制五角星。
四、总结
在 Python 中绘制五角星有多种方法,最常用和直观的是利用 turtle
库。除此之外,matplotlib
和 pygame
库也可以用于绘制五角星。每种方法都有其独特的优点和适用场景,选择哪种方法取决于你的具体需求和应用场景。无论选择哪种方法,掌握这些技巧都能帮助你在 Python 中实现更多复杂的图形绘制。
相关问答FAQs:
如何在Python中绘制五角星的基本步骤是什么?
要在Python中绘制五角星,您可以使用图形库如Turtle或Matplotlib。使用Turtle库时,您需要初始化画布,设置画笔的颜色和粗细,然后通过设置角度和边长来绘制五角星的每一条边。
绘制五角星时,如何设置颜色和样式?
在使用Turtle库绘制五角星时,可以通过pencolor()
和fillcolor()
函数设置线条颜色和填充颜色。您还可以使用begin_fill()
和end_fill()
函数来填充五角星的内部颜色。此外,可以通过pensize()
函数调整画笔的粗细,以增强视觉效果。
是否有其他Python库可以绘制五角星?
除了Turtle,Matplotlib也是一个非常强大的库,可以用于绘制五角星。您可以使用plot()
函数来定义五角星的坐标点,并使用fill()
函数为其添加颜色。其他库如Pygame也能实现类似功能,适合创建更复杂的图形和动画效果。
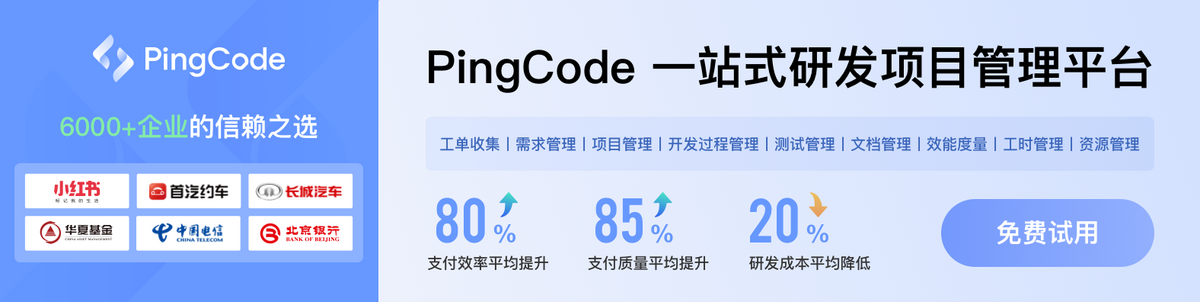