Python将程序输出保存到文件的方法有多种,主要包括使用内置的open()
函数、with
语句、sys.stdout
重定向等。这些方法各有优劣,适用于不同的场景。本文将详细介绍这些方法及其使用场景,帮助你在实际编程中选择最合适的方式。
一、使用 open()
函数
使用open()
函数是最基本也是最常见的方法之一。它允许你以多种模式打开文件(如写模式'w'
、追加模式'a'
等),并将程序输出写入文件中。
1、写模式('w')
在写模式下,文件会被清空,然后新内容会被写入。以下是一个简单的示例:
# 打开文件以写模式
file = open('output.txt', 'w')
写入内容
file.write('Hello, World!\n')
file.write('This is a sample output.\n')
关闭文件
file.close()
2、追加模式('a')
在追加模式下,新内容会被追加到文件的末尾,而不会清空现有内容:
# 打开文件以追加模式
file = open('output.txt', 'a')
写入内容
file.write('Appending a new line.\n')
关闭文件
file.close()
3、二进制模式('b')
当处理非文本文件(如图片、音频文件等)时,可以使用二进制模式:
# 打开文件以二进制写模式
file = open('output.bin', 'wb')
写入二进制数据
file.write(b'\x00\x01\x02\x03')
关闭文件
file.close()
二、使用 with
语句
with
语句是一种更为简洁和安全的方式来处理文件。它能够确保文件在操作完成后自动关闭,即使发生异常也不例外。
1、写模式('w')
以下是使用with
语句的示例:
with open('output.txt', 'w') as file:
file.write('Hello, World!\n')
file.write('This is a sample output.\n')
2、追加模式('a')
同样,使用追加模式也很简单:
with open('output.txt', 'a') as file:
file.write('Appending a new line.\n')
3、二进制模式('b')
处理二进制文件时:
with open('output.bin', 'wb') as file:
file.write(b'\x00\x01\x02\x03')
三、使用 sys.stdout
重定向
有时你可能希望将整个程序的标准输出重定向到文件中,这时候可以使用sys.stdout
重定向。
1、标准输出重定向
以下是一个示例:
import sys
保存原始的标准输出
original_stdout = sys.stdout
打开文件以写模式
with open('output.txt', 'w') as file:
# 重定向标准输出到文件
sys.stdout = file
print('Hello, World!')
print('This is a redirected output.')
恢复原始的标准输出
sys.stdout = original_stdout
2、临时重定向
如果你只需要在某个特定函数或者代码块中重定向标准输出,也可以使用上下文管理器:
from contextlib import redirect_stdout
with open('output.txt', 'w') as file:
with redirect_stdout(file):
print('Hello, World!')
print('This is a redirected output.')
四、使用 logging
模块
对于更复杂的程序,尤其是需要记录日志时,使用logging
模块会更加方便和灵活。
1、基本配置
以下是一个简单的配置示例:
import logging
配置日志记录
logging.basicConfig(filename='output.log', level=logging.INFO)
记录信息
logging.info('This is an info message.')
logging.error('This is an error message.')
2、自定义配置
你可以根据需要自定义日志格式、级别等:
import logging
创建日志记录器
logger = logging.getLogger('my_logger')
logger.setLevel(logging.DEBUG)
创建文件处理器
file_handler = logging.FileHandler('output.log')
file_handler.setLevel(logging.DEBUG)
创建日志格式
formatter = logging.Formatter('%(asctime)s - %(name)s - %(levelname)s - %(message)s')
file_handler.setFormatter(formatter)
添加处理器到记录器
logger.addHandler(file_handler)
记录日志
logger.debug('This is a debug message.')
logger.info('This is an info message.')
logger.warning('This is a warning message.')
logger.error('This is an error message.')
logger.critical('This is a critical message.')
五、使用第三方库
除了内置功能外,Python的生态系统中还有许多第三方库可以帮助你更方便地将程序输出保存到文件中。
1、pandas
如果你处理的是数据表格,pandas
库提供了非常便捷的文件保存功能:
import pandas as pd
创建数据框
data = {
'Name': ['Alice', 'Bob', 'Charlie'],
'Age': [25, 30, 35]
}
df = pd.DataFrame(data)
保存到CSV文件
df.to_csv('output.csv', index=False)
2、json
对于JSON格式的数据,Python内置的json
库同样提供了简便的方法:
import json
创建字典
data = {
'name': 'Alice',
'age': 25,
'city': 'New York'
}
保存到JSON文件
with open('output.json', 'w') as file:
json.dump(data, file)
3、numpy
如果你处理的是数值数组,numpy
库也提供了文件保存功能:
import numpy as np
创建数组
arr = np.array([1, 2, 3, 4, 5])
保存到文本文件
np.savetxt('output.txt', arr)
六、综合示例
最后,我们通过一个综合示例,结合上述多种方法,将不同类型的程序输出保存到不同的文件中:
import sys
import logging
import pandas as pd
import json
import numpy as np
配置日志记录
logging.basicConfig(filename='output.log', level=logging.INFO)
打开文件以写模式
with open('output.txt', 'w') as file:
# 重定向标准输出到文件
with redirect_stdout(file):
print('Hello, World!')
print('This is a redirected output.')
创建数据框并保存到CSV文件
data = {
'Name': ['Alice', 'Bob', 'Charlie'],
'Age': [25, 30, 35]
}
df = pd.DataFrame(data)
df.to_csv('output.csv', index=False)
创建字典并保存到JSON文件
data = {
'name': 'Alice',
'age': 25,
'city': 'New York'
}
with open('output.json', 'w') as file:
json.dump(data, file)
创建数组并保存到文本文件
arr = np.array([1, 2, 3, 4, 5])
np.savetxt('output.txt', arr)
记录日志
logging.info('This is an info message.')
logging.error('This is an error message.')
通过以上示例,你可以看到,Python提供了丰富多样的方法来将程序输出保存到文件中。根据具体需求选择最合适的方法,可以使你的代码更加简洁、易读和高效。
相关问答FAQs:
如何在Python中将输出直接写入文件?
在Python中,可以使用内置的open()
函数来创建或打开一个文件,并使用write()
方法将程序输出保存到该文件中。示例代码如下:
with open('output.txt', 'w') as file:
file.write('这是要保存到文件中的内容。\n')
使用with
语句可以确保文件在写入后自动关闭,避免资源泄露。
如何将多个输出结果保存到同一个文件中?
如果需要将多个输出结果写入同一文件,可以在打开文件时使用追加模式('a'
)而不是写入模式('w'
)。这样,每次写入时不会覆盖原有内容。示例:
with open('output.txt', 'a') as file:
file.write('这是新的一行内容。\n')
如何将Python程序的标准输出重定向到文件?
可以通过重定向sys.stdout
来将所有标准输出保存到文件中。以下是实现的示例:
import sys
with open('output.txt', 'w') as file:
sys.stdout = file
print('这条信息会被写入文件。')
# 其他print语句也会写入文件
sys.stdout = sys.__stdout__ # 恢复标准输出
这样,所有的print
语句将会把内容写入指定的文件,直到恢复标准输出。
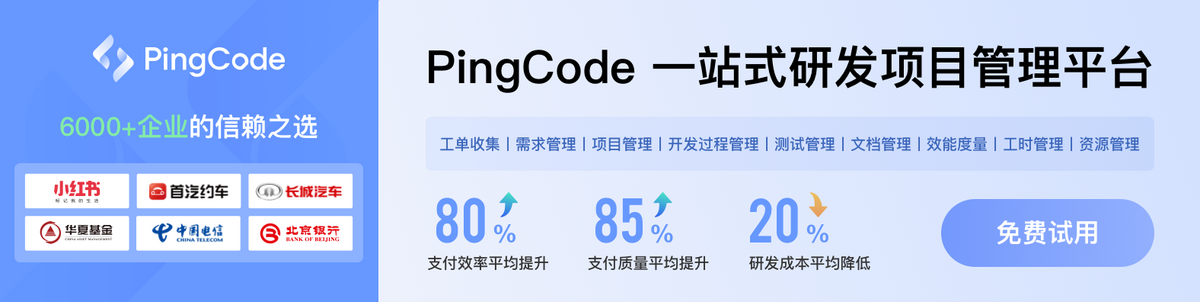