在Python中绘制多个多彩五角星,可以通过以下几个步骤实现:使用turtle库、定义绘制五角星的函数、使用循环创建多个五角星、为每个五角星设置不同颜色。
使用turtle库是绘制图形的一种简单且直观的方式。通过定义一个绘制五角星的函数,我们可以方便地绘制单个五角星,并在循环中调用这个函数来绘制多个五角星。通过设置不同的颜色,我们可以使每个五角星都具有独特的色彩。
一、安装和导入所需的库
首先,确保你已经安装了turtle库。通常,Python自带turtle库,如果没有安装,可以通过pip进行安装:
pip install PythonTurtle
在Python脚本中导入turtle库:
import turtle
import random
二、定义绘制五角星的函数
我们需要一个函数来绘制单个五角星。这可以通过五次绘制直线和转动角度来实现。
def draw_star(turtle_obj, size, color):
turtle_obj.color(color)
turtle_obj.begin_fill()
for _ in range(5):
turtle_obj.forward(size)
turtle_obj.right(144)
turtle_obj.end_fill()
在这个函数中,我们使用turtle的begin_fill()和end_fill()方法来填充颜色。每次绘制一条边后,向右转144度,这样五次循环就能绘制一个五角星。
三、设置绘制多个五角星的循环
为了绘制多个五角星,我们可以使用一个循环。在每次循环中,我们随机设置五角星的位置和颜色。
def draw_multiple_stars(number_of_stars):
screen = turtle.Screen()
screen.bgcolor("white")
star_turtle = turtle.Turtle()
star_turtle.speed(0)
colors = ["red", "blue", "green", "yellow", "purple", "orange"]
for _ in range(number_of_stars):
x = random.randint(-300, 300)
y = random.randint(-300, 300)
size = random.randint(20, 100)
color = random.choice(colors)
star_turtle.penup()
star_turtle.goto(x, y)
star_turtle.pendown()
draw_star(star_turtle, size, color)
screen.mainloop()
在这个函数中,我们创建了一个turtle对象,并设置了随机的位置、大小和颜色。通过调用draw_star函数,我们可以绘制多个五角星。
四、运行程序
最后,我们只需要调用draw_multiple_stars函数,并传入我们想要绘制的五角星数量。
if __name__ == "__main__":
draw_multiple_stars(20)
完整代码
import turtle
import random
def draw_star(turtle_obj, size, color):
turtle_obj.color(color)
turtle_obj.begin_fill()
for _ in range(5):
turtle_obj.forward(size)
turtle_obj.right(144)
turtle_obj.end_fill()
def draw_multiple_stars(number_of_stars):
screen = turtle.Screen()
screen.bgcolor("white")
star_turtle = turtle.Turtle()
star_turtle.speed(0)
colors = ["red", "blue", "green", "yellow", "purple", "orange"]
for _ in range(number_of_stars):
x = random.randint(-300, 300)
y = random.randint(-300, 300)
size = random.randint(20, 100)
color = random.choice(colors)
star_turtle.penup()
star_turtle.goto(x, y)
star_turtle.pendown()
draw_star(star_turtle, size, color)
screen.mainloop()
if __name__ == "__main__":
draw_multiple_stars(20)
这个完整代码将绘制20个随机位置、随机颜色和随机大小的五角星。通过这些步骤和代码示例,你可以轻松地在Python中绘制多个多彩五角星,并且可以根据需要进行调整和扩展。
相关问答FAQs:
如何选择适合的Python库来绘制五角星?
在Python中,有多个库可以用于绘图,其中最常用的是Matplotlib和Turtle。Matplotlib适合于创建静态图形,功能强大,适用于复杂数据可视化;而Turtle则更适合于教育和简单的图形绘制,特别是绘制几何图形如五角星。选择合适的库取决于你的需求和项目的复杂程度。
我可以如何自定义五角星的颜色和大小?
绘制五角星时,可以通过设置颜色参数和坐标来改变其颜色和大小。在使用Matplotlib时,可以使用fill
函数来填充颜色,并通过调整坐标值来实现大小的变化;在Turtle中,可以使用color
函数设置颜色,并通过begin_fill
和end_fill
来填充颜色,同时使用shapesize
来调整大小。
绘制多个五角星时,如何确保它们排列整齐?
为了确保多个五角星的排列整齐,可以通过设置统一的坐标系来控制它们的位置。在Matplotlib中,可以使用循环结构来绘制多个五角星,并通过改变坐标值来控制它们的间距;而在Turtle中,可以使用penup
和pendown
方法在绘制前移动到新的位置,从而确保五角星之间的均匀间距。
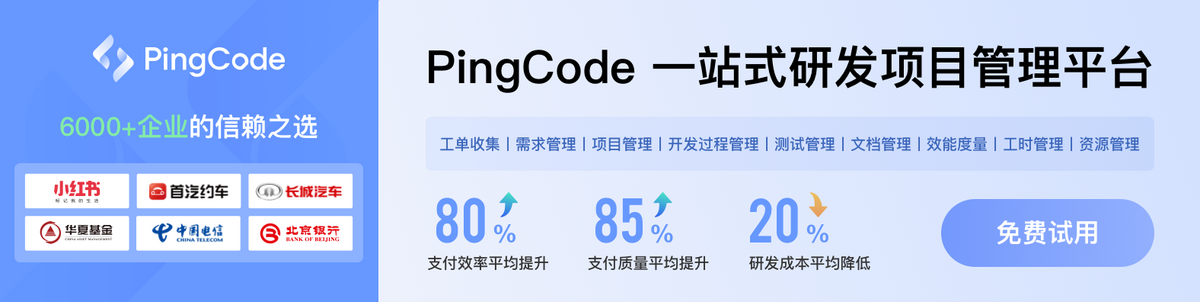