使用Python判断一个字符串的方法包括使用比较运算符、字符串方法和正则表达式。 下面将详细讲解其中一种方法,即使用字符串方法中的 in
操作符进行判断。
in 操作符:这是最简单且直观的方法之一。通过 in
操作符,可以直接判断一个字符串是否包含在另一个字符串中。如果想判断一个字符串是否严格等于另一个字符串,可以使用 ==
操作符。
# 示例代码
string1 = "Hello, world!"
string2 = "Hello"
if string2 in string1:
print(f'"{string2}" is in "{string1}"')
else:
print(f'"{string2}" is not in "{string1}"')
在接下来的内容中,将详细介绍几种常用的判断字符串的方法,包括比较运算符、字符串方法、正则表达式等。
一、比较运算符
1.1 使用 ==
和 !=
在Python中,比较运算符 ==
和 !=
用于判断两个字符串是否相等或不相等。这是最直接的判断方式。
str1 = "hello"
str2 = "hello"
str3 = "world"
判断两个字符串是否相等
if str1 == str2:
print("str1 is equal to str2")
判断两个字符串是否不相等
if str1 != str3:
print("str1 is not equal to str3")
1.2 使用 in
和 not in
in
和 not in
运算符用于判断一个字符串是否包含在另一个字符串中。
sentence = "The quick brown fox jumps over the lazy dog"
word = "quick"
判断字符串是否包含
if word in sentence:
print(f'"{word}" is in the sentence.')
判断字符串是否不包含
if word not in sentence:
print(f'"{word}" is not in the sentence.')
二、字符串方法
2.1 使用 .startswith()
和 .endswith()
.startswith()
和 .endswith()
方法用于判断字符串是否以特定的子字符串开始或结束。
filename = "example.txt"
判断字符串是否以特定子字符串开始
if filename.startswith("exam"):
print("Filename starts with 'exam'.")
判断字符串是否以特定子字符串结束
if filename.endswith(".txt"):
print("Filename ends with '.txt'.")
2.2 使用 .find()
和 .index()
.find()
方法返回子字符串在字符串中的第一个索引位置,如果找不到则返回 -1。.index()
方法也返回子字符串的第一个索引位置,但如果找不到则会引发 ValueError 异常。
text = "Python is fun"
substring = "fun"
使用 .find() 方法
position = text.find(substring)
if position != -1:
print(f"Found '{substring}' in the text at position {position}.")
else:
print(f"'{substring}' not found in the text.")
使用 .index() 方法
try:
position = text.index(substring)
print(f"Found '{substring}' in the text at position {position}.")
except ValueError:
print(f"'{substring}' not found in the text.")
三、正则表达式
正则表达式是一种强大的字符串匹配工具,适用于复杂的字符串匹配和提取。
3.1 使用 re.search()
re.search()
方法用于搜索字符串中是否存在匹配的正则表达式模式。
import re
pattern = r"\bfun\b"
text = "Python is fun"
使用 re.search() 方法
if re.search(pattern, text):
print(f"Pattern '{pattern}' found in the text.")
else:
print(f"Pattern '{pattern}' not found in the text.")
3.2 使用 re.match()
re.match()
方法用于从字符串的开始位置匹配正则表达式模式。
import re
pattern = r"Python"
text = "Python is fun"
使用 re.match() 方法
if re.match(pattern, text):
print(f"Pattern '{pattern}' matches the start of the text.")
else:
print(f"Pattern '{pattern}' does not match the start of the text.")
3.3 使用 re.fullmatch()
re.fullmatch()
方法用于整个字符串匹配正则表达式模式。
import re
pattern = r"Python is fun"
text = "Python is fun"
使用 re.fullmatch() 方法
if re.fullmatch(pattern, text):
print(f"Pattern '{pattern}' fully matches the text.")
else:
print(f"Pattern '{pattern}' does not fully match the text.")
四、案例分析
为了更好地理解上述方法,下面通过几个案例来展示如何使用这些方法进行字符串判断。
4.1 案例一:用户输入验证
假设我们需要验证用户输入的邮箱地址是否符合标准格式,可以使用正则表达式来实现。
import re
def is_valid_email(email):
pattern = r"^[a-zA-Z0-9_.+-]+@[a-zA-Z0-9-]+\.[a-zA-Z0-9-.]+$"
return re.match(pattern, email) is not None
email = "test@example.com"
if is_valid_email(email):
print("Valid email address.")
else:
print("Invalid email address.")
4.2 案例二:日志文件分析
假设我们需要从日志文件中提取包含特定关键词的行,可以使用字符串方法或正则表达式来实现。
import re
def extract_lines_with_keyword(log_file_path, keyword):
with open(log_file_path, 'r') as file:
lines = file.readlines()
# 使用 in 操作符
matching_lines_in = [line for line in lines if keyword in line]
# 使用正则表达式
pattern = re.compile(re.escape(keyword))
matching_lines_re = [line for line in lines if pattern.search(line)]
return matching_lines_in, matching_lines_re
log_file_path = "system.log"
keyword = "ERROR"
lines_in, lines_re = extract_lines_with_keyword(log_file_path, keyword)
print("Lines found using 'in' operator:")
print("\n".join(lines_in))
print("Lines found using regex:")
print("\n".join(lines_re))
通过上述几个案例,相信大家对如何使用Python判断字符串有了更深入的了解。无论是简单的字符串比较,还是复杂的正则表达式匹配,Python都提供了丰富的工具和方法来满足不同的需求。在实际应用中,可以根据具体场景选择合适的方法来处理字符串判断问题。
相关问答FAQs:
如何在Python中检查一个字符串是否等于另一个字符串?
在Python中,可以使用“==”运算符来判断一个字符串是否等于另一个字符串。例如,str1 == str2
将返回True,如果str1和str2的内容完全相同。如果需要比较时忽略大小写,可以使用str1.lower() == str2.lower()
来实现。
Python提供了哪些方法来查找字符串的特定值?
Python的字符串对象提供了多种方法来查找特定值。例如,使用in
运算符可以检查一个字符串是否包含另一个字符串:if substring in main_string:
。此外,使用str.find(substring)
和str.index(substring)
方法可以找到子字符串的位置,返回-1表示未找到,而index方法在未找到时会引发异常。
如何在Python中比较字符串的相似性?
比较字符串的相似性可以使用多种方法。最常见的是使用Levenshtein距离,衡量将一个字符串转换为另一个字符串所需的最小编辑操作数。Python中有第三方库如python-Levenshtein
可以很方便地实现此功能。此外,可以使用difflib
模块中的SequenceMatcher
来计算两个字符串的相似度得分,这对于文本比较非常有效。
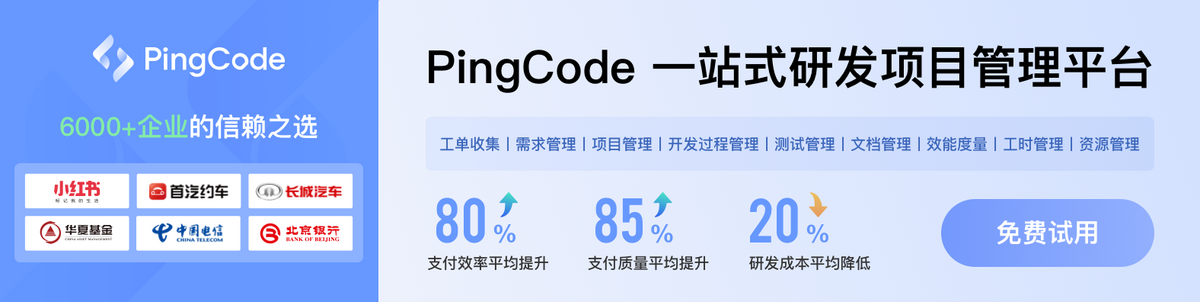